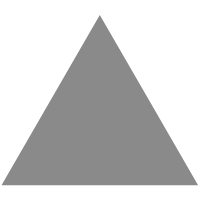
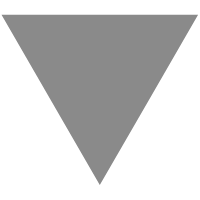
这八个NumPy函数可以解决90%的常见问题
source link: https://www.51cto.com/article/756469.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
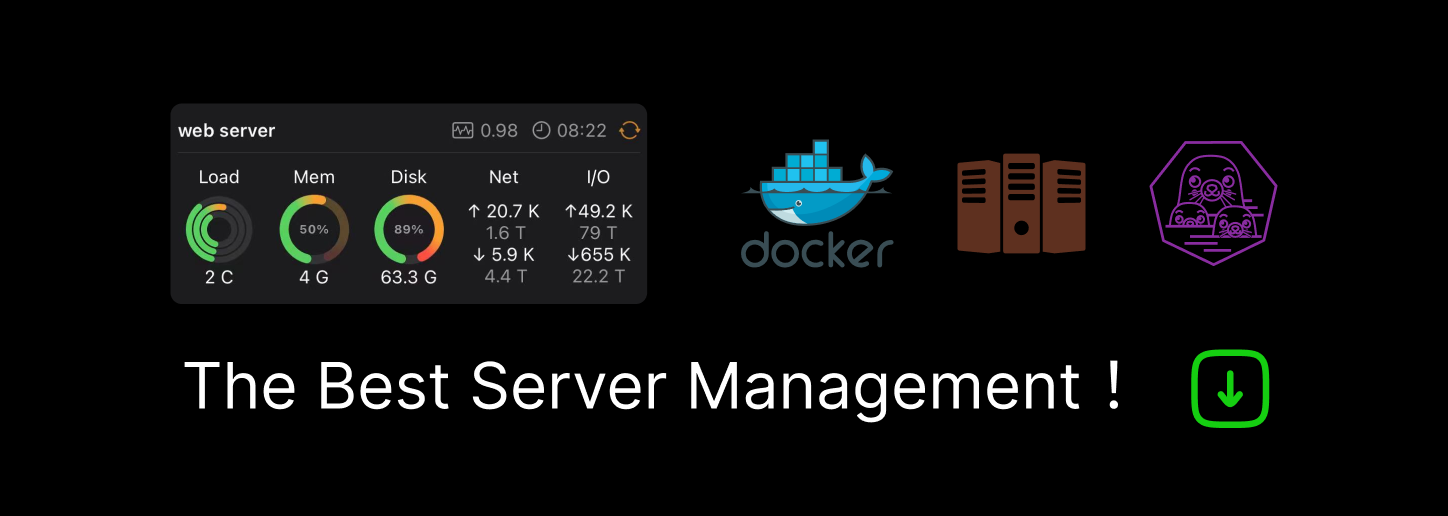
这八个NumPy函数可以解决90%的常见问题
NumPy是一个用于科学计算和数据分析的Python库,也是机器学习的支柱。可以说NumPy奠定了Python在机器学习中的地位。NumPy提供了一个强大的多维数组对象,以及广泛的数学函数,可以对大型数据集进行有效的操作。这里的“大”是指数百万行。
NumPy是一个用于科学计算和数据分析的Python库,也是机器学习的支柱。可以说NumPy奠定了Python在机器学习中的地位。NumPy提供了一个强大的多维数组对象,以及广泛的数学函数,可以对大型数据集进行有效的操作。这里的“大”是指数百万行。
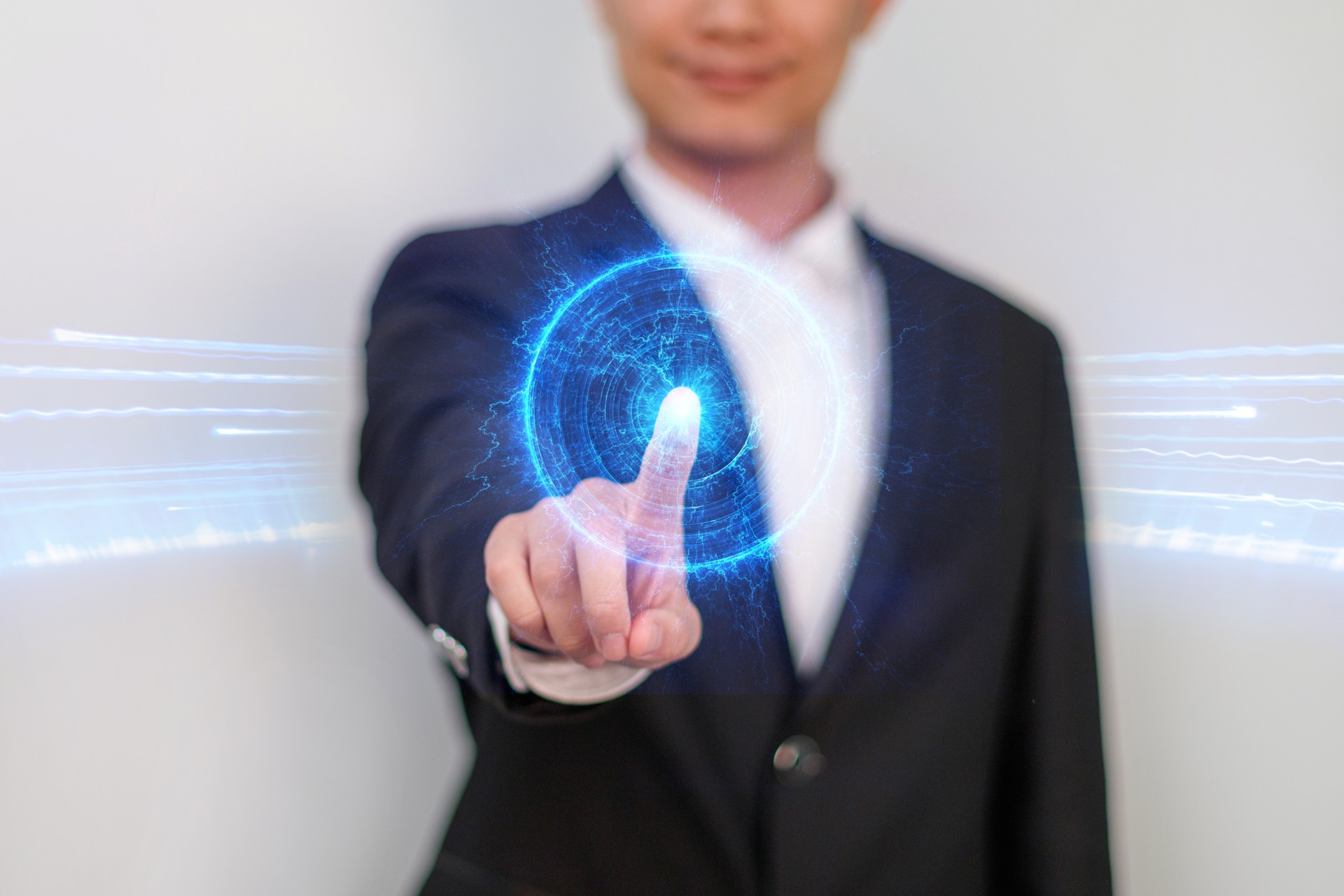
Numpy快速而高效的原因是底层的C代码,这比使用Python进行数组的操作要快上几百倍,并且随着数据量级的上升而上升。
本文中整理了一些可以解决常见问题的主要的NumPy函数。
1、创建数组
numpy.array:创建新的NumPy数组
# Create an array using np.array()
arr = np.array([1, 2, 3, 4, 5])
print(arr)
Ouput: [1 2 3 4 5]
numpy.zeros:创建一个以零填充的数组。
# Create a 2-dimensional array of zeros
arr = np.zeros((3, 4))
[[0. 0. 0. 0.]
[0. 0. 0. 0.]
[0. 0. 0. 0.]]
类似的还有numpy.ones:创建一个都是1的数组 / numpy.empty:在不初始化数组元素的情况下创建数组。
使用numpy.random:生成随机数组的函数。
# Generate a random integer between 0 and 9
rand_int = np.random.randint(10)
print(rand_int)
numpy.linspace:在指定范围内生成均匀间隔的数字。
# Generate an array of 5 values from 0 to 10 (inclusive)
arr = np.linspace(0, 10, 5)
# Print the array
print(arr)
[ 0. 2.5 5. 7.5 10. ]
numpy.range:用间隔的值创建数组。
# Generate an array from 0 to 10 (exclusive) with step size 1
arr = np.arange(0, 10, 2)
# Print the array
print(arr)
[1 3 5 7 9]
2、查看数组信息
numpy.shape:返回一个表示数组形状的元组。
numpy.ndim:返回数组的维度数。
numpy.dtype:获取数组中元素的数据类型。可以是int型,float型,bool型等等。
3、数组操作函数
numpy.reshape:改变数组的形状。
# Create a 1-dimensional array
arr = np.array([1, 2, 3, 4, 5, 6])
# Reshape the array to a 2x3 matrix
reshaped_arr = np.reshape(arr, (2, 3))
[[1 2 3]
[4 5 6]]
numpy.transpose:用于排列数组的维度。它返回一个轴调换后的新数组。
# Create a 2-dimensional array
arr = np.array([[1, 2, 3],
[4, 5, 6]])
# Transpose the array
transposed_arr = np.transpose(arr)
[[1 4]
[2 5]
[3 6]]
numpy.concatate:沿现有轴连接数组。
# Create two 1-dimensional arrays
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# Concatenate the arrays along axis 0 (default)
concatenated_arr = np.concatenate((arr1, arr2))
[1 2 3 4 5 6]
numpy.split:分割数据,numpy.resize:改变数组的形状和大小。
numpy.vstack:将多个数组垂直堆叠以创建一个新数组。
# Create two 1-dimensional arrays
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# Vertically stack the arrays
stacked_arr = np.vstack((arr1, arr2))
[[1 2 3]
[4 5 6]]
numpy.hstack:与vstack类似,但是是水平堆叠数组。
4、数学函数
numpy.sum:计算数组元素的和。
numpy.mean:计算数组的算术平均值。
numpy.max:返回数组中的最大值。
numpy.min:返回数组中的最小值。
numpy.abs:计算元素的绝对值。
numpy.exp:计算所有元素的指数。
numpy.subtract:对两个数组的对应元素进行减法运算。
numpy.multiply:对两个数组的对应元素进行乘法运算。
numpy.divide:对两个数组的对应元素进行除法运算。
numpy.sin:计算数组中每个元素的正弦值。
numpy.cos:计算数组中每个元素的余弦值。
numpy.log:计算数组中每个元素的自然对数(以e为底的对数)。
5、统计函数
numpy.std:计算数组的标准差。
# Create a 1-dimensional array
arr = np.array([1, 2, 3, 4, 5])
# Compute the standard deviation of the array
std = np.std(arr)
1.4142135623730951
numpy.var:计算数组的方差。
numpy.histogram:计算一组数据的直方图。
numpy.percentile:计算数组的第n个百分位数。它返回低于给定百分比的数据的值。
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Calculate the 50th percentile (median) of the data
median = np.percentile(data, 50)
# Calculate the 25th and 75th percentiles (quartiles) of the data
q1 = np.percentile(data, 25)
q3 = np.percentile(data, 75)
Median: 5.5
Q1: 3.25
Q3: 7.75
numpy.corcoef:计算两个数组之间的相关系数。numpy.mean: 计算数组元素的平均值。numpy.median:计算数组元素的中位数。
numpy.random.rand:在区间[0,1]内从均匀分布生成随机数数组
# Generate a 1-dimensional array of random numbers
random_array = np.random.rand(5)
[0.35463311 0.67659889 0.5865293 0.77127035 0.13949178]
numpy.random.normal:从正态(高斯)分布生成随机数
# Generate a random number from a normal distribution
random_number = np.random.normal()
-0.6532785285205665
6、线性代数函数
numpy.dot:计算两个数组的点积。
# Create two arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# Compute the dot product of the arrays
dot_product = np.dot(a, b)
32
numpy.linalg.inv:计算一个方阵的逆, numpy.linalg.eig:一个方阵的特征值和特征向量。numpy.linalg.solve:求解一个线性方程组。
7、排序函数
numpy.sort:沿指定轴返回数组的排序副本
# Create a 2D array
arr = np.array([[3, 1, 5], [2, 4, 6]])
# Sort the array along the second axis (columns)
sorted_arr = np.sort(arr, axis=1)
[[1 3 5]
[2 4 6]]
numpy.argsort:返回按升序对数组排序的索引
# Create an array
arr = np.array([3, 1, 5, 2, 4])
# Get the indices that would sort the array
sorted_indices = np.argsort(arr)
[1 3 0 4 2]
8、其他一些高级的函数
numpy.unique:在数组中查找唯一的元素。
arr = np.array([2, 1, 3, 2, 1, 4, 5, 4])
# Get the unique elements of the array
unique_values = np.unique(arr)
[1 2 3 4 5]
numpy.fft:傅里叶变换的函数。
numpy.ma:供对掩码数组的支持。
- numpy.ma.array:从现有的数组或序列创建一个掩码数组。
- numpy.ma.masked_array:从现有数组和掩码中创建一个掩码数组。
- numpy.ma.mask:表示掩码数组中的掩码值。
- numpy.ma.masked_invalid:屏蔽数组中无效的(NaN, Inf)元素。
- numpy.ma.masked_greate, numpy.ma.masked_less:掩码大于或小于给定值的元素。
arr = np.array([1, 2, 3, np.nan, 5])
# Create a masked array by masking the invalid values
masked_arr = ma.masked_invalid(arr)
[1 2 3 5]
numpy.apply_along_axis:沿着数组的特定轴应用函数。
numpy.wheres:一个条件函数,根据给定条件返回数组中满足条件的元素的索引或值。
condition = np.array([True, False, True, False])
# Create two arrays
array_true = np.array([1, 2, 3, 4])
array_false = np.array([5, 6, 7, 8])
result = np.where(condition, array_true, array_false)
[1 6 3 8]
以上就是Numpy最经常被使用的函数,希望对你有所帮助。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK