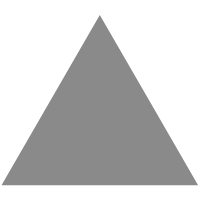
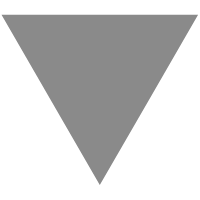
使用phpunit进行单元测试
source link: https://blog.p2hp.com/archives/11136
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
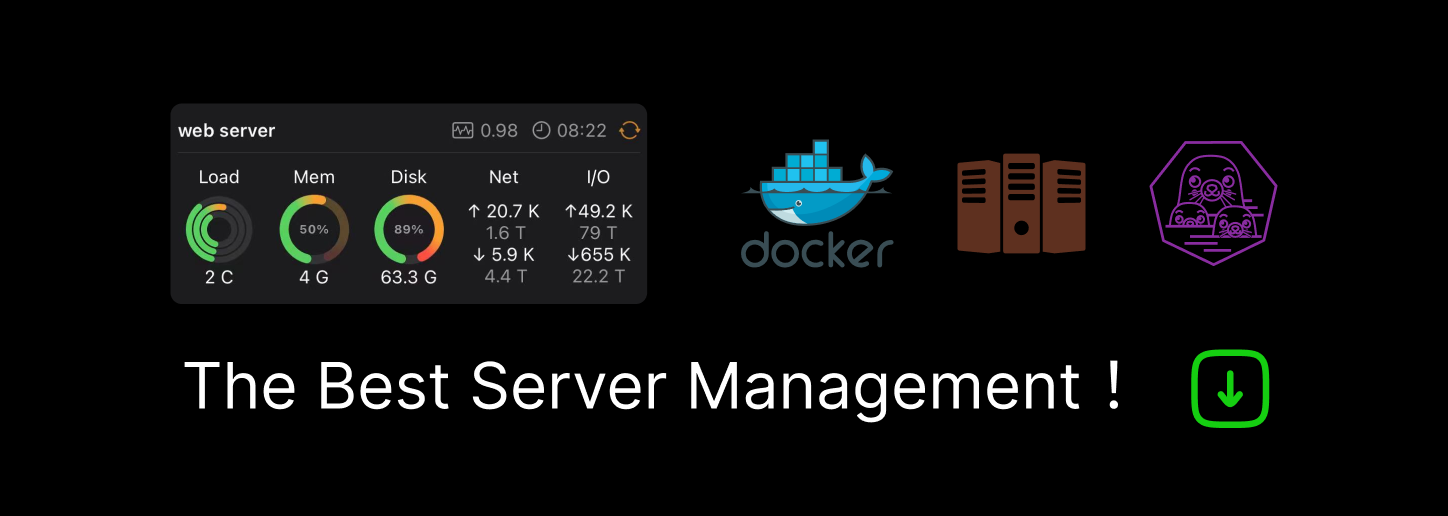
本教程假定您使用 PHP 8.1 或 PHP 8.2。您将学习如何编写简单的单元测试以及如何下载和运行 PHPUnit.
PHPUnit 10 的文档可以在这里找到。
下载:可以用以下2种方法之一:
1.PHP 存档 (PHAR)
我们分发了一个PHP存档(PHAR),其中包含使用PHPUnit 10所需的一切。只需从这里下载并使其可执行:
wget -O phpunit https://phar.phpunit.de/phpunit-10.phar ➜ chmod +x phpunit ➜ ./phpunit --version PHPUnit 10.0.0 by Sebastian Bergmann and contributors.
2.Composer
您可以使用 Composer 将 PHPUnit 作为本地、每个项目、开发时依赖项添加到您的项目中:
➜ composer require --dev phpunit/phpunit ^10 ➜ ./vendor/bin/phpunit --version PHPUnit 10.0.0 by Sebastian Bergmann and contributors.
上面显示的示例假定composer
在您的$PATH
上。
您的 composer.json
应该看起来像这样:
{ "autoload": { "classmap": [ "src/" ] }, "require-dev": { "phpunit/phpunit": "^10" } }
src/Email.php
<?php declare(strict_types=1); final class Email { private string $email; private function __construct(string $email) { $this->ensureIsValidEmail($email); $this->email = $email; } public static function fromString(string $email): self { return new self($email); } public function asString(): string { return $this->email; } private function ensureIsValidEmail(string $email): void { if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { throw new InvalidArgumentException( sprintf( '"%s" is not a valid email address', $email ) ); } } }
tests/EmailTest.php
<?php declare(strict_types=1); use PHPUnit\Framework\TestCase; final class EmailTest extends TestCase { public function testCanBeCreatedFromValidEmail(): void { $string = '[email protected]'; $email = Email::fromString($string); $this->assertSame($string, $email->asString()); } public function testCannotBeCreatedFromInvalidEmail(): void { $this->expectException(InvalidArgumentException::class); Email::fromString('invalid'); } }
测试执行:以下2种方法都可以:
1.PHP 存档 (PHAR)
➜ ./phpunit --bootstrap src/autoload.php tests PHPUnit 10.0.0 by Sebastian Bergmann and contributors. .. 2 / 2 (100%) Time: 70 ms, Memory: 10.00MB OK (2 tests, 2 assertions)
上面假设你已经下载了phpunit.phar
并将其作为phpunit
放入你的$PATH
,并且src/autoload.php
是一个为要测试的类设置自动加载 的脚本。这样的脚本通常使用 phpab 等工具生成。
--bootstrap src/autoload.php
指示 PHPUnit 命令行测试运行程序在运行测试之前包含src/autoload.php
.
tests
指示 PHPUnit 命令行测试运行程序执行在 tests
目录的 *Test.php
源代码文件中声明的所有测试.
2.Composer
➜ ./vendor/bin/phpunit tests PHPUnit 10.0.0 by Sebastian Bergmann and contributors. .. 2 / 2 (100%) Time: 70 ms, Memory: 10.00MB OK (2 tests, 2 assertions)
上面假设 vendor/autoload.php
(由 Composer 管理的自动加载器脚本)存在,并且能够加载 Email
类的代码。根据设置自动加载的方式,您可能需要立即运行composer dump-autoload
。
tests
指示 PHPUnit 命令行测试运行程序执行在 tests
目录的 *Test.php
源代码文件中声明的所有测试.
一些测试组件推荐:
https://packagist.org/packages/mockery/mockery
phpunit/phpunit
fakerphp/faker
https://github.com/phpstan/phpstan
vimeo/psalm
mikey179/vfsstream
rector/rector
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK