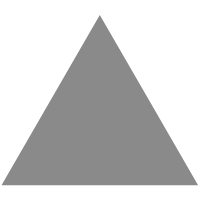
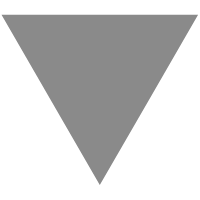
Replace every node in a linked list with its closest bell number
source link: https://www.geeksforgeeks.org/replace-every-node-in-a-linked-list-with-its-closest-bell-number/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
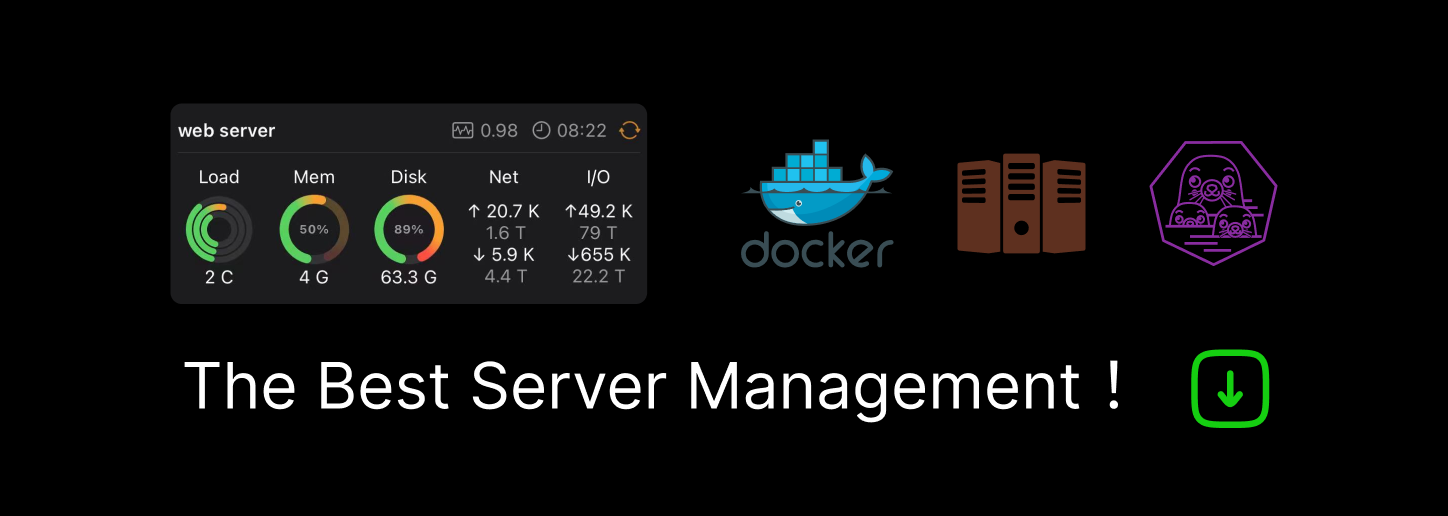
Replace every node in a linked list with its closest bell number
Given a singly linked list, the task is to replace every node with its closest bell number.
Bell numbers are a sequence of numbers that represent the number of partitions of a set. In other words, given a set of n elements, the Bell number for n represents the total number of distinct ways that the set can be partitioned into subsets.
The first few Bell numbers are 1, 1, 2, 5, 15, 52, 203, 877, 4140, 21147, …
Examples:
Input: 14 -> 7 -> 190 -> 2 -> 5 -> NULL
Output: 15 -> 5 -> 203 -> 2 -> 5 -> NULL
Explanation: The closest Bell numbers for each node are:
- Node 1 (value = 14): Closest Bell number = 15.
- Node 2 (value = 7): Closest Bell number = 5.
- Node 3 (value = 190): Closest Bell number = 203.
- Node 4 (value = 2): Closest Bell number = 2.
- Node 5 (value = 5): Closest Bell number = 5.
Input: 50 -> 1 -> 4 -> NULL
Output: 52 -> 1 -> 5 -> NULL
Explanation: The closest Bell numbers for each node are:
- Node 1 (value = 50): Closest Bell number = 52.
- Node 1 (value = 1): Closest Bell number = 1.
- Node 1 (value = 4): Closest Bell number = 5.
Approach: This can be solved with the following idea:
The algorithm first calculates the Bell number at a given index by filling a 2D array using a dynamic programming approach. Then, it finds the closest Bell number to a given node value by iterating through the Bell numbers until it finds a Bell number greater than or equal to the node value. It then compares the difference between the previous and current Bell numbers to determine which one is closer to the node value. Finally, it replaces each node in the linked list with its closest Bell number using a while loop that iterates through each node in the linked list.
Steps of the above approach:
- Define a bellNumber function that takes an integer n as an argument and returns the nth Bell number. The function uses dynamic programming to calculate the Bell number.
- Define a closestBell function that takes an integer n as an argument and returns the closest Bell number to n.
- This function iteratively calls the bellNumber function until it finds the smallest Bell number greater than or equal to n.
- If n is less than the first Bell number (which is 1), then the function returns the first Bell number. Otherwise, the function compares the difference between n and the previous Bell number to the difference between the next Bell number and n and returns the closer Bell number.
- Define a replaceWithBell function that takes the head of a linked list as an argument and replaces the data value of each node in the list with the closest Bell number to its original data value.
- The function iterates through each node in the list, calls the closestBell function to find the closest Bell number to the node’s original data value, and assigns that value to the node’s data field.
Below is the implementation of the above approach:
// C++ code for the above approach: #include <cmath> #include <iostream> using namespace std; // Node structure of singly linked list struct Node { int data; Node* next; }; // Function to add a new node at the // beginning of the linked list void push(Node** head_ref, int new_data) { Node* new_node = new Node; new_node->data = new_data; new_node->next = (*head_ref); (*head_ref) = new_node; } // Function to print the linked list void printList(Node* node) { while (node != NULL) { cout << node->data; if (node->next != NULL) { cout << " -> "; } node = node->next; } } // Function to find the Bell number at // the given index int bellNumber(int n) { int bell[n + 1][n + 1]; bell[0][0] = 1; for (int i = 1; i <= n; i++) { bell[i][0] = bell[i - 1][i - 1]; for (int j = 1; j <= i; j++) { bell[i][j] = bell[i - 1][j - 1] + bell[i][j - 1]; } } return bell[n][0]; } // Function to find the closest Bell number // to a given node value int closestBell(int n) { int bellNum = 0; while (bellNumber(bellNum) < n) { bellNum++; } if (bellNum == 0) { return bellNumber(bellNum); } else { int prev = bellNumber(bellNum - 1); int curr = bellNumber(bellNum); return (n - prev < curr - n) ? prev : curr; } } // Function to replace every node with // its closest Bell number void replaceWithBell(Node* node) { while (node != NULL) { node->data = closestBell(node->data); node = node->next; } } // Driver code int main() { Node* head = NULL; // Creating the linked list push(&head, 5); push(&head, 2); push(&head, 190); push(&head, 7); push(&head, 14); // Function call replaceWithBell(head); printList(head); return 0; }
15 -> 5 -> 203 -> 2 -> 5
Time Complexity: O(n3)
Auxiliary Space: O(n2)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK