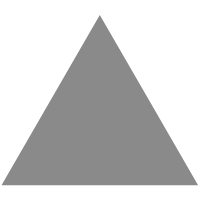
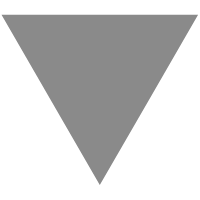
ES2023 features list
source link: https://h3manth.com/ES2023/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
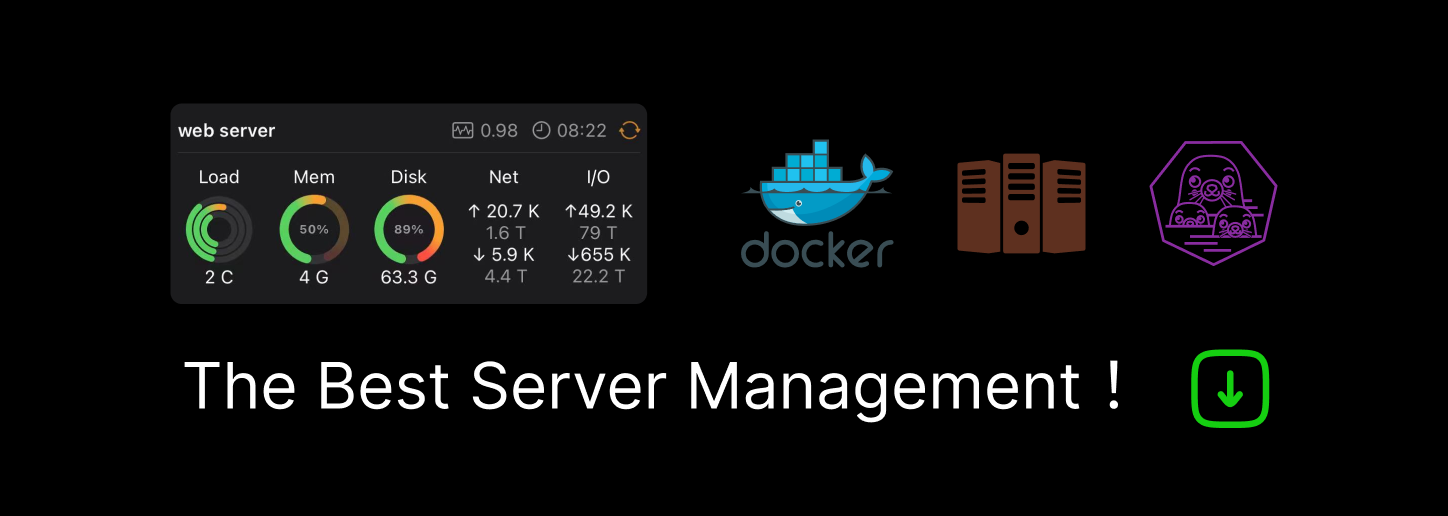
ES2023 Features!
Array find from last
Array.prototype.findLast and Array.prototype.findLastIndex 📕
let nums = [5,4,3,2,1];
let lastEven = nums.findLast((num) => num % 2 === 0); // 2
let lastEvenIndex = nums.findLastIndex((num) => num % 2 === 0); // 3
Hashbang Grammar
#! for JS 📕
The first line of this script begins with #!, which indicates a comment that can contain any text.
#!/usr/bin/env node
// in the Script Goal
'use strict';
console.log(1);
Symbols as WeakMap keys
Use symbols in weak collections and registries 📕
Note: registered symbols aren’t allowed as weakmap keys.
let sym = Symbol("foo");
let obj = {name: "bar"};
let wm = new WeakMap();
wm.set(sym, obj);
console.log(wm.get(sym)); // {name: "bar"}
sym = Symbol("foo");
let ws = new WeakSet();
ws.add(sym);
console.log(ws.has(sym)); // true
sym = Symbol("foo");
let wr = new WeakRef(sym);
console.log(wr.deref()); // Symbol(foo)
sym = Symbol("foo");
let cb = (value) => {
console.log("Finalized:", value);
};
let fr = new FinalizationRegistry(cb);
obj = {name: "bar"};
fr.register(obj, "bar", sym);
fr.unregister(sym);
Change Array by copy
return changed Array and TypedArray copies 📕
Note: typed array doesn’t have tospliced.
const greek = ['gamma', 'aplha', 'beta']
greek.toSorted(); // [ 'aplha', 'beta', 'gamma' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
const nums = [0, -1, 3, 2, 4]
nums.toSorted((n1, n2) => n1 - n2); // [-1,0,2,3,4]
nums; // [0, -1, 3, 2, 4]
const greek = ['gamma', 'aplha', 'beta']
greek.toReversed(); // [ 'beta', 'aplha', 'gamma' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
const greek = ['gamma', 'aplha', 'beta']
greek..toSpliced(1,2); // [ 'gamma' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
greek.toSpliced(1,2, ...['delta']); // [ 'gamma', 'delta' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
const greek = ['gamma', 'aplha', 'beta']
greek..toSpliced(1,2); // [ 'gamma' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
greek.toSpliced(1,2, ...['delta']); // [ 'gamma', 'delta' ]
greek; // [ 'gamma', 'aplha', 'beta' ]
const greek = ['gamma', 'aplha', 'beta'];
greek.with(2, 'bravo'); // [ 'gamma', 'aplha', 'bravo' ]
greek; // ['gamma', 'aplha', 'beta'];
With ❤️ Hemanth.HM
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK