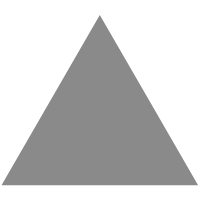
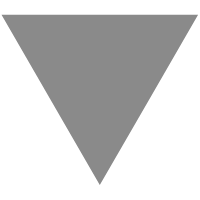
Count pairs with special numbers
source link: https://www.geeksforgeeks.org/count-pairs-with-special-numbers/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
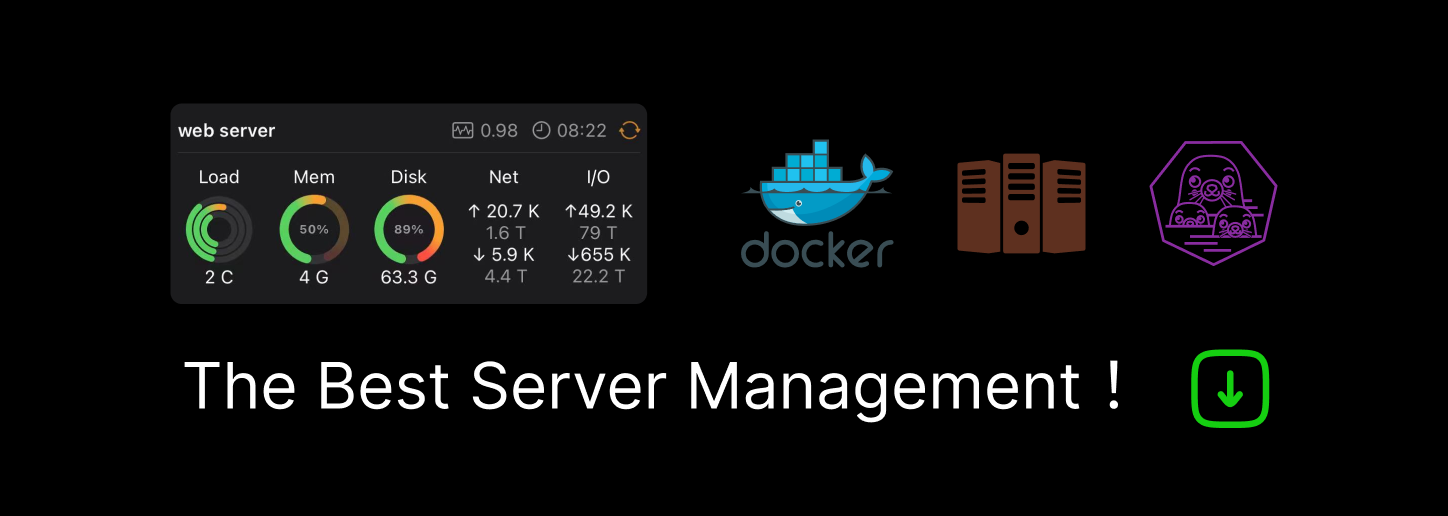
Count pairs with special numbers
Given an array arr[] of size N. You need to find the total number of good pairs such that the if you chose two numbers arr[i] and arr[j] then the following condition holds arr[i] + SpecialNumber(arr[j]) = = arr[j] + SpecialNumber(arr[i]). Here Special Number indicates the number after removing the digits at the even position of the given number like if there is a number 1234 then the SpecialNumber(1234) will be 13 which comes after removing 2 and 4 from even positions.
Examples:
Input:- N = 3, arr[] = [4, 5, 6]
Output:- 3
Explanation: There is no number whose length is two so numbers remain the same after converting them to special numbers and 4 + SpecialNumber(6) == 6 + SpecialNumber(4), similarly with (4, 5) and with (5, 6).Input: N = 4, arr[] = [12, 13, 12, 20]
Output: 1
Explanation: only one pair occurs which is (12, 12).
Approach: This can be solved with the following idea:
- As we have to find such pairs which hold the condition arr[i] + SpecialNumber(arr[j]) == arr[j] + SpecialNumber(arr[i]).
- Let’s make this simple by changing the equation to arr[i] – SpecialNumber(arr[i]) == arr[j] – SpecialNumber(arr[j]).
- Now we just have to find out the numbers whose difference with their special number is equal.
- After finding the count of such a number we can simply apply the formula to find the total pairs which is, let’s say that there are n numbers with the same difference of their value and their special number. Then the total pairs we can form by use of them are (n-1)*(n)/2.
- We will find the total pairs in linear time by using this formula.
Below are the steps involved in the implementation of the code:
- For finding the difference between the number and its special number first of all we need to find the special number for every number.
- After this, we will find the difference between each number with its special number and store it in a unordered_map.
- After this, we will just traverse the map and count the pairs which we can form for a particular value.
Below is the implementation of the code:
// C++ code for the above approac #include <bits/stdc++.h> using namespace std; // Function to find special number // of a number int SpecialNumber(int n) { // Coverting number to string // for easy use string num = to_string(n); int ans = 0; for (int i = 0; i < num.length(); i++) { // Taking only odd position // digits in 1-based indexing if (i % 2 == 0) { ans = ans * 10 + (num[i] - '0'); } } // Returning special number return ans; } // Function to count pairs int CountPairs(int n, vector<int> arr) { // To store total pairs int ans = 0; // Map to store difference of // number and its special number unordered_map<int, int> mm; for (int i = 0; i < n; i++) { // Taking specialnumber int temp = SpecialNumber(arr[i]); mm[arr[i] - temp]++; } // Iterating over map for (auto x : mm) { // Taking frequency of a // particular difference int temp = x.second; // Counting total pairs of a // particular difference ans += (temp * (temp - 1)) / 2; } return ans; } // Driver code int main() { int N = 3; vector<int> arr = { 4, 5, 6 }; // Function call cout << CountPairs(N, arr); return 0; }
3
Time Complexity: O(N*X)
Auxiliary Space: O(N)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK