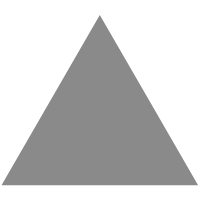
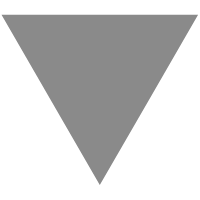
How to Get Elements by Class, ID, Tag Name or Selectors in JavaScript
source link: https://code.tutsplus.com/tutorials/how-to-get-elements-by-class-id-or-tag-name-in-javascript--cms-106818
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
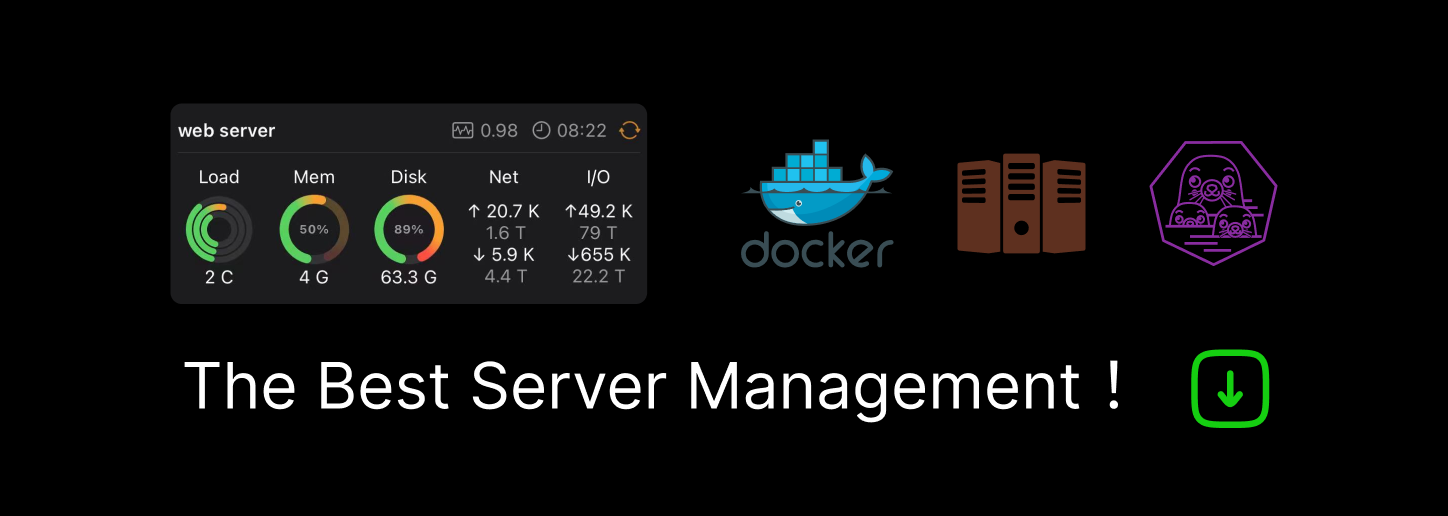
Any kind of DOM manipulation first requires you to select the DOM elements that you want to manipulate. There are quite a few methods that you can use to get access to any element in your DOM. In this tutorial, we will learn how to use these methods.
Access a DOM Element by ID
Elements in your DOM can have an id
attribute that identifies them uniquely. This id
is used for a lot of purposes such as a fragment identifier or for scripting and styling.
Since each element is supposed to have a unique value for the id attribute, you can easily access that element fairly quickly using the getElementbyId()
method. It is important to keep in mind that the id
is case-sensitive. This method will return null
if there is no element with the specified id
.
Another side effect of having a single unique id per document is that this method is only available for the document
object. You cannot call it on any other elements in the DOM. Here is an example of using this method:
<ul> |
|
2 |
<li>Apple</li> |
3 |
<li>Banana</li> |
4 |
<li id="favorite">Mango</li> |
5 |
<li>Lichi</li> |
6 |
<li>Papaya</li> |
7 |
<li>Grapes</li> |
8 |
</ul> |
We can get a reference to the list element with id
set to favorite
by using the following JavaScript:
let favorite_fruit = document.getElementById('favorite'); |
|
2 |
let hated_fruit = document.getElementById('hated'); |
3 |
|
4 |
// Ouptut: <li id="favorite">Mango</li> |
5 |
console.log(favorite_fruit); |
6 |
|
7 |
// Output: null |
8 |
console.log(hated_fruit); |
There was no element with id
value hated so we got null
instead.
Access DOM Elements by Using Tag Names
When we write our HTML or markup we use tags to create different elements. The name of HTML element goes inside the start tags and end tags. In our previous example, we used two different tags. The ul
tag for creating an unordered list and the li
tag for listing our elements.
There can be multiple elements in a single document with the same tag names. We can get access to them by using the getElementsByTagName()
method. This method give back a live HTMLCollection
interface. The term live here means that the collection will update automatically to stay synchronized with the DOM tree and you won't have to make calls to the method whenever the DOM changes.
The method is defined for both the document
object as well as the Element
class which is inherited by all element objects. Here is an example of using this method:
<h1>The <span>Blue-Throated Macaw</span> (Ara Glaucogularis)</h1> |
|
2 |
|
3 |
<p id="intro">The blue-throated macaw (Ara glaucogularis) is a species of <span>macaw</span> |
4 |
that is <span>endemic</span> to a small area of north-central |
5 |
<span>Bolivia</span>, known as the Llanos de Moxos. |
6 |
Recent <span>population</span> and range estimates suggest that about |
7 |
350 to 400 individuals remain in the wild.</p> |
You can see that there are a total of five span
tags in our markup and four of those are within our p
tag which uses the string intro as its id
.
We will use the getElementsByTagName()
method twice to get two separate collections of span
elements. One for the whole document and other for the intro paragraph.
let span_elems = document.getElementsByTagName("span"); |
|
2 |
let intro_p = document.getElementById("intro"); |
3 |
let p_span_elems = intro_p.getElementsByTagName("span"); |
4 |
|
5 |
// Output: HTMLCollection { 0: span, 1: span, 2: span, 3: span, 4: span, length: 5 } |
6 |
console.log(span_elems); |
7 |
|
8 |
// Output: <span>Blue-Throated Macaw</span> |
9 |
console.log(span_elems[0]) |
// Output: HTMLCollection { 0: span, 1: span, 2: span, 3: span, length: 4 } |
|
console.log(p_span_elems); |
As you can see, the object that we get back contains a length
property which you can use to get the total number of elements in the collection. We can also directly access DOM elements in the collection by using a zero-based index.
Access DOM Elements by Using Class Names
We can assign class names to different DOM elements by using the class
attribute. A single element can have multiple space-separated classes. Two or more different DOM elements can also have same class applied to them.
The classes you assign will also be case-sensitive so using bird-name and Bird-name as class names will not group the elements together for either for the purpose of scripting or class selectors.
We can get a list of all the elements with one or more specified class names by using the getElementsbyClassName()
method. This method is also defined for both the document
object as well as the Element
class. When called on an element, this method will only return elements which are descendants of the specified root element.
The elements are returned as a live HTMLCollection
and you can access those elements by using indexes. It is important to keep in mind that the elements in the collection will update whenever the DOM changes. This means that you cannot rely on an element always being present if its classes have been manipulated after the first call to this method.
Here are some basic examples of using this method:
<h1>The <span class="bird-name important">Blue-Throated Macaw</span> (Ara Glaucogularis)</h1> |
|
2 |
|
3 |
<p id="intro">The <b class="bird-name important">blue-throated macaw</b> (Ara <span class="big-word tooltip">glaucogularis</span>) is |
4 |
a species of <span>macaw</span> that is <span>endemic</span> to a small area of |
5 |
north-central <b class="important">Bolivia</b>, known as the Llanos de Moxos. |
6 |
Recent <span class="big-word tooltip">population</span> and range estimates |
7 |
suggest that about 350 to 400 <span class="big-word">individuals</span> remain |
8 |
in the wild.</p> |
In the above markup the name of the bird is mentioned in two different places using two different tags. We cannot use the getElementsByTagName()
method if we want to select both those elements. So, the getElementsByClassName()
method comes to our rescue:
let bird_name_elems = document.getElementsByClassName("bird-name"); |
|
2 |
let important_elems = document.getElementsByClassName("important"); |
3 |
let big_word_tooltips = document.getElementsByClassName("big-word tooltip"); |
4 |
|
5 |
// Output: HTMLCollection { 0: span.bird-name.important, 1: b.bird-name.important, length: 2 } |
6 |
console.log(bird_name_elems); |
7 |
|
8 |
// Output: HTMLCollection { 0: span.bird-name.important, 1: b.bird-name.important, 2: b.important, length: 3 } |
9 |
console.log(important_elems); |
// Output: HTMLCollection { 0: span.big-word.tooltip, 1: span.big-word.tooltip, length: 2 } |
|
console.log(big_word_tooltips); |
In our third example, we supplied two different class names to the getElementsByClassName()
method. This mean that only elements with both these classes were present in our collection.
Access DOM Elements Using Specified Selector(s)
In all the examples so far, we used either the ID, the tag name or the class names to access different elements. We never used a combination of them. It is possible to just use the previous three methods to get to all elements in the DOM. However, things can quickly get complicated.
An alternative here is to use the querySelector()
method which will return the first element that matches the selector you specified in the argument. One important thing to remember is that using this method with pseudo-elements will not return anything.
let id_class_combo = document.getElementById("intro").getElementsByClassName("bird-name"); |
|
2 |
let para_bird = document.querySelector("#intro .bird-name"); |
3 |
|
4 |
// Output: <b class="bird-name important">blue-throated macaw</b> |
5 |
console.log(id_class_combo[0]); |
6 |
|
7 |
// Output: <b class="bird-name important">blue-throated macaw</b> |
8 |
console.log(para_bird); |
There is also a possibility that you might want to select multiple elements which will match the specified selectors, you should consider using the querySelectorAll()
method in this case. Here is an example:
let id_class_combo = document.getElementById("intro").getElementsByClassName("important"); |
|
2 |
let para_important = document.querySelectorAll("#intro .important"); |
3 |
|
4 |
// Output: HTMLCollection { 0: b.bird-name.important, 1: b.important, length: 2 } |
5 |
console.log(id_class_combo); |
6 |
|
7 |
// Output: NodeList [ b.bird-name.important, b.important ] |
8 |
console.log(para_important); |
As you can see, we get the same two elements whether we use the getElementsByClassName()
method chained to getElementById()
or directly use the querySelectorAll()
method.
However, there is one big difference. You will get a live HTMLCollection
when you use getElementsByClassName()
but a static NodeList
when you use the querySelectorAll()
method.
You can iterate over either of them to access individual element using for..of
as shown below:
let para_important = document.querySelectorAll("#intro .important"); |
|
2 |
|
3 |
for(elem of para_important) { |
4 |
console.log(elem); |
5 |
} |
6 |
/* Output |
7 |
|
8 |
<b class="bird-name important">blue-throated macaw</b> |
9 |
<b class="important">Bolivia</b> |
*/ |
You can also iterate over a NodeList
by using forEach()
but it won't work with an HTMLCollection
.
Final Thoughts
In this tutorial, we learned about different methods of getting elements from DOM. We can access these elements either by using getElementsByTagName()
, getElementById()
or getElementsByClassName()
. We can also access these elements by using the querySelector()
or querySelectorAll()
method and passing a specific selector.
The first three methods are faster so you can use them when performance is paramount. On the other hand, the query selector methods are easier to use and help you avoid long method call chains.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK