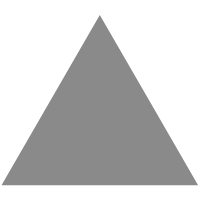
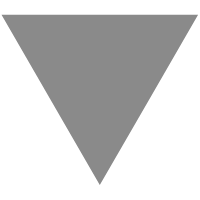
Custom Training of Large Language Models (LLMs): A Detailed Guide With Code Samp...
source link: https://dzone.com/articles/custom-training-of-large-language-models-a-compreh
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
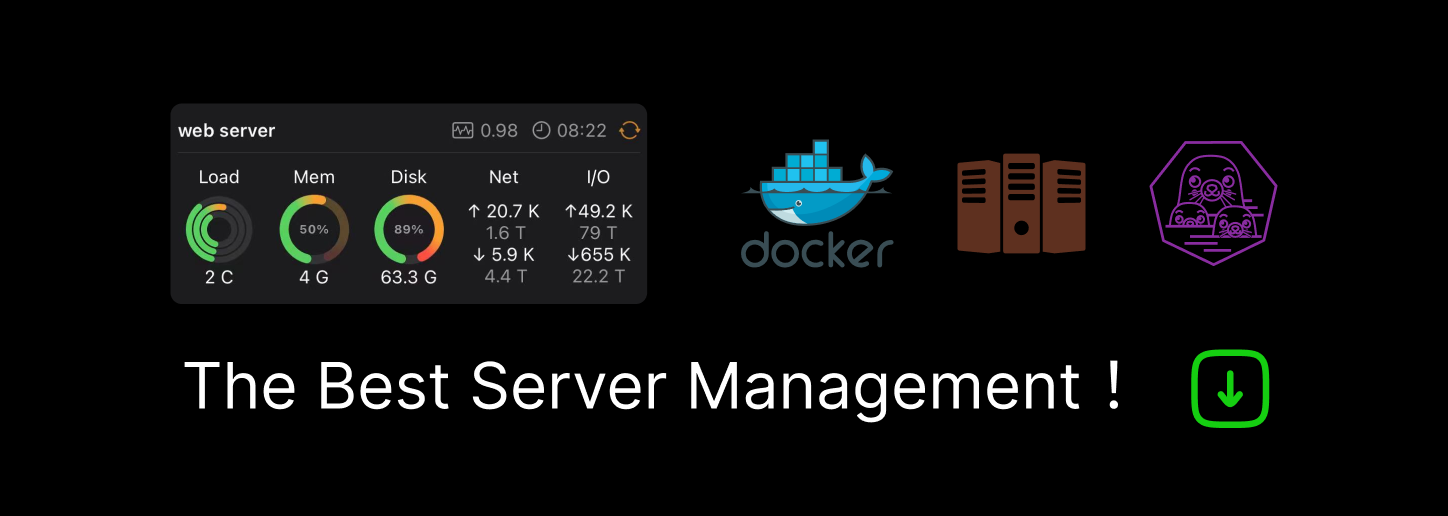
In recent years, large language models (LLMs) like GPT-4 have gained significant attention due to their incredible capabilities in natural language understanding and generation. However, to tailor an LLM to specific tasks or domains, custom training is necessary. This article offers a detailed, step-by-step guide on custom training LLMs, complete with code samples and examples.
Prerequisites
Before diving in, ensure you have:
- Familiarity with Python and PyTorch.
- Access to a pre-trained GPT-4 model.
- Adequate computational resources (GPUs or TPUs).
- A dataset in a specific domain or task for fine-tuning.
Step 1: Prepare Your Dataset
To fine-tune the LLM, you'll need a dataset that aligns with your target domain or task. Data preparation involves:
1.1 Collecting or Creating a Dataset
Ensure your dataset is large enough to cover the variations in your domain or task. The dataset can be in the form of raw text or structured data, depending on your needs.
1.2 Preprocessing and Tokenization
Clean the dataset, removing irrelevant information and normalizing the text. Tokenize the text using the GPT-4 tokenizer to convert it into input tokens.
from transformers import GPT4Tokenizer
tokenizer = GPT4Tokenizer.from_pretrained("gpt-4")
data_tokens = tokenizer(data_text, truncation=True, padding=True, return_tensors="pt")
Step 2: Configure the Training Parameters
Fine-tuning involves adjusting the LLM's weights based on the custom dataset. Set up the training parameters to control the training process:
from transformers import GPT4Config, GPT4ForSequenceClassification
config = GPT4Config.from_pretrained("gpt-4", num_labels=<YOUR_NUM_LABELS>)
model = GPT4ForSequenceClassification.from_pretrained("gpt-4", config=config)
training_args = {
"output_dir": "output",
"num_train_epochs": 4,
"per_device_train_batch_size": 8,
"gradient_accumulation_steps": 1,
"learning_rate": 5e-5,
"weight_decay": 0.01,
}
Replace <YOUR_NUM_LABELS>
with the number of unique labels in your dataset.
Step 3: Set Up the Training Environment
Initialize the training environment using the TrainingArguments
and Trainer
classes from the transformers
library:
from transformers import TrainingArguments, Trainer
training_args = TrainingArguments(**training_args)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=data_tokens
)
Step 4: Fine-Tune the Model
Initiate the training process by calling the train
method on the Trainer
instance:
trainer.train()
This step may take a while depending on the dataset size, model architecture, and available computational resources.
Step 5: Evaluate the Fine-Tuned Model
After training, evaluate the performance of your fine-tuned model using the evaluate
method on the Trainer
instance:
trainer.evaluate()
Step 6: Save and Use the Fine-Tuned Model
Save the fine-tuned model and use it for inference tasks:
model.save_pretrained("fine_tuned_gpt4")
tokenizer.save_pretrained("fine_tuned_gpt4")
To use the fine-tuned model, load it along with the tokenizer:
model = GPT4ForSequenceClassification.from_pretrained("fine_tuned_gpt4")
tokenizer = GPT4Tokenizer.from_pretrained("fine_tuned_gpt4")
Example input text:
input_text = "Sample text to be processed by the fine-tuned model."
Tokenize input text and generate model inputs:
inputs = tokenizer(input_text, return_tensors="pt")
Run the fine-tuned model:
outputs = model(**inputs)
Extract predictions:
predictions = outputs.logits.argmax(dim=-1).item()
Map predictions to corresponding labels:
model = GPT4ForSequenceClassification.from_pretrained("fine_tuned_gpt4")
tokenizer = GPT4Tokenizer.from_pretrained("fine_tuned_gpt4")
# Example input text
input_text = "Sample text to be processed by the fine-tuned model."
# Tokenize input text and generate model inputs
inputs = tokenizer(input_text, return_tensors="pt")
# Run the fine-tuned model
outputs = model(**inputs)
# Extract predictions
predictions = outputs.logits.argmax(dim=-1).item()
# Map predictions to corresponding labels
label = label_mapping[predictions]
print(f"Predicted label: {label}")
Replace label_mapping
with your specific mapping from prediction indices to their corresponding labels. This code snippet demonstrates how to use the fine-tuned model to make predictions on new input text.
While this guide provides a solid foundation for custom training LLMs, there are additional aspects you can explore to enhance the process, such as:
- Experimenting with different training parameters, like learning rate schedules or optimizers, to improve model performance.
- Implementing early stopping or model checkpoints during training to prevent overfitting and save the best model at different stages of training.
- Exploring advanced fine-tuning techniques like layer-wise learning rate schedules, which can help improve performance by adjusting learning rates for specific layers.
- Performing extensive evaluation using metrics relevant to your task or domain, and using techniques like cross-validation to ensure model generalization.
- Investigating the usage of domain-specific pre-trained models or pre-training your model from scratch if the available LLMs do not cover your specific domain well.
By following this guide and considering the additional points mentioned above, you can tailor large language models to perform effectively in your specific domain or task. Please reach out to me for any questions or further guidance.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK