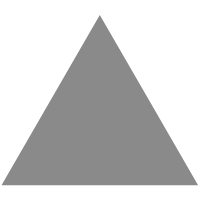
1
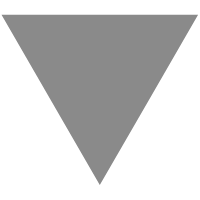
深度学习--PyTorch定义Tensor以及索引和切片 - 林每天都要努力
source link: https://www.cnblogs.com/ssl-study/p/17336833.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
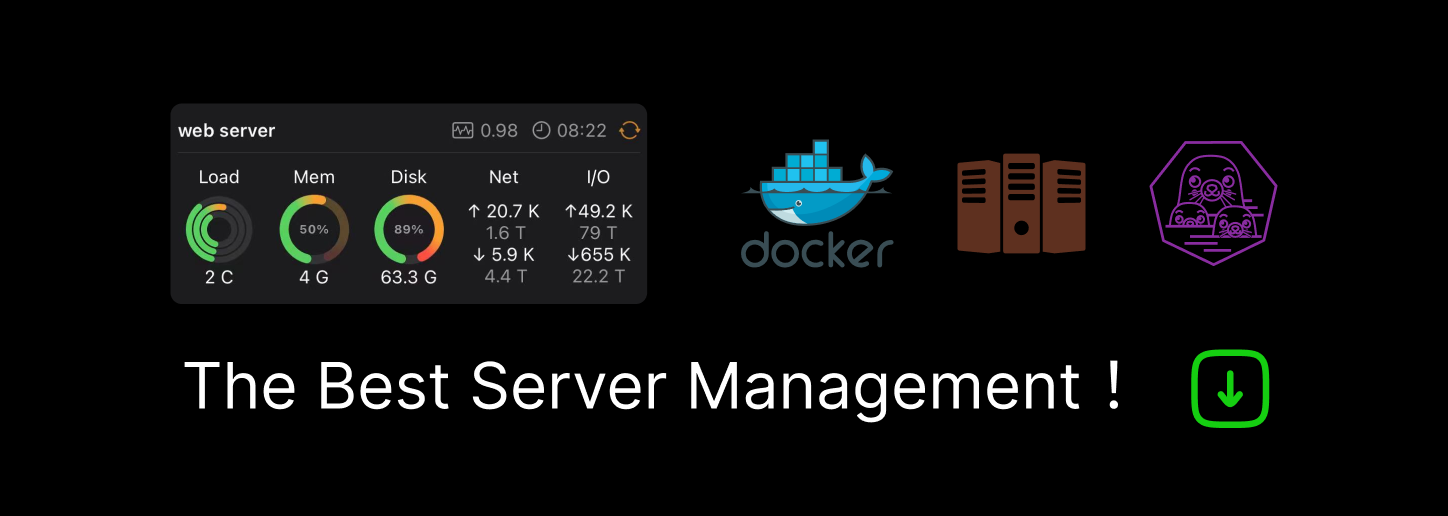
深度学习--PyTorch定义Tensor
一、创建Tensor
1.1未初始化的方法
这些方法只是开辟了空间,所附的初始值(非常大,非常小,0),后面还需要我们进行数据的存入。
- torch.empty():返回一个没有初始化的Tensor,默认是FloatTensor类型。
#torch.empty(d1,d2,d3)函数输入的是shape torch.empty(2,3,5) #tensor([[[-1.9036e-22, 6.8944e-43, 0.0000e+00, 0.0000e+00, -1.0922e-20],# [ 6.8944e-43, -2.8812e-24, 6.8944e-43, -5.9272e-21, 6.8944e-43],# [ 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00]],## [[ 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00],# [ 0.0000e+00, 0.0000e+00, 1.4013e-45, 0.0000e+00, 0.0000e+00],# [ 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00, 0.0000e+00]]])
- torch.FloatTensor():返回没有初始化的FloatTensor。
#torch.FloatTensor(d1,d2,d3)torch.FloatTensor(2,2) #tensor([[-0.0000e+00, 4.5907e-41],# [-7.3327e-21, 6.8944e-43]])
- torch.IntTensor():返回没有初始化的IntTensor。
#torch.IntTensor(d1,d2,d3)torch.IntTensor(2,2) #tensor([[ 0, 1002524760],# [-1687359808, 492]], dtype=torch.int32)
1.2 随机初始化
-
随机均匀分布:rand/rand_like,randint
rand:[0,1)均匀分布;randint(min,max,[d1,d2,d3]) 返回[min,max)的整数均匀分布
#torch.rand(d1,d2,d3)torch.rand(2,2) #tensor([[0.8670, 0.6158],# [0.0895, 0.2391]]) #rand_like()a=torch.rand(3,2)torch.rand_like(a) #tensor([[0.2846, 0.3605],# [0.3359, 0.2789],# [0.5637, 0.6276]]) #randint(min,max,[d1,d2,d3])torch.randint(1,10,[3,3,3]) #tensor([[[3, 3, 8],# [2, 7, 7],# [6, 5, 9]],## [[7, 9, 9],# [6, 3, 9],# [1, 5, 6]],## [[5, 4, 8],# [7, 1, 2],# [3, 4, 4]]])
-
随机正态分布 randn
randn返回一组符合N(0,1)正态分布的随机数据
#randn(d1,d2,d3)torch.randn(2,2) #tensor([[ 0.3729, 0.0548],# [-1.9443, 1.2485]]) #normal(mean,std) 需要给出均值和方差torch.normal(mean=torch.full([10],0.),std=torch.arange(1,0,-0.1)) #tensor([-0.8547, 0.1985, 0.1879, 0.7315, -0.3785, -0.3445, 0.7092, 0.0525, 0.2669, 0.0744])#后面需要用reshape修正成自己想要的形状
1.3 赋值初始化
- full:返回一个定值
#full([d1,d2,d3],num)torch.full([2,2],6) #tensor([[6, 6],# [6, 6]]) torch.full([],6)#tensor(6) 标量 torch.full([1],6)#tensor([6]) 向量
- arange:返回一组阶梯,等差数列
#torch.arange(min,max,step):返回一个[min,max),步长为step的集体数组,默认为1torch.arange(0,10) #tensor([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) torch.arange(0,10,2)#tensor([0, 2, 4, 6, 8])
- linspace/logspace:返回一组阶梯
#torch.linspace(min,max,steps):返回一个[min,max],数量为steps的数组torch.linspace(1,10,11) #tensor([ 1.0000, 1.9000, 2.8000, 3.7000, 4.6000, 5.5000, 6.4000, 7.3000,# 8.2000, 9.1000, 10.0000]) #torch.logspace(a,b,steps):返回一个[10^a,10^b],数量为steps的数组torch.logspace(0,1,10) #tensor([ 1.0000, 1.2915, 1.6681, 2.1544, 2.7826, 3.5938, 4.6416, 5.9948,# 7.7426, 10.0000])
- ones/zeros/eye:返回全1全0或者对角阵 ones_like/zeros_like
#torch.ones(d1,d2)torch.ones(2,2) #tensor([[1., 1.],# [1., 1.]]) #torch.zeros(d1,d2)torch.zeros(2,2) #tensor([[0., 0.],# [0., 0.]]) #torch.eye() 只能接收一个或两个参数torch.eye(3) #tensor([[1., 0., 0.],# [0., 1., 0.],# [0., 0., 1.]]) torch.eye(2,3) #tensor([[1., 0., 0.],# [0., 1., 0.]])
1.4 随机打散变量
- randperm:一般用于位置操作。类似random.shuffle()。
torch.randperm(8)#tensor([2, 6, 7, 5, 3, 4, 1, 0])
二、索引与切片
- 简单索引方式
a=torch.rand(4,3,28,28)a[0].shape#torch.Size([3, 28, 28])a[0,0,0,0]#tensor(0.9373)
- 批量索引方式 开始位置:结束位置 左边取的到,右边取不到 算是一种切片 [0,1,2]->[-3,-2,-1]
a[:2].shape#torch.Size([2, 3, 28, 28])a[1:].shape#torch.Size([3, 3, 28, 28])
- 隔行采样方式 开始位置:结束位置:间隔
a[:,:,0:28:2,:].shape#torch.Size([4, 3, 14, 28])
- 任意取样方式 a.index_select(d,[d层的数据索引])
a.index_select(0,torch.tensor([0,2])).shape#torch.Size([2, 3, 28, 28]) a.index_select(1,torch.tensor([0,2])).shape#torch.Size([4, 2, 28, 28])
- ...任意维度取样
a[...].shape#torch.Size([4, 3, 28, 28]) a[0,...].shape#torch.Size([3, 28, 28]) a[:,2,...].shape#torch.Size([4, 28, 28])
- 掩码索引mask x.ge(0.5) 表示大于等于0.5的为1,小于0.5的为0
#torch.masked_select 取出掩码对应位置的值x=torch.randn(3,4)mask=x.ge(0.5)torch.masked_select(x,mask) #tensor([1.6950, 1.2207, 0.6035])
- 具体索引 take(变量,位置) 会把变量变为一维的
x=torch.randn(3,4)torch.take(x,torch.tensor([0,1,5])) #tensor([-2.2092, -0.2652, 0.4848])
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK