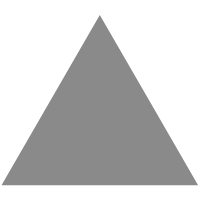
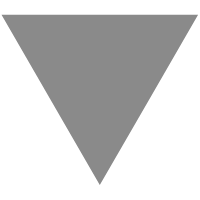
Building RESTful APIs with Node.js and Express
source link: https://abhishekdhapare.hashnode.dev/building-restful-apis-with-nodejs-and-express
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
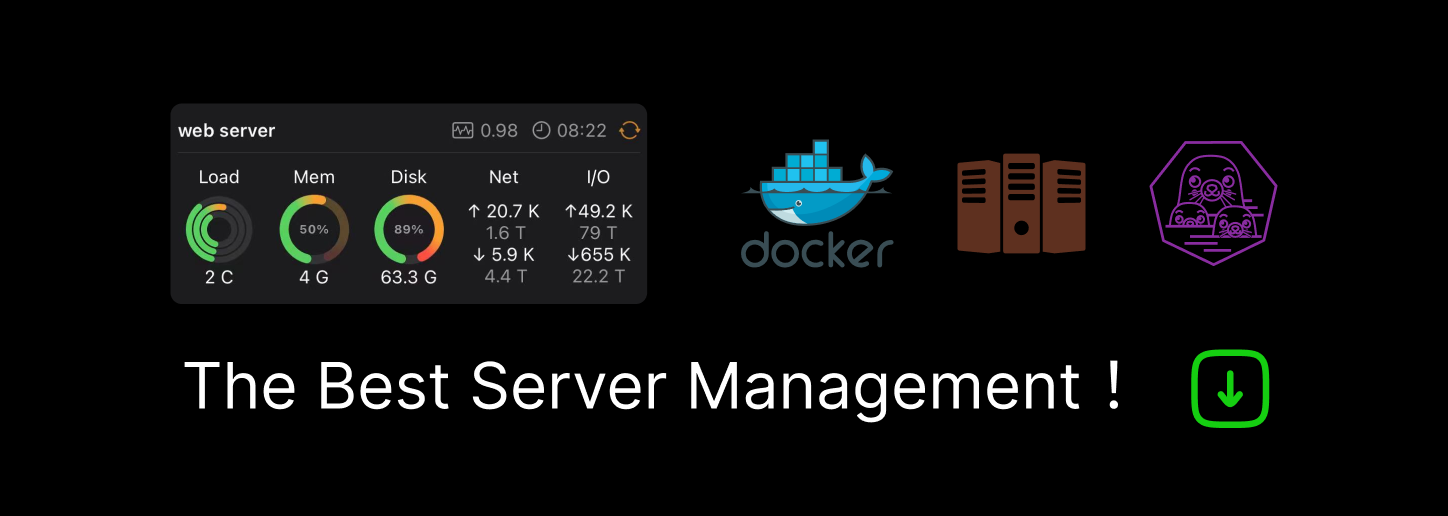
What is Rest API?
REST (Representational State Transfer) API is a software architecture style that defines a set of constraints and principles for building web services. RESTful APIs are used to build web services that are scalable, maintainable, and easy to consume.
REST API is based on HTTP protocol and uses the HTTP methods (GET, POST, PUT, DELETE, etc.) to access resources and manipulate their representations. A resource can be anything that is identified by a URI (Uniform Resource Identifier) such as a file, a document, a database record, or a collection of records.
REST API is stateless, meaning that each request from the client to the server contains all the necessary information to complete the request, and the server does not store any client context between requests. This makes REST API simple, flexible, and easy to cache, which helps improve performance.
RESTful APIs typically return data in a standard format such as JSON (JavaScript Object Notation) or XML (Extensible Markup Language), making them easy to consume by any client that understands the format. REST API is widely used for building web services that are used by mobile apps, web apps, and other client-side applications.
Folder Structure
When building a REST API with Node.js and Express, there are many different ways to structure your folders and files. However, here's an example of a common folder structure:
- app
- controllers
- user.js
- models
- user.js
- routes
- index.js
- user.js
- utils
- auth.js
- config
- database.js
- node_modules
- public
- .env
- .gitignore
- index.js
- package.json
- package-lock.json
Here's a brief explanation of each folder and file:
app: This folder contains the core application code.
controllers: This folder contains the logic for handling requests and responses. For example, user.js might contain functions for handling CRUD operations on user data. models: This folder contains the database schema and functions for interacting with the database. For example, user.js might define the schema for a user and contain functions for finding, creating, updating, and deleting user data. routes: This folder contains the routing logic for the API endpoints. For example, user.js might define the routes for CRUD operations on user data, and index.js might define the root endpoint. utils: This folder contains utility functions that can be used throughout the application. For example, auth.js might contain functions for verifying user authentication and authorization. config: This folder contains configuration files for the application. For example, database.js might contain the configuration settings for connecting to a database.
node_modules: This folder contains the dependencies installed by npm.
public: This folder contains static files that can be served by the API. For example, images, CSS, or JavaScript files.
.env: This file contains environment variables that are specific to the local development environment.
.gitignore: This file specifies which files and folders should be ignored by git.
index.js: This file is the entry point for the application, and it sets up the server and middleware.
package.json: This file specifies the dependencies and metadata for the application.
package-lock.json: This file contains the exact versions of the dependencies that were installed by npm, to ensure consistency across different environments.
This folder structure is just one example, and you may need to modify it based on your specific requirements.
Build REST API
To write a REST API in Node.js using Express and MySQL, follow these steps:
Install the required packages:
npm install express mysql body-parser cors
Create a new Express application:
const express = require('express'); const app = express();
Connect to your MySQL database:
const mysql = require('mysql'); const connection = mysql.createConnection({ host: 'localhost', user: 'root', password: 'password', database: 'database_name' }); connection.connect();
Add middleware to parse incoming request bodies and enable CORS:
const bodyParser = require('body-parser'); const cors = require('cors'); app.use(bodyParser.json()); app.use(cors());
Define your API endpoints:
app.get('/users', (req, res) => { connection.query('SELECT * FROM users', (error, results) => { if (error) { res.status(500).send(error); } else { res.send(results); } }); }); app.post('/users', (req, res) => { const { name, email } = req.body; connection.query('INSERT INTO users (name, email) VALUES (?, ?)', [name, email], (error, results) => { if (error) { res.status(500).send(error); } else { res.send(results); } }); });
Start the server:
app.listen(3000, () => { console.log('Server listening on port 3000'); });
This is just a basic example, and you can customize it according to your specific requirements. Also, make sure to handle errors and sanitize input data to prevent security issues. Will talk more about it in the next blog.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK