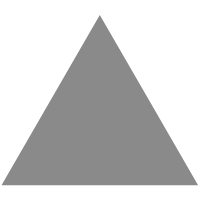
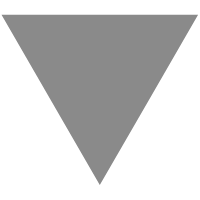
Understanding Object-Oriented Programming Concepts in JavaScript
source link: https://abhishekdhapare.hashnode.dev/understanding-object-oriented-programming-concepts-in-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
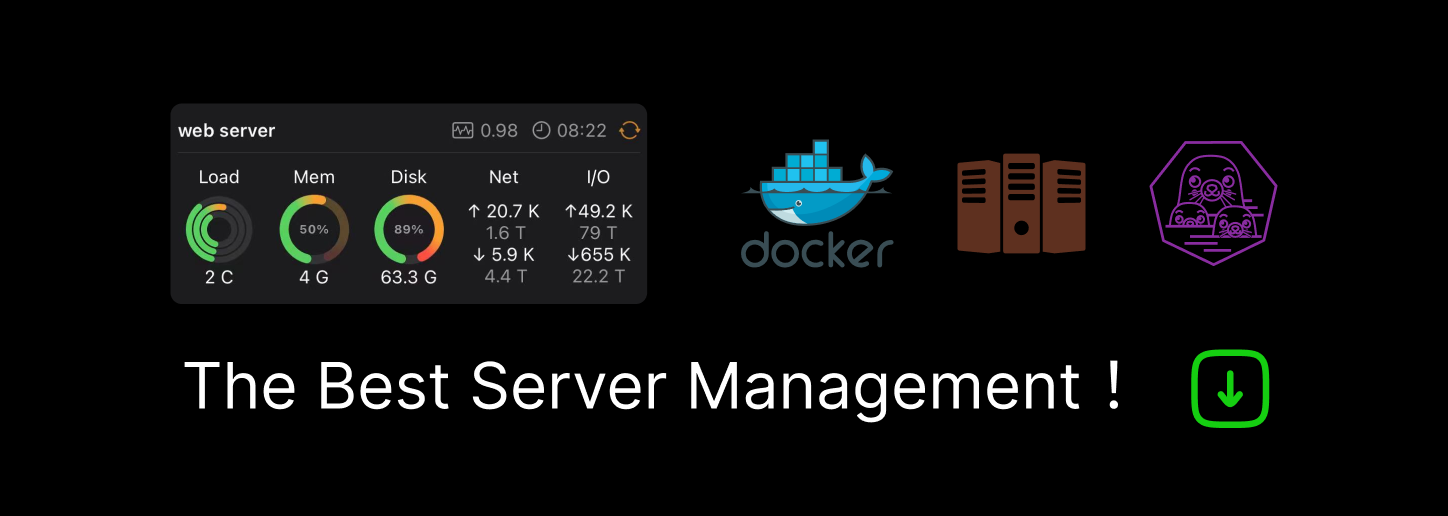
Object-Oriented Programming (OOP) is a programming paradigm that focuses on the use of objects to organize and structure code. OOP provides a way to represent real-world entities and their relationships in code. JavaScript is a language that supports OOP, and in this article, we'll explore the key OOP concepts in JavaScript, including objects, classes, and inheritance.
Objects in JavaScript
In JavaScript, objects are collections of key-value pairs, where the key is a string or symbol, and the value can be any valid JavaScript value, including functions, objects, and primitive values. Objects can be created using object literals or the Object()
constructor.
Here's an example of creating an object using an object literal:
const person = {
name: 'John',
age: 30,
greet: function() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
}
};
person.greet(); // Output: Hello, my name is John and I'm 30 years old.
In this example, we create a person
object with three properties: name
, age
, and greet
. The greet
property is a function that logs a message to the console.
Classes in JavaScript
JavaScript also supports the class
keyword, which provides syntactic sugar for creating constructor functions and prototype-based inheritance. Classes in JavaScript are based on prototypes, which are objects that serve as templates for creating other objects.
Here's an example of creating a class in JavaScript:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
}
}
const person = new Person('John', 30);
person.greet(); // Output: Hello, my name is John and I'm 30 years old.
In this example, we create a Person
class with a constructor function that takes two parameters: name
and age
. We also define a greet
method that logs a message to the console. We then create a new person
object using the new
keyword and pass in two arguments to the constructor.
Inheritance in JavaScript
JavaScript supports inheritance through the use of prototype chains. In a prototype chain, objects inherit properties and methods from their prototypes, which can in turn inherited from other prototypes.
Here's an example of inheritance in JavaScript:
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
constructor(name) {
super(name);
}
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Fido');
dog.speak(); // Output: Fido barks.
In this example, we create an Animal
class with a speak
method that logs a message to the console. We then create a Dog
class that extends the Animal
class and overrides the speak
method. We create a new dog
object using the new
keyword and call its speak
method.
Conclusion
OOP is a powerful programming paradigm that can help organize and structure code. In JavaScript, we can use objects, classes, and inheritance to achieve this. By using these concepts, we can write cleaner, more modular, and more maintainable code. Whether you're building a small script or a large-scale application, understanding OOP concepts in JavaScript is essential for writing high-quality code.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK