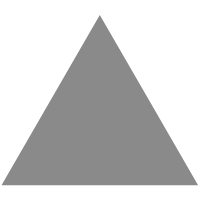
3
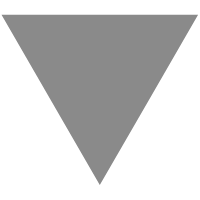
QT实现可拖动自定义控件 - 熊来闯一闯
source link: https://www.cnblogs.com/xionglaichuangyichuang/p/17294378.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
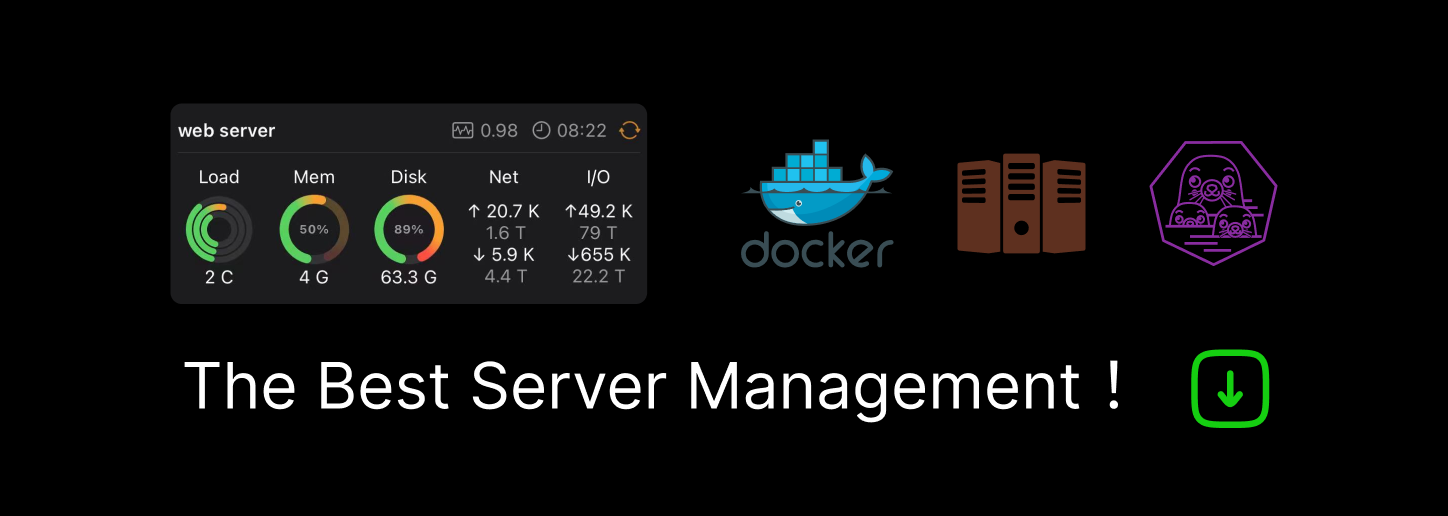
QT实现可拖动自定义控件
使用QT实现自定义类卡牌控件Card,使其能在父类窗口上使用鼠标进行拖动。
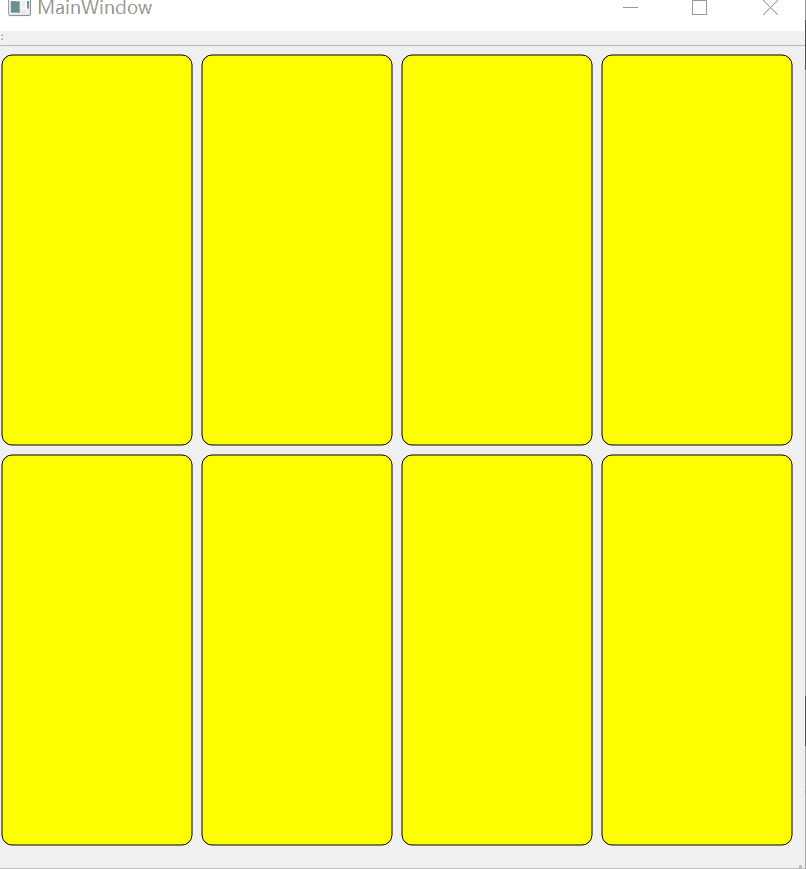
控件类头文件card.h
#ifndef CARD_H #define CARD_H #include <QWidget> #include <QPaintEvent> #include <QPainter> #include <QMouseEvent> class Card:public QWidget { Q_OBJECT public: explicit Card(QWidget *parent = nullptr); ~Card(); protected: void paintEvent(QPaintEvent *event) override; void mousePressEvent(QMouseEvent *event) override; signals: void sendSelf(Card *w); }; #endif // CARD_H
控件类头文件card.cpp
#include "card.h" Card::Card(QWidget *parent):QWidget(parent) { this->setGeometry(0,0,200,400); //设置控件窗口大小 } Card::~Card() { } void Card::paintEvent(QPaintEvent *event) { QPainter painter(this); //创建画笔 painter.setRenderHint(QPainter::Antialiasing,true); QBrush brush; //创建笔刷 brush.setColor(Qt::yellow); //设置笔刷颜色 brush.setStyle(Qt::SolidPattern); painter.setBrush(brush); //设置画笔笔刷 painter.drawRoundedRect(QRectF(5,5,190,390),10,10); //绘制圆角矩形并填充 } void Card::mousePressEvent(QMouseEvent *event) { Q_UNUSED(event); emit sendSelf(this); //信号发送该控件地址 }
以上是基于widget类的控件类;
父窗口头文件mainwindow.h
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include "card.h" #include <QDebug> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private: Ui::MainWindow *ui; Card* cd[8]; Card *temp; QPoint startP; QPoint yuanP; private slots: void getObject(Card *w); protected: void mouseMoveEvent(QMouseEvent *event) override; void mouseReleaseEvent(QMouseEvent *event) override; }; #endif // MAINWINDOW_H
父窗口头文件mainwindow.cpp
#include "mainwindow.h" #include "ui_mainwindow.h" MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); for(int i=0;i<8;i++) { cd[i] = new Card(this); connect(cd[i],&Card::sendSelf,this,&MainWindow::getObject); cd[i]->move(i%4*200,i/4*400+20); } } MainWindow::~MainWindow() { delete ui; } //获取控件坐标 void MainWindow::getObject(Card *w) { temp = w; startP = cursor().pos()-this->pos(); yuanP = temp->pos(); /*将此小部件提升到父小部件堆栈的顶部*/ temp->raise(); } //移动事件 void MainWindow::mouseMoveEvent(QMouseEvent *event) { temp->move(yuanP.x()+event->x()-startP.x(),yuanP.y()+event->y()-startP.y()); } //拖拽对象置顶,卡牌积压的时候,拖动的那张卡牌置顶 void MainWindow::mouseReleaseEvent(QMouseEvent *event) { temp->raise(); }
以下是工程源码:
链接:https://pan.baidu.com/s/1YH95FzZV7_idanob2qeHig
提取码:wbsy
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK