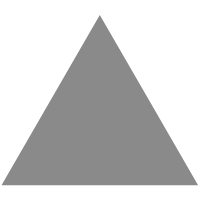
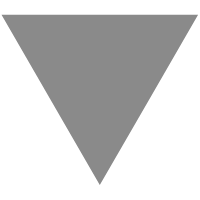
.net core基于HttpClient实现的网络请求库 - 胖纸不争
source link: https://www.cnblogs.com/donpangpang/p/17279574.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
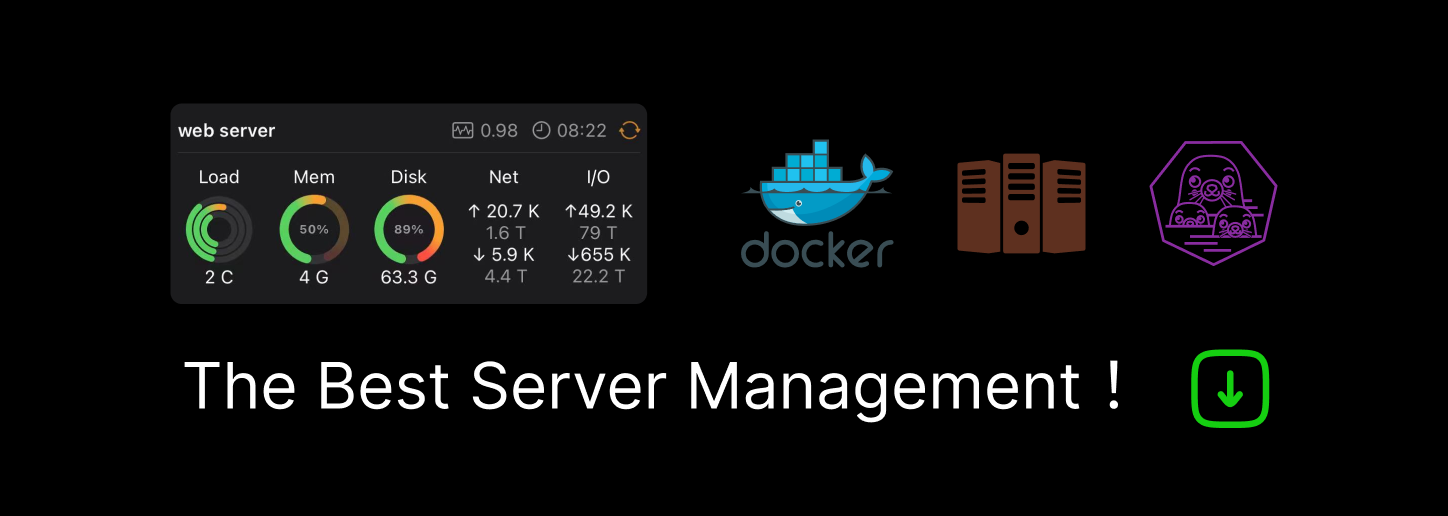
Soda.Http
基于HttpClient
封装的 Http 请求库。如果有什么好想法,可以提 Issue 或者 Pr。,如果想要使用,直接在nuget
搜索Soda.Http
即可。
Github项目地址:Soda.Http
预载配置并不是必须的,但是有助于我们进行一些通用基础设置,例如Headers、Accept、BaseUrl等等。
配置BaseUrl
之后,可以直接使用QSodaHttp.Uri()
代替QSodaHttp.Url()
,直接填写接口即可。
在AspNetCore
中:
services.AddSodaHttp(opts =>
{
opts.BaseUrl = "http://localhost:8080/";
opts.Accept = new[]
{
"application/json",
"text/plain",
"*/*"
};
opts.EnableCompress = false;
opts.Headers = new[]{
("X-Ca-Test", "key")
};
});
较为通用的写法,程序构建时:
QSodaHttp.AddSodaHttp(opts =>
{
opts.BaseUrl = "http://localhost:8080/";
opts.Accept = new[]
{
"application/json",
"text/plain",
"*/*"
};
opts.EnableCompress = false;
opts.Headers = new[]{
("X-Ca-Test", "key")
};
})
2 全局配置 Authentication
有时需要全局配置 Authentication,如果在代码中请求中独立配置了 Authentication 则会覆盖全局 Authentication
QSodaHttp.InitAuthentication("Bearer", "Values");
如果你是塞到 Header 里的这种做法
QSodaHttp.AddHeader("X-Ca-Key", "Values");
3 Http 请求
3.1 QSodaHttp
API 示例:
var result = await QSodaHttp.Url("https://www.baidu.com/")
.Header("X-Ca-Key", "XXX")
.Authentication("Bearer", "XXX")
.Params(new { Id = "123456" })
.Body(new { })
// .Form(...)
// .File(...)
.PostAsync<string>();
简单示例:
// 配置BaseUrl
var services = new ServiceCollection();
services.AddSodaHttp(opts =>
{
opts.EnableCompress = false;
opts.BaseUrl = "http://localhost:5050/";
});
var res = await QSodaHttp.Uri("/Test/Get").Params(new { Id = "123456" }).GetAsync<object>();
var res = await QSodaHttp.Uri("/Test/TestGetResult").Params(new { Id = "123456", Ids = new[] { "123", "456" } }).GetAsync<object>();
var res = await QSodaHttp.Uri("/Test/Post").Body(new { Id = "123456", Ids = new[] { "123", "456" } }).PostAsync<object>();
var res = await QSodaHttp.Uri("/Test/PostResult")
.Params(new { Id = "123456", Ids = new[] { "123", "456" } })
.Body(new { Id = "123456", Ids = new[] { "123", "456" } })
.PostAsync<object>();
var res = await QSodaHttp.Uri("/Test/Delete").Params(new { Id = "123456" }).DeleteAsync<object>();
var res = await QSodaHttp.Uri("/Test/DeleteResult").Params(new { Id = "123456", Ids = new[] { "123", "456" } }).DeleteAsync<object>();
var res = await QSodaHttp.Uri("/Test/Put").Params(new { Id = "123456" }).PutAsync<object>();
var res = await QSodaHttp.Uri("/Test/PutResult")
.Body(new { Id = "123456", Ids = new[] { "123", "456" } })
.PutAsync<object>();
var res = await QSodaHttp.Uri("Patch").Params(new { Id = "123456" }).PatchAsync<object>();
var res = await QSodaHttp.Uri("PatchResult")
.Body(new { Id = "123456", Ids = new[] { "123", "456" } })
.PatchAsync<object>();
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK