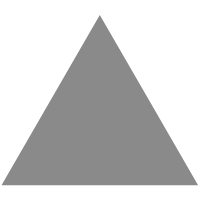
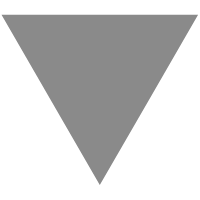
Laravel 10: Features at a Glance
source link: https://devm.io/php/php-laravel-10-features
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
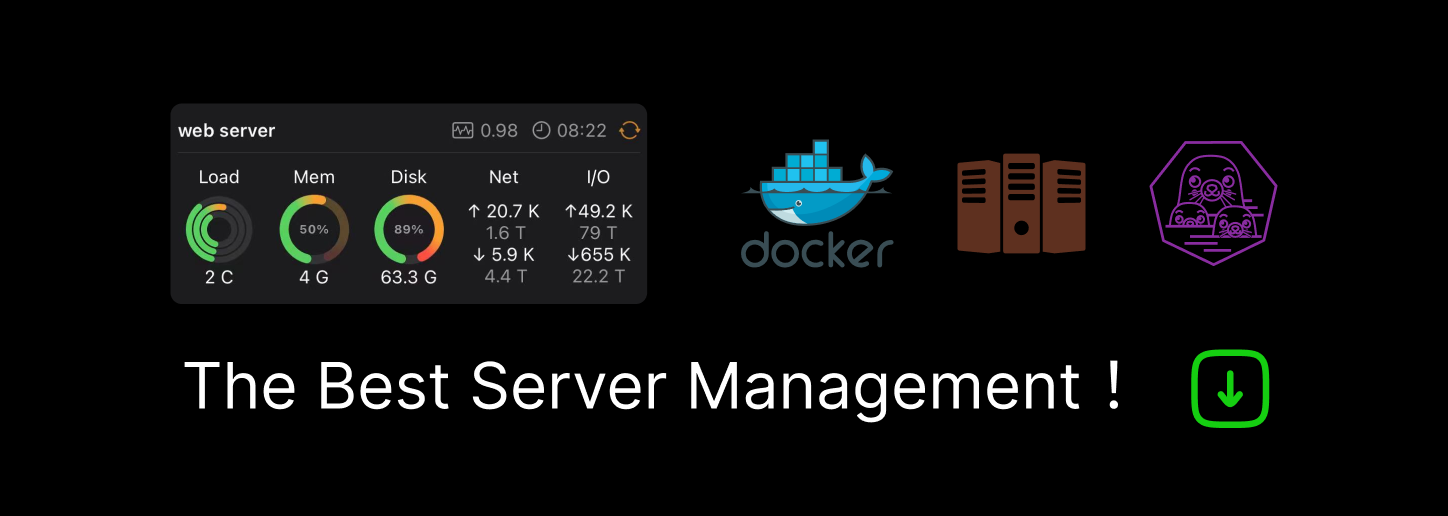
Version 10 of the PHP framework Laravel was released on February 14, 2023. The highlights mainly include Laravel Pennant — a system for managing feature flags — stronger typing, and measuring the performance of unit tests.
The most important innovations summarized
- Laravel Pennant: Feature flag management system
- Stronger typing
- Facade for better interaction with external processes
- More support for the Artisan Make command
- You can now measure the performance of unit tests
- New helper for generating passwords
- Facelift for Laravel Horizon and Telescope
- PHP 8.0 is no longer supported
- Doctrine/dbal is no longer needed
Stronger type hinting
In Laravel 10, type hints have been added to most methods. When generating new controllers, they are now supplied by default:
TaskController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
class TaskController extends Controller
{
public function index(): Response
{
//
}
}
While upgrading our test project, we needed to make minor adjustments to the typing. In this instance, the parameters that we passed were actually incorrect. Normally, the conversion should work without any problems here.
Specifically, the following type-hints have been added to Laravel:
- Return types
- Method parameters
With generated controllers, the return type may have to be changed. This can happen when returning a view, for example. If you don’t want to do this, you can adapt the stubs — the templates for class generation — yourself. For this, you can use “php artisan stub:publish” to publish the stubs first. This creates a new folder /stubs with the templates for code generation. You can remove or modify the types here.
Laravel Pennant: System for Feature Flags
With Laravel Pennant, you can enable or disable features in the project with flags. It has many different use cases:
- Developers can test features in the live system before access is active for everyone
- You can release certain features only to beta users giving you initial feedback.
- You can release features depending on the server (for instance: staging, live environment, or even different customer systems)
- Features can also be unlocked through selected subscription models. Then, some features will only be available for premium users.
- If you work with A/B testing, you can activate a feature for half of your users and see how key figures change for them.
For testing, a feature can be defined in the AppServiceProvider, for example:
AppServiceProvider.php
…
public function boot() {
Feature::define("reporting",function(){
return Auth::user()?->is_admin;
});
}
…
Now you can use different ways to check whether the function “reporting” is active. You can do this with an if-condition:
TestController.php
…
if(Feature::active("reporting")) {
dd("active");
}
…
But testing is also possible in blade templates:
reporting.blade.php
…
@feature('reporting')
aktiv
@else
inaktiv
@endfeature
…
Laravel Pennant also has a trait called HasFeatures, which can be used to assign features to a user. You can use $user->features->active($feature) to check if this feature is active for a user.
When features grow out of the beta stage, they can be easily activated for all users through a migration. The corresponding function is called Feature::activateForEveryone.
The features are stored in the database by default, but it’s also possible to store it as an array.
Process Facade
Laravel 10 adds a new facade that makes it easier to interact with external processes. For example, if you want to know which processes are currently running on the server, you can display them like this:
ProcessController.php
…
$process = Process::run("ps -aux");
dd($process->output());
…
The external command is executed with run. The output fetches according to the result as a string.
You can also execute processes in parallel:
ProcessController.php
…
[$first, $second, $third] = Process::concurrently(function (Pool $pool) {
$pool->command('echo 1');
$pool->command('echo 2');
$pool->command('echo 3');
});
dd($first->output(),$second->output(),$third->output());
…
It waits accordingly until all processes are completed. The facade can also be used to check external processes in the unit tests. In addition to the output, process...
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK