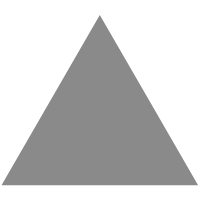
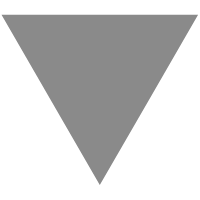
How to get form data in jQuery using serialize and serializeArray Method
source link: https://www.laravelcode.com/post/how-to-get-form-data-in-jquery-using-serialize-and-serializearray-method
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
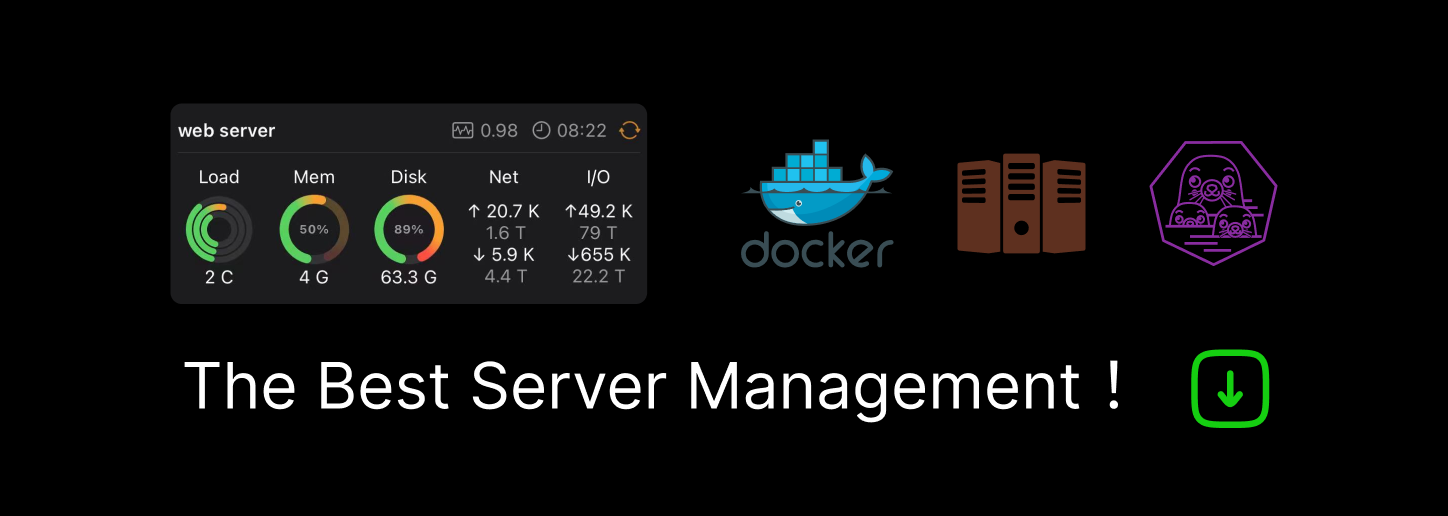
How to get form data in jQuery using serialize and serializeArray Method
jQuery serialize() method used to create a url encoded query string from form field values. This is used to send Ajax request by creating serialize data on click or load event. This way, you don't need to get one by one input value and send it to Ajax request.
Syntax:
$(selector).serialize();
Below example output the query string data of form fields when button clicks.
<!DOCTYPE html>
<html>
<body>
<form>
<input type="text" name="name" value="Harsukh">
<input type="email" name="email" value="[email protected]">
<button type="button" id="button">Submit</button>
<p id="value"></p>
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$('#button').click(function() {
var data = $('form').serialize();
$('#value').text(data);
});
</script>
</body>
</html>
In another way, jQuery serializeArray() method used to creates an array of objects from field values. This is similar to serialize() method except it creates array of object instead of query string. This method is usedful in sending Ajax request where json format data is required.
Syntax:
$(selector).serializeArray();
You can find the below example of use of serializeArray() method.
<!DOCTYPE html>
<html>
<body>
<form>
<input type="text" name="name" value="Harsukh">
<input type="email" name="email" value="[email protected]">
<button type="button" id="button">Submit</button>
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$('#button').click(function() {
var data = $('form').serializeArray();
console.log(data); // [{name:"name",value:"Harsukh"},{name:"email",value:"[email protected]"}]
});
</script>
</body>
</html>
Extra:
Ajax json data should be in key: value format. To create one level json data, you can use the below function.
function serializeObject(obj) {
var jsn = {};
$.each(obj, function() {
if (jsn[this.name]) {
if (!jsn[this.name].push) {
jsn[this.name] = [jsn[this.name]];
}
jsn[this.name].push(this.value || '');
} else {
jsn[this.name] = this.value || '';
}
});
return jsn;
};
This will return simple json object for the above example. If you want to add more data into json before Ajax request send, you can use Javascript push() method to add more data into json and send Ajax.
console.log(serializeObject(data)); // {name: "Harsukh", email: "[email protected]"}
I hope it will help you.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK