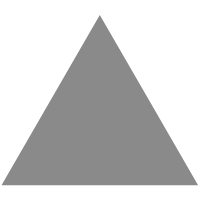
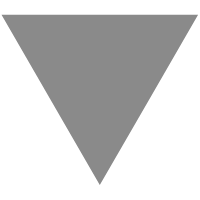
Convert Char Array to an integer in C++
source link: https://thispointer.com/convert-char-array-to-an-integer/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
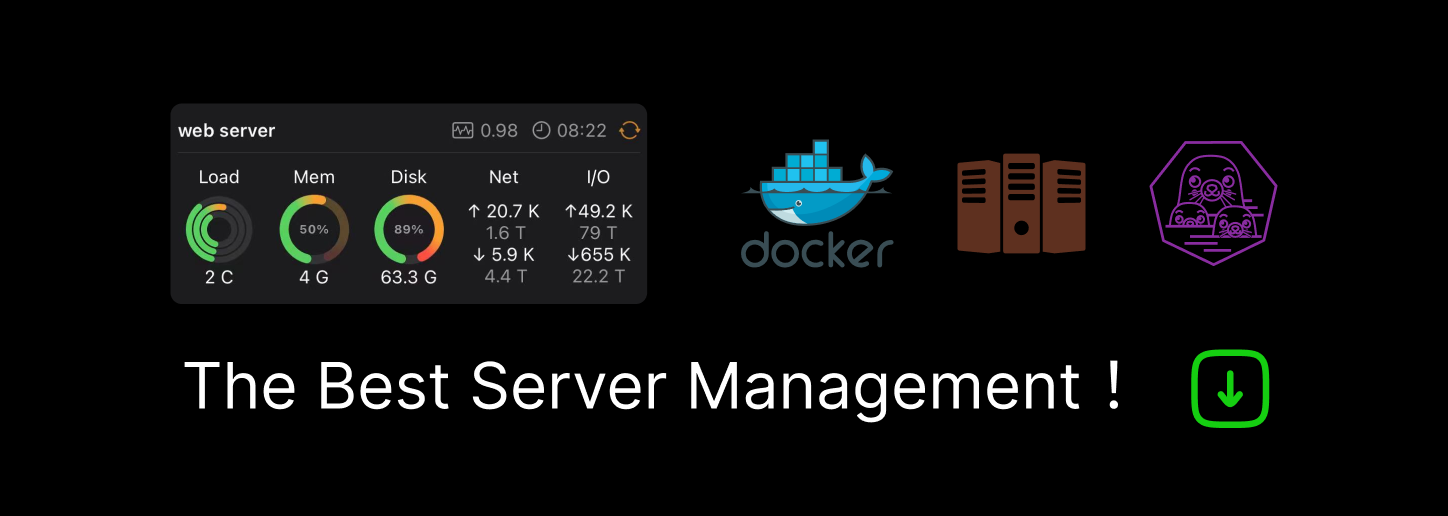
Convert Char Array to an integer in C++ – thisPointer
This tutorial will discuss about a unique way to convert char array to an integer.
In C++, the string.h
header file provides a function stoi()
. It accepts a character pointer as an argument, and interprets its contents as an integer value. It returns an int value. We can use this function to convert a char array to integer.
If the given string can not be converted into an integer value or its out of range, then it will raise an exception i.e. either invalid_argument
or out_of_range
exception. Therefore, while using the stoi()
function for converting a char array to int, we need to make sure that we catch all the exceptions.
Let’s see the complete example,
#include <iostream> #include <string.h> // Returns true, string is successfully conveted // to integer bool ConverToInt(const char* arr, int& value ) { bool result = false; try { // convert char array to string value = std::stoi( arr); result = true; } catch( const std::exception& ) {} return result; } int main() { char arr[10] = "234"; int value = 9; // Convert char array to integer if(ConverToInt(arr, value) ) { std::cout<<"Integer Value is : " << value << std::endl; } else { std::cout<<"Char Array can not be converted to an integer \n"; } return 0; }
Advertisements
Output :
Integer Value is : 234
Summary
Today we learned about several ways to convert char array to an integer. Thanks.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK