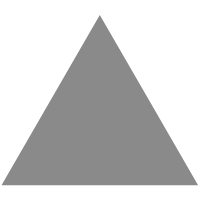
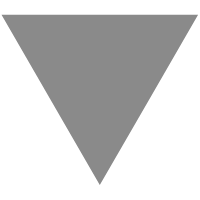
How To Extract a ZIP File and Remove Password Protection in Java
source link: https://dzone.com/articles/how-to-extract-a-zip-file-and-remove-password-prot
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
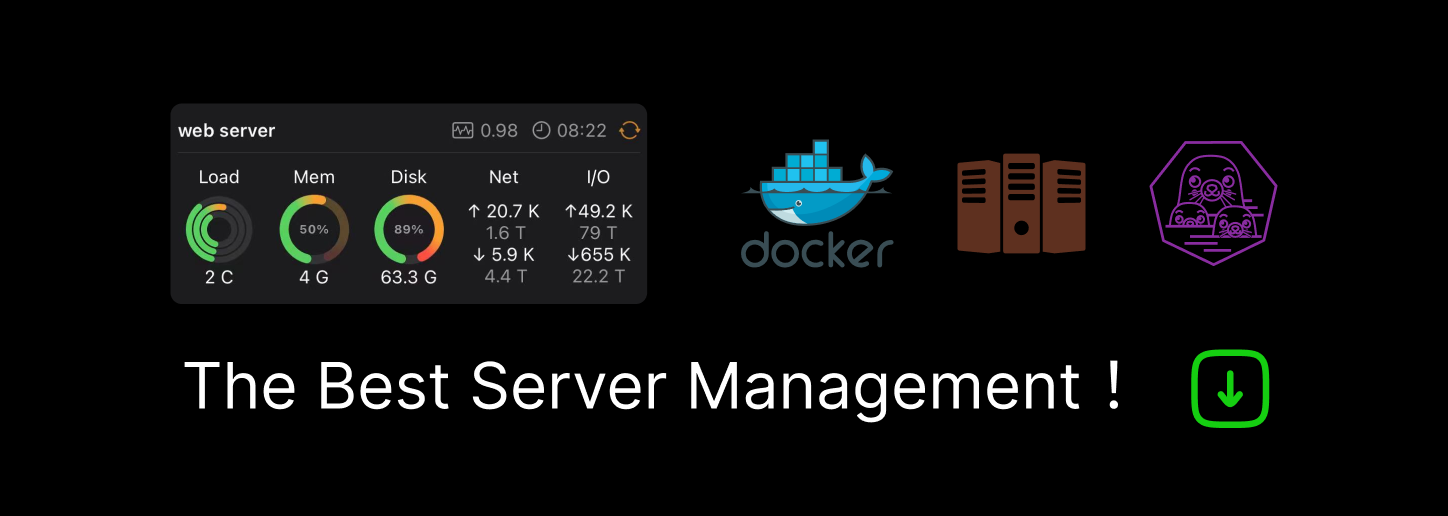
How To Extract a ZIP File and Remove Password Protection in Java
In this tutorial, learn how to automate ZIP file extraction in Java using two APIs with ready-to-run Java code examples provided.
Creating ZIP files is, by design, a simple and highly intuitive process. Users on any major operating system (Windows, Linux, Mac, etc.) can combine numerous folders full of bulky files in just a few clicks, instantly reducing bundles of documents into manageable, compressed archives which makes storing and sharing that content far less burdensome. Opening ZIP files is just as easy: a downstream recipient of a ZIP file only needs to download and extract (or “unzip”) the file’s contents to access the content contained within. Password protection is the icing on the cake: anyone can quickly secure their ZIP archive with a personalized password, ensuring only those with exact permissions can access the archive’s contents.
This ease of use has made .zip an extremely popular solution in both limited and large-scale file-sharing scenarios. One person might create a single ZIP archive with 10 files they need to share with their colleague, while another might create dozens of ZIP files containing hundreds of files each, with multiple layers of directories in each file.
In the former scenario, manually downloading, opening, and accessing the ZIP file is quite efficient. After all, it’s only a limited use case, and the few seconds spent downloading and extracting (or simply saving) a single ZIP archive is hardly a burden or a distraction from other tasks. In the latter scenario, however, there’s a more complex challenge in play. Large-scale file sharing of any kind, including ZIP file sharing, instantly makes manual file processing less efficient. On top of that, the specific organization of those files begins to play a bigger role. Broad ZIP archives often contain internal directories and subdirectories which are organized based on hierarchies relevant to the person who sent that information, and not necessarily relevant to the recipient. If, for example, a large ZIP archive is shared containing directories relevant to multiple separate stakeholders with separate storage access permissions, the process of sending each file to its new home will become a burden. The files will need to be extracted, diverted, and stored (or re-zipped in a new archive), and that could take a considerable amount of time given the volume of compressed files in play.
Thankfully, given the simple directorial hierarchies at play within ZIP archives, API automation is a great solution for processing them in large volumes. Programmatically opening, extracting, and diverting the contents of large ZIP files is quite straightforward: once ZIP files are processed and their contents are exposed via API response, developers can create scripts to customize where specific subdirectories or file encodings should be sent. These unzipped contents can rapidly reach the correct destination and be manually (or programmatically) re-zipped in a new archive that is more relevant to its recipient.
Demonstration
In this article, I’ll highlight two APIs that can be used to programmatically extract the contents of a ZIP archive. Both APIs can be used for free (up to 800 API calls per month) with a free-tier Cloudmersive API key, and they can be easily implemented using the ready-to-run Java code examples provided further down the page.
The first of these two APIs is designed to extract and decompress a regular ZIP archive (one which does not have active encryption or password protection). This API’s response will return all relevant directories and documents contained within an input ZIP file relative to their directorial hierarchies, starting with any “loose leaf” files which are not attached to a specific directory. The name of each file will be returned alongside a string containing the encoding for that file, making it easy to pass all of the above content to your desired storage location. Below, I’ve included an example JSON response model to illustrate the way this information is presented:
{
"Successful": true,
"FilesInZip": [
{
"FileName": "string",
"FileContents": "string"
}
],
"DirectoriesInZip": [
{
"DirectoryName": "string",
"DirectoriesInDirectory": [
null
],
"FilesInDirectory": [
{
"FileName": "string",
"FileContents": "string"
}
]
}
]
}
The second API can be used to decrypt and remove password protection from those ZIP files with active security measures in place. Only the input file and a valid password are required to call this API, and the response body will contain the decrypted ZIP file encoding with password protection removed. This file can be subsequently processed by the previously described API for extraction and decompression.
To begin structuring your API calls, your first step is to install the Java SDK. To install with Maven, first, add the following reference to the repository in pom.xml:
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
After that, add a reference to the dependency in pom.xml:
<dependencies>
<dependency>
<groupId>com.github.Cloudmersive</groupId>
<artifactId>Cloudmersive.APIClient.Java</artifactId>
<version>v4.25</version>
</dependency>
</dependencies>
To install with Gradle, add it to your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Then, add the dependency in build.gradle:
dependencies {
implementation 'com.github.Cloudmersive:Cloudmersive.APIClient.Java:v4.25'
}
With installation out of the way, your next step is to structure each API request. To call the “Extract, Decompress Folders from a ZIP Archive” iteration, copy and paste from the code examples provided below:
// Import classes:
//import com.cloudmersive.client.invoker.ApiClient;
//import com.cloudmersive.client.invoker.ApiException;
//import com.cloudmersive.client.invoker.Configuration;
//import com.cloudmersive.client.invoker.auth.*;
//import com.cloudmersive.client.ZipArchiveApi;
ApiClient defaultClient = Configuration.getDefaultApiClient();
// Configure API key authorization: Apikey
ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey");
Apikey.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Apikey.setApiKeyPrefix("Token");
ZipArchiveApi apiInstance = new ZipArchiveApi();
File inputFile = new File("/path/to/inputfile"); // File | Input file to perform the operation on.
try {
ZipExtractResponse result = apiInstance.zipArchiveZipExtract(inputFile);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ZipArchiveApi#zipArchiveZipExtract");
e.printStackTrace();
}
To call the “Decrypt and Remove Password Protection” iteration, copy and paste from the following code examples:
// Import classes:
//import com.cloudmersive.client.invoker.ApiClient;
//import com.cloudmersive.client.invoker.ApiException;
//import com.cloudmersive.client.invoker.Configuration;
//import com.cloudmersive.client.invoker.auth.*;
//import com.cloudmersive.client.ZipArchiveApi;
ApiClient defaultClient = Configuration.getDefaultApiClient();
// Configure API key authorization: Apikey
ApiKeyAuth Apikey = (ApiKeyAuth) defaultClient.getAuthentication("Apikey");
Apikey.setApiKey("YOUR API KEY");
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//Apikey.setApiKeyPrefix("Token");
ZipArchiveApi apiInstance = new ZipArchiveApi();
File inputFile = new File("/path/to/inputfile"); // File | Input file to perform the operation on.
String zipPassword = "zipPassword_example"; // String | Required; Password for the input archive
try {
Object result = apiInstance.zipArchiveZipDecrypt(inputFile, zipPassword);
System.out.println(result);
} catch (ApiException e) {
System.err.println("Exception when calling ZipArchiveApi#zipArchiveZipDecrypt");
e.printStackTrace();
}
That’s all the code you’ll need to complete both API calls. It’s important to note that you should only use these APIs to unzip thoroughly vetted files from sources that you trust, as ZIP files are frequently used in cyber-attacks to deliver malware or exfiltrate data from a system.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK