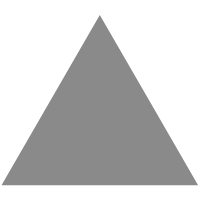
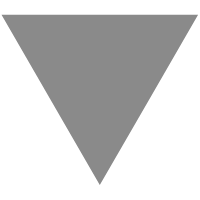
Using Alfred to Quickly Open Workspaces
source link: https://dzone.com/articles/using-alfred-to-quickly-open-workspaces
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
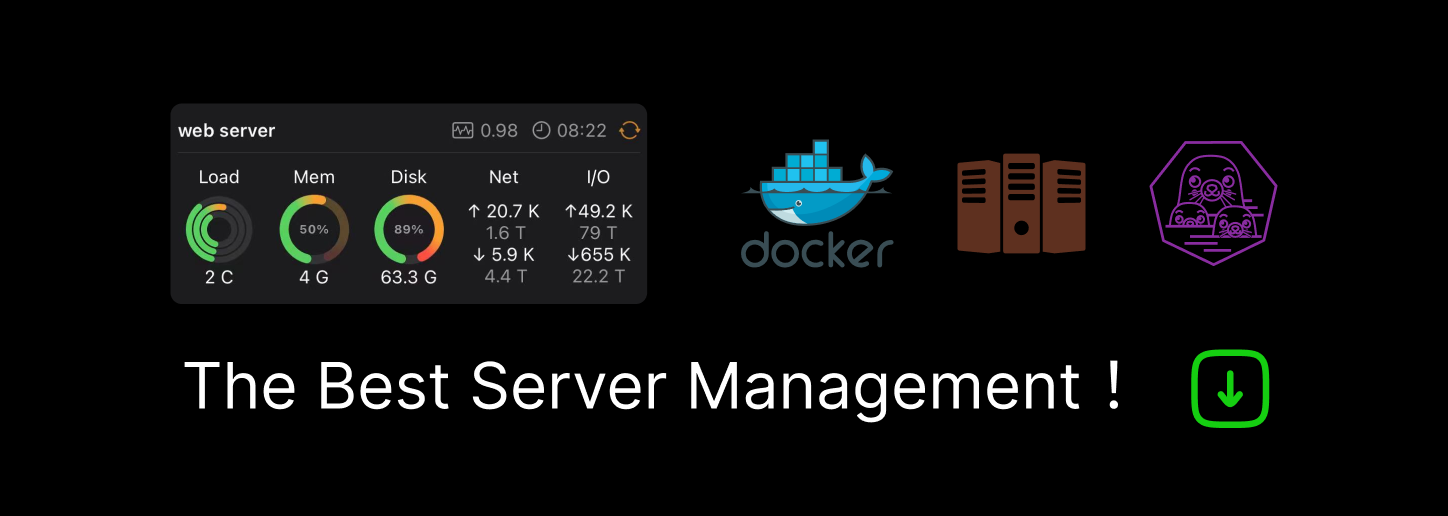
I use VSCode for almost all of my development, enabling me to save folders and their custom settings to workspace files.
Alfred is a macOS program that serves as a launcher, clipboard manager, finder, and much more. In this case, I want to use Alfred as a way to quickly and interactively choose a VSCode workspace to open.
Required Structure
Alfred’s Script Filters allow us to search a dynamic list for some item and then do something with that item. The documentation then links to the Script Filter JSON format. We’re going to create a script (binary) that outputs all discovered workspaces in the required format.
We can use basic commands to discover all workspaces we want to include:
find ~/code -type file -maxdepth 1 -name '*.code-workspace'
This gives us all workspace files in the ~/code directory. From there, we can easily create the JSON object required by Alfred.
The equivalent command from go is:
filepath.Glob(fmt.Sprintf("%s/*%s", filepath.Join(home, codeDir), workspaceFileType))
Where home
is our home directory, and codeDir
is the folder in our home directory that contains our workspaces. Now that I’ve found all our workspace files, I just need to create JSON objects from them that Alfred will consume.
We’ll define a basic struct to represent that object:
type alfredList struct {
Items []alfredItem `json:"items"` // All the items to choose from
}
type alfredItem struct {
UID string `json:"uid"` // ID, just the basename
Type string `json:"type"` // Type of item, always 'file'
Title string `json:"title"` // Title to display - friendly name
Subtitle string `json:"subtitle"` // Subtitle to display - short filepath
File string `json:"arg"` // Filepath to open if the item is selected
Autocomplete string `json:"autocomplete"` // String to use for autocomplete
}
We can definitely get fancier with our objects, but for now, we’ll keep it simple. Our function will create an alfredItem given the file path to a workspace file:
// newAlfredItem creates an alfredItem given the path of a workspace file
func newAlfredItem(workspaceFile string) alfredItem {
// Remove leading path and file extension
baseName := strings.Replace(filepath.Base(workspaceFile), workspaceFileType, "", 1)
return alfredItem{
UID: baseName,
Type: "file",
Title: baseName,
Subtitle: filepath.Join("~", codeDir, baseName), // Use '~' instead of $HOME
File: workspaceFile,
Autocomplete: strings.ReplaceAll(baseName, "-", " ") // Split dash-separated words
}
}
So we want to feed all discovered workflow files into our newAlfredItem
method and then print those in the required format. With basic error handling, we end up with this:
matches, err := filepath.Glob(fmt.Sprintf("%s/*%s", filepath.Join(home, codeDir), workspaceFileType))
if err != nil {
log.Fatal(err)
}
list := alfredList{}
for _, m := range matches {
list.Items = append(list.Items, newAlfredItem(m))
}
output, err := json.Marshal(list)
if err != nil {
log.Fatal(err)
}
fmt.Println(string(output))
Using From Alfred
Create a new blank workflow. In the new workflow, add a Script Filter by selecting Inputs -> Script Filter. We need to give it some keywords that will bring up the list when we type it. I used ‘vc’ because nothing else was using it.
To get the result of our program into the list of items, we’ll just compile our Go program and run the binary from our workflow.
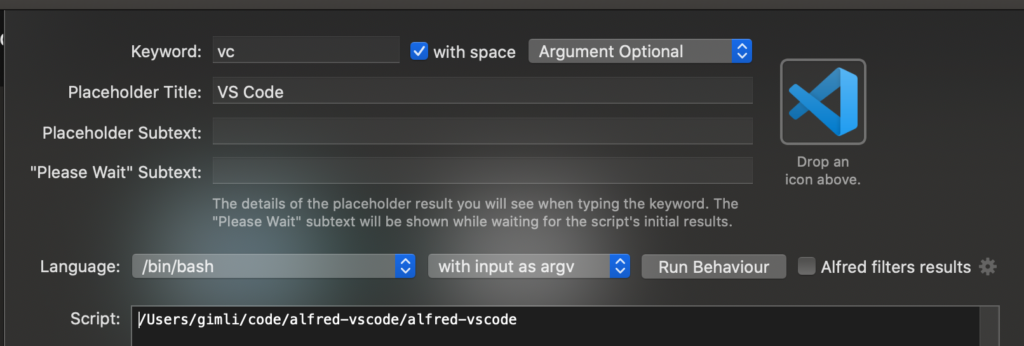
When we run the workflow by entering the keyword vc, we see a list of the discovered workspaces:
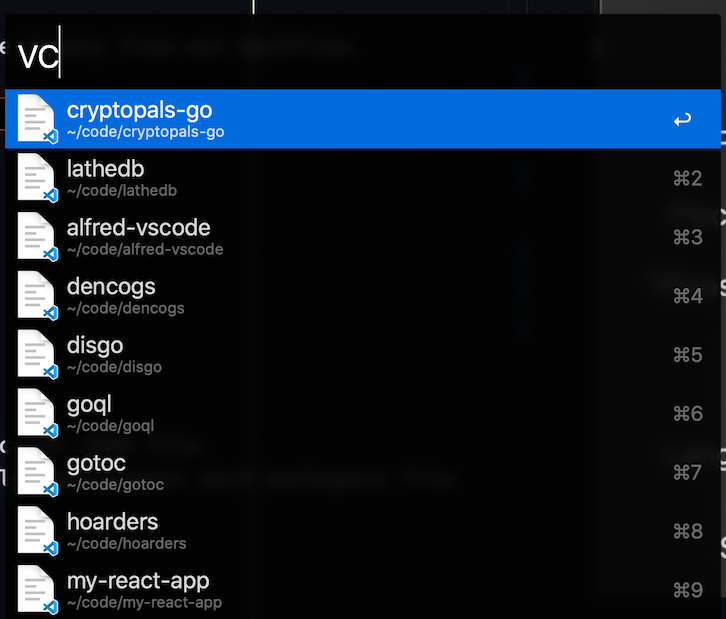
By hitting Enter on a single workspace, we’ll open it in VS Code.
Improvements
Unless we’re adding a lot of workspaces each day, we’ll be fine getting our list from a static-ish JSON file. In the past, I’ve used Lingon to create a job that periodically rediscovers each workspace file and saves them to a JSON file.
Recommend
-
89
Alfred-Youdao Youdao Dict for Alfred.
-
19
Visual Studio Code project manager using Alfred workflowPublished: 2021.03.26 | 1 minutes readI spend a lot of time using Visual Studio Code, jumping from one project to another. To make it more efficient, I would love to have...
-
2
Using a custom devfile registry and C++ with Red Hat CodeReady Workspaces
-
5
Using Kubernetes Workspaces with Code StreamOne feature that has been consistently requested by customers using Code Stream is the ability to use Kubernetes instead of a stand-alone Docker host to execute CI tasks in Pipelines. Well, today I’...
-
3
Alfred 4 Workflow Open in VSCode Feature
-
6
Citrix exec explains why orgs are using DaaS to secure hybrid workspaces
-
5
AppleScript for using Kitty in Alfred. This was made for bash, but can easily be made to work with any shell.
-
10
AI AlfredSummarize articles in 1 click using the AIFree OptionsAI Alfred is your best friend for summaries. It can summarize whatever you want in...
-
3
BlogUsing environment based Terraform Workspaces for development with Terraform Cloud Andrew Stump 23 Aug, 2023
-
2
Getting a Bundle ID using an Alfred File Action
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK