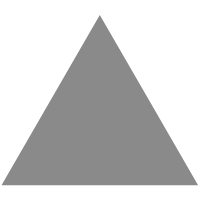
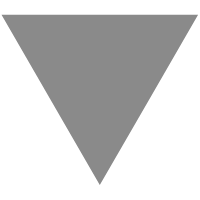
Mathematically Inclined Java
source link: https://devm.io/java/java-math-libraries
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
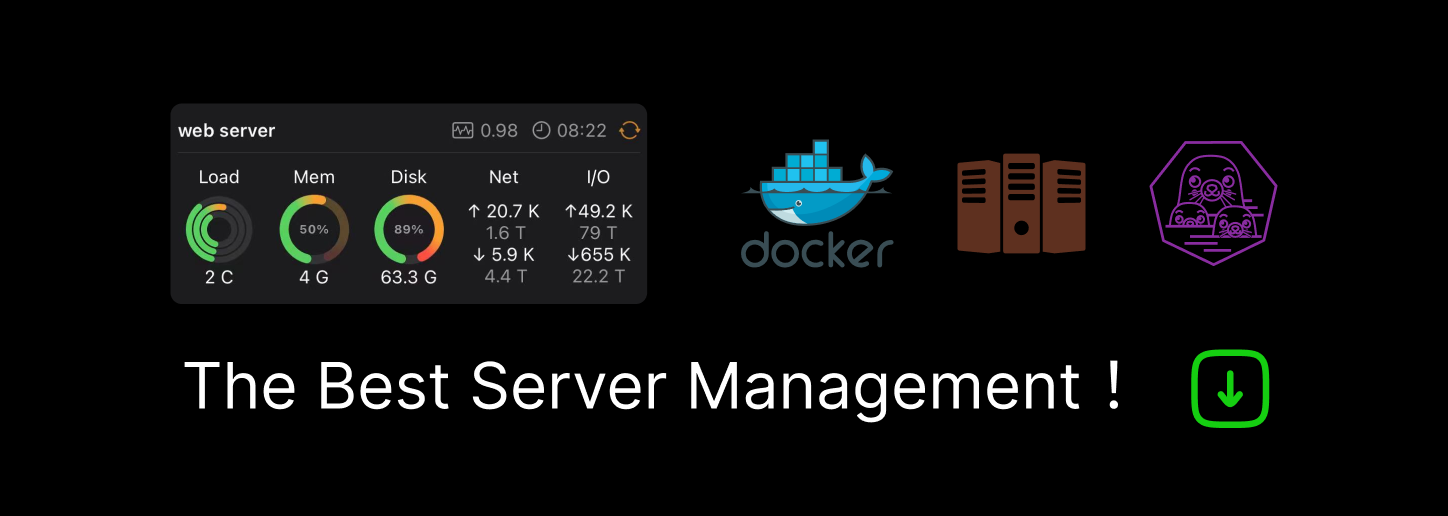
Granted, higher math is complex and time-consuming to learn. Selected Java libraries save programmers from having to deal with higher math.
This century, if not the whole millennium, will be characterized by data becoming the new oil. As a result, the number of technology-related tasks will increase, pleasing data scientists and IT specialists, but it may require (very) good knowledge of mathematics. Meanwhile, higher mathematics with all of its proofs, derivatives, integrals, matrices, and differential equations requires more time until the theory works. Implementing the whole thing in Java so that it works properly is yet another thing in itself. For example, the result of an integral or a derivative determined with a Java program might not be as accurate as a calculator. Whereas, existing math libraries have already been tested for accuracy.
User input is usually processed first as a string in command line tools. This is where Expr can be useful. Expr can parse literal numbers, variables, arithmetic and relational operators, elementary functions, and trigonometric functions. Another use of Expr could be a graphical program that draws function graphs. This is because Expr can parse and calculate a function’s value at a given point.
Expr can’t be obtained from a Java repository, instead the previously downloaded source files must be compiled. The resulting .jar file can then be included in the project. With Linux, first unpack the ZIP file and generate the .jar file as follows:
$ cd expr-master
$ make
Suppose you write a command line tool which outputs the x and y components of the velocity vector v, specifying the velocity and the direction theta. Here, one function is required for each vector component. The functions are defined as strings in Expr:
String v_x = "v * cos(theta)";
String v_y = "v * sin(theta)";
As is typical with Java, you retrieve the arguments, which represent the magnitude of the velocity and direction, using the Main method:
double v = Double.valueOf(args[0]).doubleValue();
double theta = Double.valueOf(args[1]).doubleValue();
Expr recognizes variables occurring in the function. In order to further process the variables contained there, specially defined variables of the type Variable are required, which accommodate the variable name occuring in the function. Optionally, you can specify which variables a value can be assigned to (Listing 1). In the specially defined function setFunction(), the string is parsed with the function it contains and, if needed, catches the exception. If you use the explain() method in the Catch block, you can give users an easy to understand error message with hints. In our example, Expr returns an error message if the function is defined as follows:
String v_x = "v(cos(theta))";
I got as far as "v" and then saw "(".
The first part makes sense, but I don't see how the rest connects to it.
An example of a formula I can parse is "v*(cos(theta))".
Developers can spare having a user-friendly error message, as Expr already takes care of it. The calcVelocityComponent method from Listing 1 is given the values for velocity and the direction variable, which is used to calculate components of the velocity vector. The advantage is that variables’ values are not strings, preserving type safety and code readability.
Listing 1
import expr.Expr;
import expr.Parser;
import expr.SyntaxException;
import expr.Variable;
public class MyExpr {
private Expr expr;
private Variable v;
private Variable theta;
private Parser parse;
public MyExpr(String func, double v, double t) {
this.v = Variable.make("v");
this.theta = Variable.make("theta");
this.parse = new Parser();
this.parse.allow(this.v);
this.parse.allow(this.theta);
setFunction(func);
calcVelocityComponent(v,t);
}
private void setFunction(String f) {
try {
this.expr = this.parse.parseString(f);
} catch (SyntaxException e) {
System.err.println(e.explain());
}
}
private void calcVelocityComponent(double val,double t) {
this.v.setValue(val);
this.theta.setValue(t);
System.out.println(this.expr.value());
}
}
For example, this way a function’s value range can be determined by using a for loop which calculates the y-values in a given interval.
Polynom
The Java library Polynom is all about polynomials. It provides methods and classes that allow operations on the polynomial. The Java library was last updated in 2017, but it’s a favorite because it greatly simplifies working with polynomials.
Theoretically, there are four different ways of creating a polynomial with the eponymous Java library, but only three worked in our test. Listing 2 shows how a polynomial can be defined in Java. The listing also includes other methods, like the readable output of a polynomial (cf. show()).
Listing 2
import com.polynom.polynom.Polynom;
public class MyPolynom {
private Polynom polynom;
// first option
public MyPolynom() {
this.polynom = new Polynom();
}
// second option
public MyPolynom(int a, int deg ) {
this.polynom = new Polynom(a,deg);
}
// third option
public MyPolynom(double[] iCoeff ) {
this.polynom = new Polynom(iCoeff);
}
// fourth option
public MyPolynom(String s ) {
this.polynom = new Polynom(s);
}
public void show() {
System.out.println(this.polynom);
}
public static void show(Polynom p) {
System.out.println(p);
}
public void showCoefficients() {
double[] coeffs = this.polynom.getCoeffs();
System.out.println("Coefficients:" + Arrays.toString(coeffs));
}
public static void printResults(double[] dArr ) {
System.out.println("Result: "+Arrays.toString(dArr));
}
public static void printResults(double d ) {
System.out.println("Result: "+d);
}
public Polynom getPolynom() {
return this.polynom;
}
public void printDegree() {
int n = this.polynom.degree();
System.out.println("Degree: "+n);
}
public Double calcVar(double dVal) {
double y = this.polynom.valueOf(dVal);
return y;
}
public double[] calcVar(double[] dVals) {
double[] ys = this.polynom.valueOf(dVals);
return ys;
}
}
If no arguments are given to the constructor of the type MyPolynom, then the polynomial x^0 is created. When evaluated, this always gives the value y = 1. You can also define a one-digit polynomial with the type a^{degree} with only two arguments consisting of the coefficient and the degree. If the polynomial contains several coefficients, immediately pass a list with all of the coefficients to the constructor, even if one or more coefficients are equal to the value 0. First, start with the constant, followed by the next higher coefficient—in this case, x^1—and so on. Therefore, a fourth degree polynomial needs 4 + 1 coefficients, i.e. |N|+1. Unfortunately, the most intuitive way of passing a string as an argument to the constructor of the type Polynom didn’t work in our test. The Java library throws an exception instead. Although, the same result can be achieved with a coefficient list.
Apart from polynomial definitions, the Java library has several operations that can apply to polynomials. For example, two polynomials can be added, subtracted, and multiplied. The polynomial can also be embedded in a power function: ({Polynom})^n. You can also change the sign and output the derivative. Listing 3 shows how the constructors defined in Listing 2 are called and operations are performed on the polynomial.
Listing 3
// x^0
MyPolynom p1 = new MyPolynom();
p1.show();
//creates a polynomial consisting of a coefficient
int a = 2;
int aDegree = 3;
MyPolynom p2 = new MyPolynom(2,3);
p2.show();
// Fourth degree polynomial
double[] dCoefficients = {2, -1, -6, 1, 1};
MyPolynom p3 = new MyPolynom(dCoefficients);
p3.show();
p3.printDegree();
Polynom p13 = p3.getPolynom().differentiate();
MyPolynom.show(p13);
double[] roots = p3.getPolynom().solve();
MyPolynom.printResults(roots);
double [] xs = {0,2,4,6,8,10};
double [] ys = p3.calcVar(xs);
MyPolynom.printResults(ys);
// Quadratic equation
double[] dCoefficients4 = {-15, 10, 5};
MyPolynom p4 = new MyPolynom(dCoefficients4);
p4.show();
double[] roots4 = p4.getPolynom().solve();
MyPolynom.printResults(roots4);
double y4 = p4.calcVar(2);
MyPolynom.printResults(y4);
As the examples in Listing 3 show, the derivation is done in the blink of an eye. All that’s needed is a call to the predefined method differentiate(). Zeros no longer require eternal fiddling for ages. Instead, the method solve() calculates zeros. The specially defined method calcVar() outputs a single Y-value. Additionally, the calcVar() method can accept an entire definition range in the form of a list, and determine its associated value range. A readable polynomial outside is controlled by the Java library, so a simple Print is sufficient (Fig. 1).
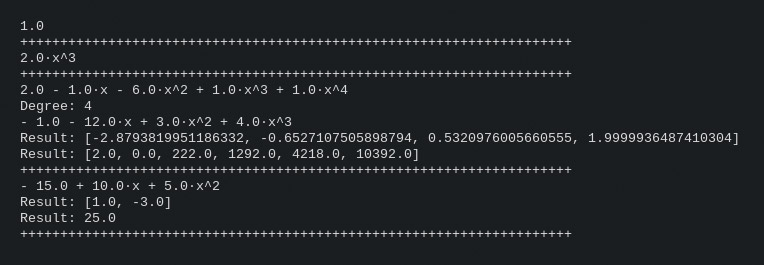
Fig. 1: Readable output of polynomials
Further examples can be found in the file Main.java, located in the downloaded ZIP file in the path ~/polynom-master/src/com/polynom/main/.
finmath lib
Business mathematics is a popular subject at LMU and it’s relevant in finance and insurance industries as well. As digitization advances in these industries, there’s a need for algorithms and methods that are interesting for mathematical finance. The Java library finmath lib provides useful classes for financial mathematics that can apply to other fields as well.
You can install the finmath lib by downloading the required .jar files from the [Maven repository]9https://mvnrepository.com/artifact/net.finmath/finmath-lib/5.1.3). To show calculation results as a graph, you also need the plot extensions. Other Java libraries are needed too: Apache Commons Math, Apache Commons Lang, JFreeChart, and ItextPDF.
One of finmath lib’s special topics is a Monte Carlo simulation, whose methods determine statistical properties during a simulation. ...
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK