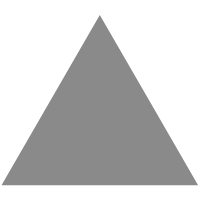
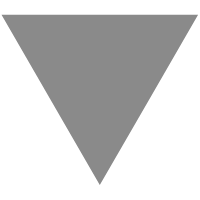
Google reCAPTCHA V3 Tutorial with Example in PHP
source link: https://www.laravelcode.com/post/google-recaptcha-v3-tutorial-with-example-in-php
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
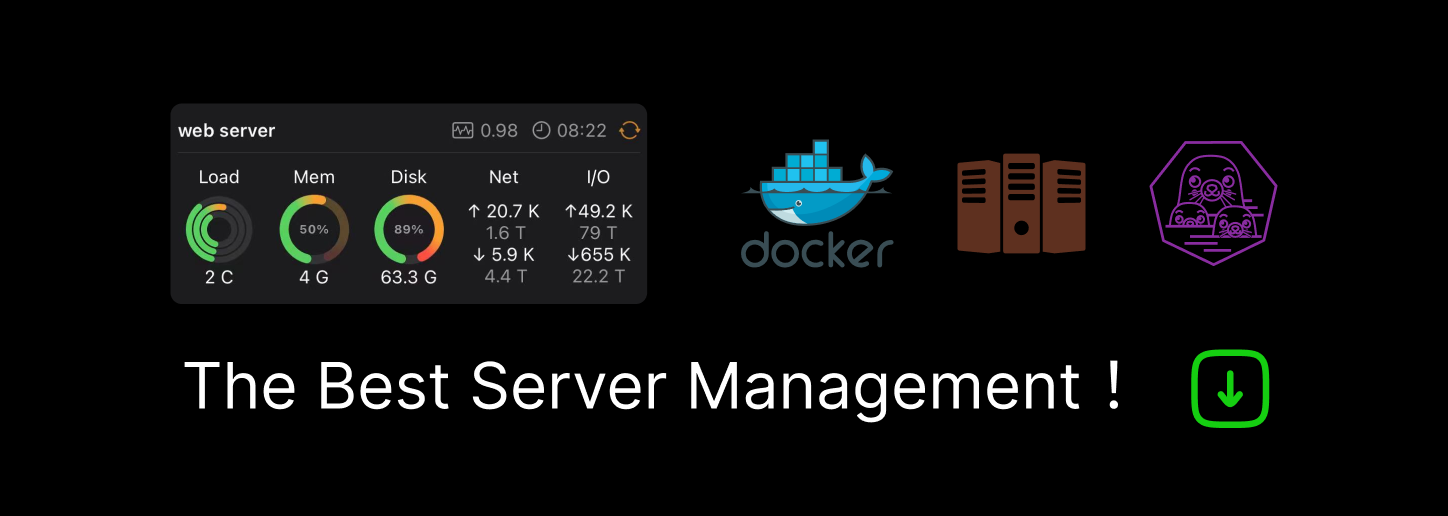
Google reCAPTCHA V3 Tutorial with Example in PHP
Google reCAPTCHA is tool by Google which protects form from brute force attack, spams and other types of automated tools. There are currently reCAPTCHA V3 and reCAPTCHA v2("I'm not a robot" Checkbox) and reCAPTCHA v2 (Invisible reCAPTCHA badge) available.
In this article, we will latest Google reCAPTCHA Version3 in a website form without using any package. We will verify token using PHP server-side processing language. Though you can verify using any server-side language with CURL request.
To use reCAPTCHA, first register and create admin console. In the register form, input Label name, reCAPTCHA type you want to use, Domain name, your email address and accept Terms and Conditions. You will get Site Key and Secret Key, that you should copy and keep somewhere safe.
There are two ways, you can setup reCAPTCHA on your website. We will use manually generate token and verify using PHP server language. For Javascript event handling, we will use JQuery library for easiness.
First of all, create the form you want to protect from spam.
<form id="login-form">
<input type="email" name="email" required>
<input type="password" name="password" required>
<input type="hidden" name="googlerecaptcha" id="googlerecaptcha" required>
<button type="submit" id="submit-contact-form">Login</button>
</form>
Load the JavaScript API with render parameter of your sitekey.
<script src="https://www.google.com/recaptcha/api.js?render=reCAPTCHA_site_key"></script>
Call grecaptcha.execute when you want to generate token. We will execute this on submit button click.
<script type="text/javascript">
$(document).ready(function() {
$(document).on('click', '#submit-contact-form', function (e) {
e.preventDefault();
grecaptcha.ready(function() {
grecaptcha.execute('reCAPTCHA_site_key', {action: 'submit'}).then(function(token) {
$('#googlerecaptcha').val(token);
$('#contact-form').submit();
});
});
});
});
</script>
Here is full code of your HTML form. We will use JQuery library to handle event easily.
<!DOCTYPE html>
<html>
<head>
<title>Google reCAPTCHA V3</title>
</head>
<body>
<form type="post" id="login-form" action="/submit.php">
<input type="email" name="email" required>
<input type="password" name="password" required>
<input type="hidden" name="googlerecaptcha" id="googlerecaptcha" required>
<button type="submit" id="submit-contact-form">Login</button>
</form>
<script src="https://www.google.com/recaptcha/api.js?render=reCAPTCHA_site_key"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function() {
$(document).on('click', '#submit-contact-form', function (e) {
e.preventDefault();
grecaptcha.ready(function() {
grecaptcha.execute('reCAPTCHA_site_key', {action: 'submit'}).then(function(token) {
$('#googlerecaptcha').val(token);
$('#contact-form').submit();
});
});
});
});
</script>
</body>
</html>
From the above code, when user click submit the form, Google reCAPTCHA will verify that form submission was done by genuine user and create the token. We will send the token to server side and verify it.
Note: The token is only valid for 2 minutes only. So you have to verify it within 2 minutes only. Token once verify, will be expired immediately and can't be used to verify again.
For server side verify, first create submit.php file where form will be submitted.
<?php
$captcha = real_escape_string($_POST['googlerecaptcha']);
$request_url = 'https://www.google.com/recaptcha/api/siteverify';
$request_data = [
'secret' => 'reCAPTCHA_secret_key',
'response' => $captcha
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $request_url);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $request_data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response_body = curl_exec($ch);
curl_close ($ch);
$response_data = json_decode($response_body, true);
if ($response_data['success'] == false) {
// return back with error that captcha is invalid
} else {
// captcha is valid and proceed for next
}
This way you can implement Google reCAPTCHA in your website. Not you can get statestic link at Google admin console.

I hope it will help you.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK