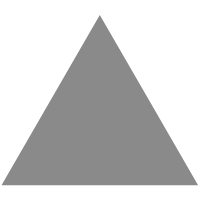
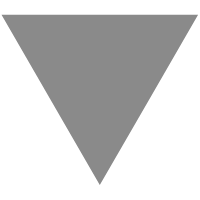
ChatGPTs Singleton Implementation
source link: https://blogs.u2u.be/peter/post/chatgpts-singleton-implementation
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
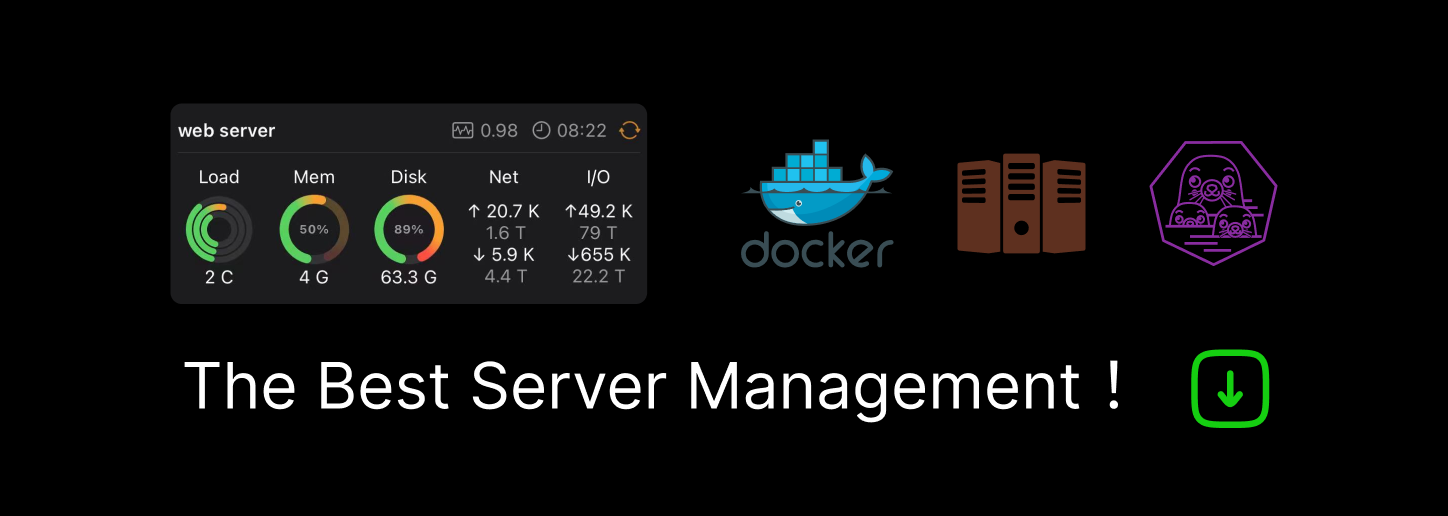
ChatGPTs Singleton Implementation
I asked ChatGPT to write me some code:

This was the answer I got:
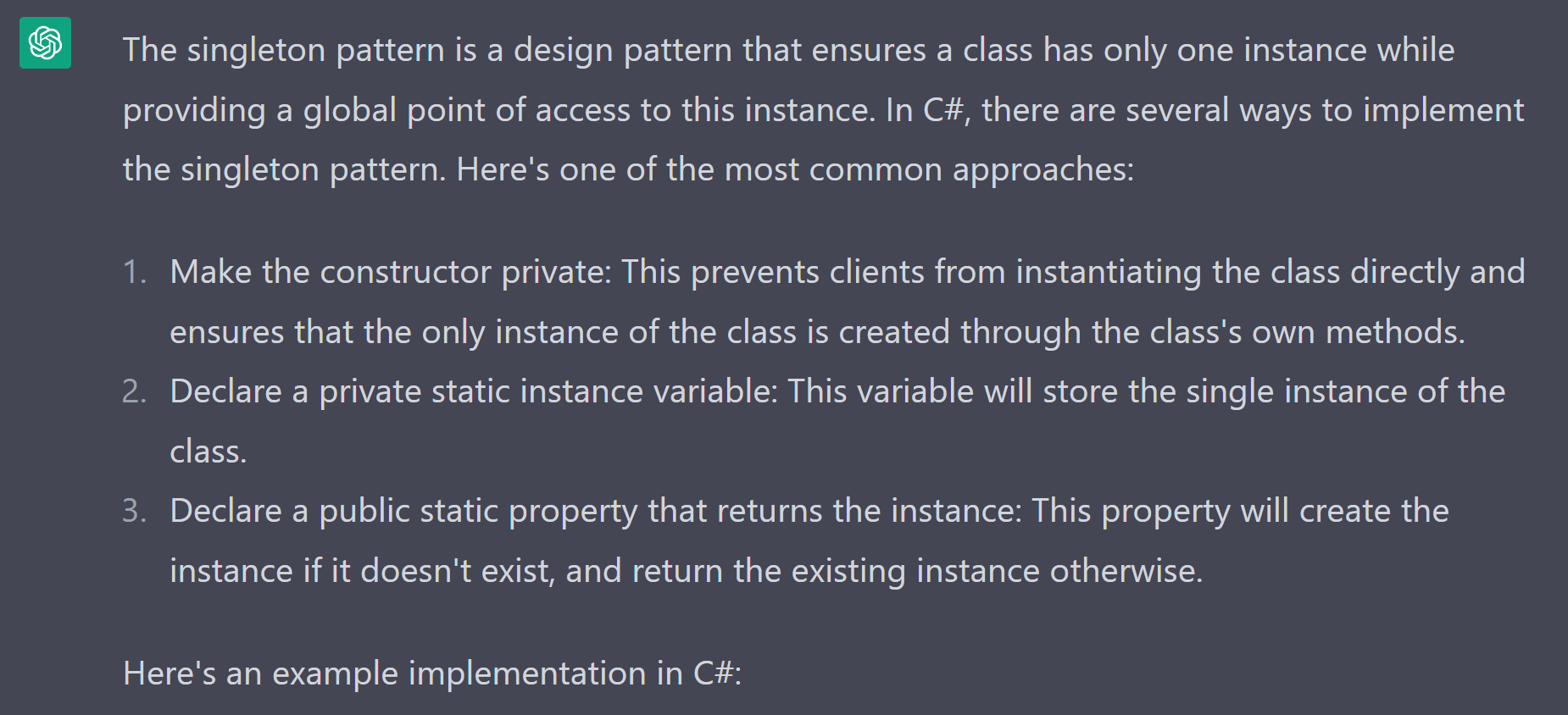
Including this code fragment
public sealed class Singleton
{
private static Singleton instance = null;
private static readonly object padlock = new object();
private Singleton() {}
public static Singleton Instance
{
get
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
}
Nice piece of code, taking care of lazy instantiation, and thread-safe.
But I don't think ChatGPT is going to replace a well-trained developer yet. Even in this code there are optimizations, like checking for null
before taking the lock:
get
{
if (instance == null)
{
lock (padlock)
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
But what I am really missing is the .NET way of implementing singleton: Let the runtime take care of this by this simple piece of code:
public sealed class Singleton
{
private Singleton() { }
private static Singleton Instance { get; } = new Singleton();
}
Here the static constructor will take care of lazy instantiation in a thread-safe way.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK