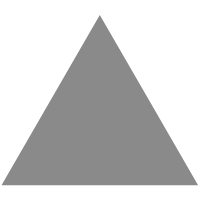
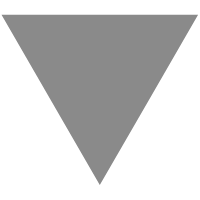
Environment Variables in JavaScript: process.env
source link: https://dmitripavlutin.com/environment-variables-javascript/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
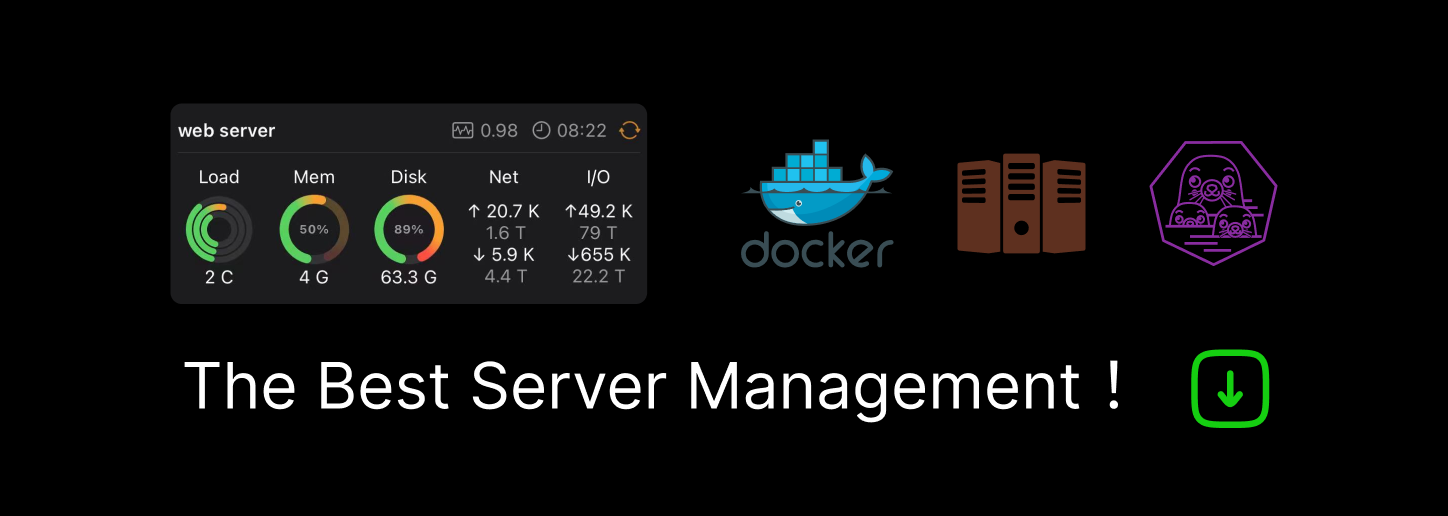
Environment Variables in JavaScript: process.env
The environment variables are defined outside of the JavaScript execution context.
There's a set of environment variables defined by the OS, for example:
USER
: the current userHOME
: the current user's home pathPWD
: the current working directoryPATH
: directories to search to execute a command
In regards to JavaScript and Node.js ecosystem, you might find the following variables common:
NODE_ENV
: determines if the script runs in development or production mode. Usually takes one of the values:production
,prod
,development
,dev
, ortest
PORT
: the port which the running application should be working with (e.g.3000
,8080
, etc.)
Let's see how you can access these environment variables (either OS or Node.js specific) in a JavaScript file.
1. process.env object
When executing a JavaScript file as a Node CLI (command line interface) command, Node creates a special object process.env
which contains the environment variables as properties.
For example, let's execute the JavaScript file /home/dmitri/main.js
in the command line:
NODE_ENV=production node main.js
Where main.js
file contains the following code:
// /home/dmitri/main.js
console.log(process.env.USER); // dmitri
console.log(process.env.PWD); // /home/dmitri/
console.log(process.env.NODE_ENV); // production
process.env.USER
is the operating system user name that executes the command. The variable has a value of 'dmitri'
because this is my OS user name.
process.env.PWD
contains the absolute path to the directory where the executed file is located. Since the executed file path is /home/dmitri/main.js
, then the current working directory path is '/home/dmitri/'
.
The above variables are taken from the environment of the operating system.
process.env.NODE_ENV
variable equals 'production'
. This variable, contrary to the 2 above, is defined by the prefix NODE_ENV=production
of the command NODE_ENV=production node main.js
.
If you'd like to provide local defaults to certain environment variables, then check the dotenv project.
2. process.env in a browser environment
The environment variables, including process.env
, are accessible to scripts running in the CLI.
process.env
, however, is not available in a browser environment. The browser doesn't define process.env
.
Fortunately, exposing environment variables to the runtime in the browser can be done using bundlers. Let's see how it's done using Vite and webpack.
2.1 Vite
Vite exposes a predefined set of variables through import.meta.env
object:
import.meta.env.MODE
: is either'development'
or'production'
import.meta.env.PROD
: istrue
in production modeimport.meta.env.DEV
: istrue
in development modeimport.meta.env.SSR
: is a boolean whether the app runs on the server sideimport.meta.env.BASE_URL
: the base URL
On top of that, Vite can also load variables from .env
file. Under the hood, Vite uses dotenv. But you don't have to manually call anything related to dotenv: Vite does everything for you.
For example, having an .env
file like this:
VITE_MY_VAR=value
then you can access this value in the browser during runtime import.meta.env.VITE_MY_VAR
, which is going to be 'value'
.
Please note that Vite exposes publicly only variables starting with VITE_
prefix.
Open the demo and see that the variables provided by Vite are rendered on the webpage.
Vite has a detailed guide on how to access the environment variables.
2.2 webpack
The built-in plugin EnvironmentPlugin exposes environment variables in webpack.
For example, to expose the NODE_ENV
env variable, you can use the following configuration:
// webpack.config.js
const { EnvironmentPlugin } = require('webpack');
module.exports = {
// ...
plugins: [
// ...
Open the demo. NODE_ENV
variable was exposed by webpack and its value is rendered on the webpage.
If NODE_ENV
variable is not available in the environment, the plugin will throw an error. But you can assign a default value to a variable using a plain JavaScript object (with the value being the default value):
// webpack.config.js
const { EnvironmentPlugin } = require('webpack');
module.exports = {
// ...
plugins: [
// ...
new EnvironmentPlugin({
With the above configuration, if NODE_ENV
variable isn't set up, webpack defaults process.env.NODE_ENV
to development
.
3. Conclusion
A JavaScript file executed in Node CLI can access the environment variables using the special object process.env
.
For example, process.env.USER
contains the user name that executes the script.
The environment variables are not available during runtime in a browser. But modern bundlers like Vite and webpack can expose certain variables.
For example, Vite exposes the current running mode of the application using import.meta.env.MODE
. In webpack EnvironmentPlugin
lets you expose the necessary variables.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK