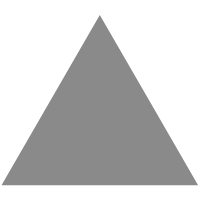
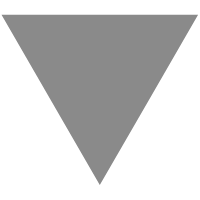
Authenticate Users Via Face Recognition On Your Website or App
source link: https://hackernoon.com/authenticate-users-via-face-recognition-on-your-website-or-app
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
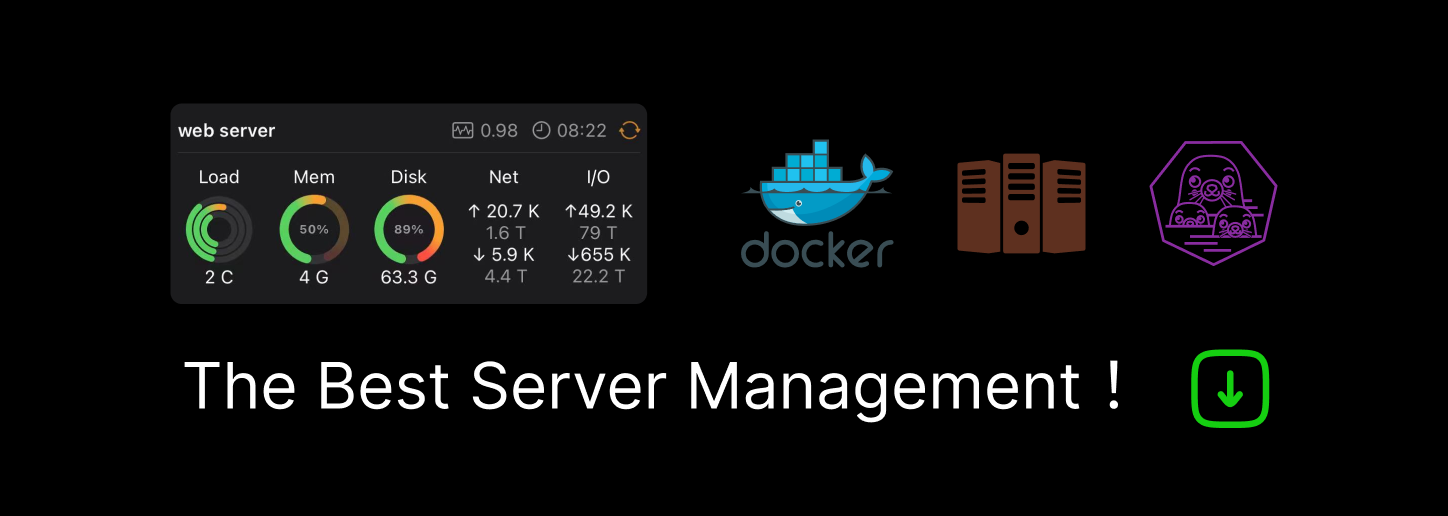
Authenticate Users Via Face Recognition On Your Website or App
Too Long; Didn't Read
In this tutorial, we will be building a simple yet smart web application, to demonstrate how to authenticate users on a typical website or web application via face recognition instead of the traditional login/password pair.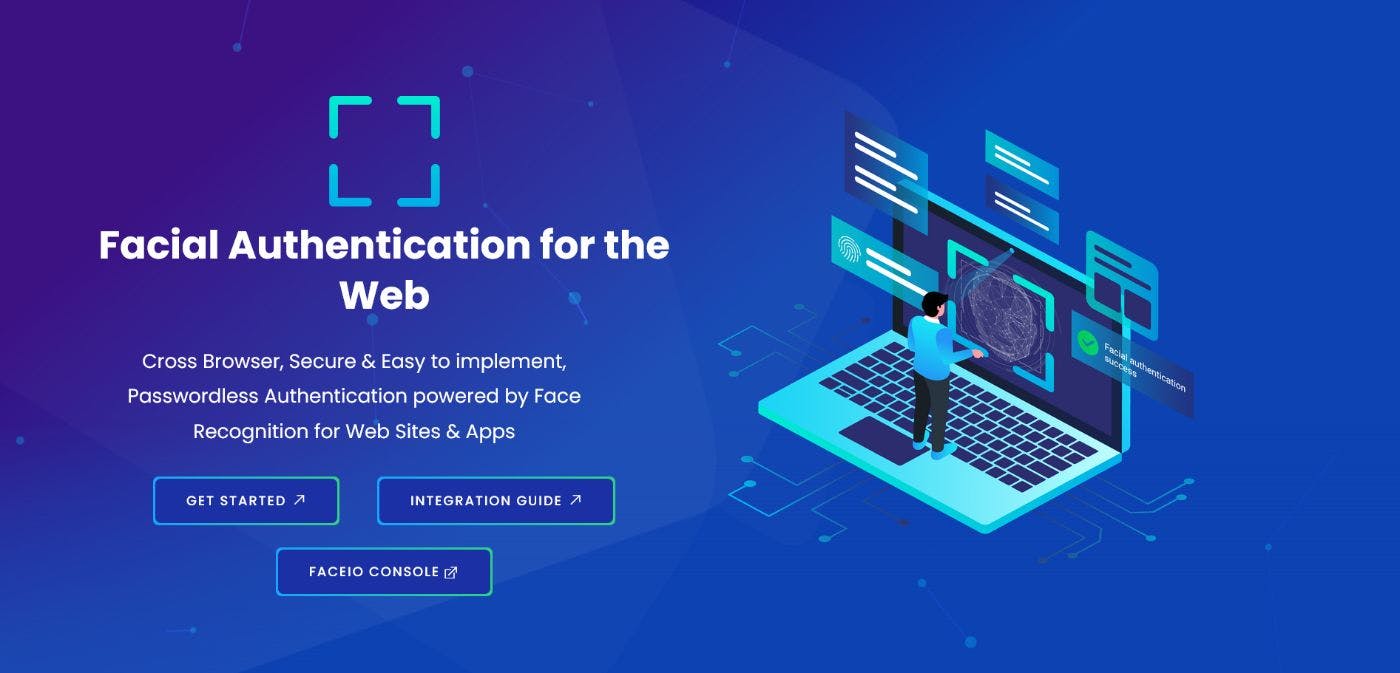
audio
element.In this tutorial, we will be building a simple yet smart web application, to demonstrate how to authenticate users on a typical website or web application via face recognition instead of the traditional login/password pair.
In this process, we are going to rely onFaceIOas the facial recognition library, React.js as the JavaScript framework, and TailwindCss as the CSS framework.
The final version of this project looks like this. Without further ado, let's get started.
Overview
Please bear in-mind is that while this tutorial is focused on integrating FaceIO with React, the fio.js, facial recognition library can be integrated with any modern JavaScript framework out here including but not limited to React, Vue, Angular, Next or even Vanilla JavaScript. The reader is invited to take a look at the FaceIO integration guide or getting started documentation for a step-by-step integration guide.
Creating a Rect.js project with tailwind CSS
We are using vite to create the ReactJs project in this tutorial. Open
your terminal and run the following command to scaffold your React
project using vite.
npm create vite@latest my-project — — template react
Now follow the terminal prompt to scaffold your project. After you
completed this step, open this project in your favorite code editor. The
project structure should look like this.
├── index.html
├── package.json
├── package-lock.json
├── public
│ └── vite.svg
├── src
│ ├── App.css
│ ├── App.jsx
│ ├── assets
│ │ └── react.svg
│ └── main.jsx
└── vite.config.js
Now, to install tailwind CSS in your vite project, follow these steps carefully.
npm install -D tailwindcss postcss autoprefixer
Now open your
tailwind.config.js
file and add your project source path. /** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Now you have successfully installed tailwind CSS in your React and vite project. Let’s focus on how to integrate face authentication in our project in the next section all powered by FaceIO.
Adding FaceIO to Your React application
The great thing about the fio.js facial recognition library is its straightforward usage. This library comes with only two major methods that are: enroll() and authenticate().
enroll() is for enrolling (registering) new users, while authenticate() is for recognizing previously enrolled users. Before discussing these methods, let’s link the fio.js library to our react application first. For that, just open the index.html file in your project and link FaceIO CDN to your project.
<body>
<script src="https://cdn.faceio.net/fio.js"></script>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
</body>
After successfully linking the FaceIO CDN, open App.jsx file and delete all the boilerplate code inside App component. To initiate our FaceIO library, we add an useEffect react hook. The code inside this hook runs only once when the component is initiated.
import "./App.css";
import { useEffect } from "react";
function App(){
let faceio;
useEffect(() => {
faceio = new faceIO("your-app-id"); // Get yours from https://console.faceio.net
}, []);
}
You can see we are using an ID as an argument while instantiating the faceIO object. When you signup for a faceIO account, you can access these IDs inside the faceIO console.
All the setup is now completed and let’s focus on authenticating the user using FaceIO.
Enroll (Register) New User via Face Recognition
FaceIO provides a very simple interface to register new users. We use enroll function to register a new user. As FaceIO needs your webcam permission, do accept the permission dialogue when prompted.
The enroll function has 2 aliases. You can use register and record functions as drop-in replacements for enroll.
When you run this function in your browser, it launches the faceIO widget. At first, it takes user consent to scan their face, then prompt to accept
the webcam permission. If all requirements are satisfied, the faceIO
widget opens your camera and scans your face. It converts your face data
into an array of floating point numbers.
After collecting the facial data, faceIO is prompted to enter a PIN code. This PIN code is used to distinguish users with extremely similar faces. You think of this as a two-factor auth when something goes south.
Let’s add faceIO enroll in our react application. We keep the app very simple. Therefore, let’s create a button called register when someone clicks on that button, we run the enroll function to enroll a new user.
After adding the register button and the javascript handler on the button click, App.jsx file looks like this.
import "./App.css";
import { useEffect } from "react";
function App() {
let faceio;
useEffect(() => {
faceio = new faceIO("fioa414d");
}, []);
const handleRegister = async () => {
try {
let response = await faceio.enroll({
locale: "auto",
payload: {
email: "[email protected]",
},
});
console.log(` Unique Facial ID: ${response.facialId}
Enrollment Date: ${response.timestamp}
Gender: ${response.details.gender}
Age Approximation: ${response.details.age}`);
} catch (error) {
console.log(error);
}
};
return (
<section className="w-full h-screen flex flex-col items-center justify-center">
<h1 className="font-sans font-bold text-xl mb-4">
Face Authentication by FaceIO
</h1>
<div className="flex flex-col justify-center items-center">
<button
className="block px-4 py-2 outline-none bg-blue-500 rounded text-white mb-2"
onClick={handleRegister}
>
register
</button>
</div>
</section>
);
}
export default App;
In the above code, you can see I wrap the code inside a try-catch block. If something goes wrong, FaceIO throws some errors. The error messages are very helpful and you can get a complete list of error codes in the official documentation.
The enroll function accepts only an optional parameter object. These
optional parameters accept properties to configure the user registration
process. In the above example, I define locale and payload properties inside the optional parameter of the enrolling function. the locale property represents the default interaction language of your user. In our case, we provide auto to automatically detect the language.
Inside the payload, you can add any JSON serializable value unique to your user. In this example, we are adding the email of the user as a payload. Whenever use log-in in the future, we can access the email payload from the FaceIO return object.
Enroll function in FaceIO returns a promise. Therefore in the example, I am using the async/await feature of javascript. If you don’t prefer async/await, you can use .then instead.
When the promise completes, it results in an userInfo object. By using the object, we can access the unique facial ID of the user. In addition to this, the userInfo object also provides a details property from which, we can get the probable age and gender of the user.
Authenticate a Previously Enrolled User
After registering the user successfully, this is the time to authenticate registered users. For this purpose, Pixlab provides the authenticate function. This authenticate function also has 3 aliases, auth, recognize and identify.
The authentication function needs only a single frame for the user to
recognize. Therefore, it is very bandwidth friendly. After successful
authentication, it returns a userData object. This userData contains the payload you have specifies in the registration and the face of the user.
Inside our App.jsx file, we make another button called Log-in. When a user clicks on this button, it invokes the handleLogIn helper function. This function is ultimately responsible to invoke the “authenticate” function. Let’s see all of these in code:
import "./App.css";
import { useEffect } from "react";
function App() {
let faceio;
useEffect(() => {
faceio = new faceIO("fioa414d");
}, []);
const handleRegister = async () => {
try {
let response = await faceio.enroll({
locale: "auto",
payload: {
email: "[email protected]",
},
});
console.log(` Unique Facial ID: ${response.facialId}
Enrollment Date: ${response.timestamp}
Gender: ${response.details.gender}
Age Approximation: ${response.details.age}`);
} catch (error) {
console.log(error);
}
};
return (
<section className="w-full h-screen flex flex-col items-center justify-center">
<h1 className="font-sans font-bold text-xl mb-4">
Face Authentication by FaceIO
</h1>
<div className="flex flex-col justify-center items-center">
<button
className="block px-4 py-2 outline-none bg-blue-500 rounded text-white mb-2"
onClick={handleRegister}
>
register
</button>
</div>
</section>
);
}
REST API
While creating your account in FaceIO, you will be assigned an API key. You
can find this API key in the faceIO console. By using this API key, you
can interact with the FaceIO back-end with RESTful API. The base URL of the REST API is `https://api.faceio.net/`.
Currently, the FaceIO REST API supports 4 API Endpoints. Deleting the face of a user, setting the additional payload to a Facial ID, and setting the Pin code for a Facial ID. Do remember to use those API endpoints from a secured environment or from your backend server. Don’t run those APIs from the client side.
Deleting a Facial ID
To delete a given Facial ID, we have to make a get request to https://api.faceio.net/deletefacialid endpoint. This endpoint has two request parameters. One is the key that accepts the API key, another is the fid which accepts the facial ID which you want to delete.
curl — request GET \— url 'https://api.faceio.net/deletefacialid?key=APIkey&fid=FaceID'
As a response, FaceIO returns a status code and a `payload` boolean that
tells if the data along with the payload is successfully deleted or not.
FaceIO Webhooks
Webhooks are used as a communication method between servers. FaceIO provides a rich webhook experience that you can use to update your back-end server. FaceIO sends a webhook on 3 events. enroll when a new user enrolls in your system, auth when an already registered user is authenticated using faceIO, and deletion when a faceID is deleted using the REST API we have discussed above.
Each webhook request is a POST request from the faceIO server and a JSON object. The request body looks like this:
{
"eventName":"String - Event Name",
"facialId": "String - Unique Facial ID of the Target User",
"appId": "String - Application Public ID",
"clientIp": "String - Public IP Address",
"details": {
"timestamp": "Optional String - Event Timestamp",
"gender": "Optional String - Gender of the Enrolled User",
"age": "Optional String - Age of the Enrolled User"
}
}
Name of the each field is self-explanatory about what value it contains. Follow these steps to configure the webhook with the FaceIO server.
1. Connect to your account via the FACEIO Console first.On the console main view, visit the Application Manager.
2. Select the target application for which you want to set up webhooks.
3. Once the target application is selected. Enter your Webhook Endpoint URL for receiving events and save your modification.
4. You’re all set. Upon an event is triggered, FACEIO shall make an HTTP POST request to the URL you have configured.
Conclusion
This is an in-depth guide on adding facial authentication to your React
application. If you like this guide and want to know more about faceIO,
please visit the official documentation page. You can also checkout their FAQ page if you have any questions.
Also published here.
The Web Development and eCommerce Writing Contest is brought to you by Elastic Path in partnership with HackerNoon. Publish your thoughts and experiences on #eCommerce or #web-development to win a cash prize!
Elastic Path is the only commerce company making Composable Commerce accessible for tech teams with unique product merchandising requirements and complex go-to-market strategies. Explore our product today!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK