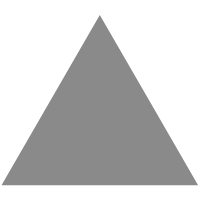
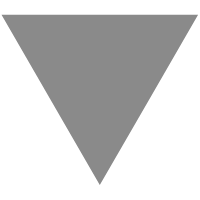
Abap2ui5 Project – Development of UI5 Selection Screens in pure ABAP (no app dep...
source link: https://blogs.sap.com/2023/01/22/abap2ui5-project-development-of-ui5-selection-screens-in-pure-abap-no-app-deployment-or-javascript-needed/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
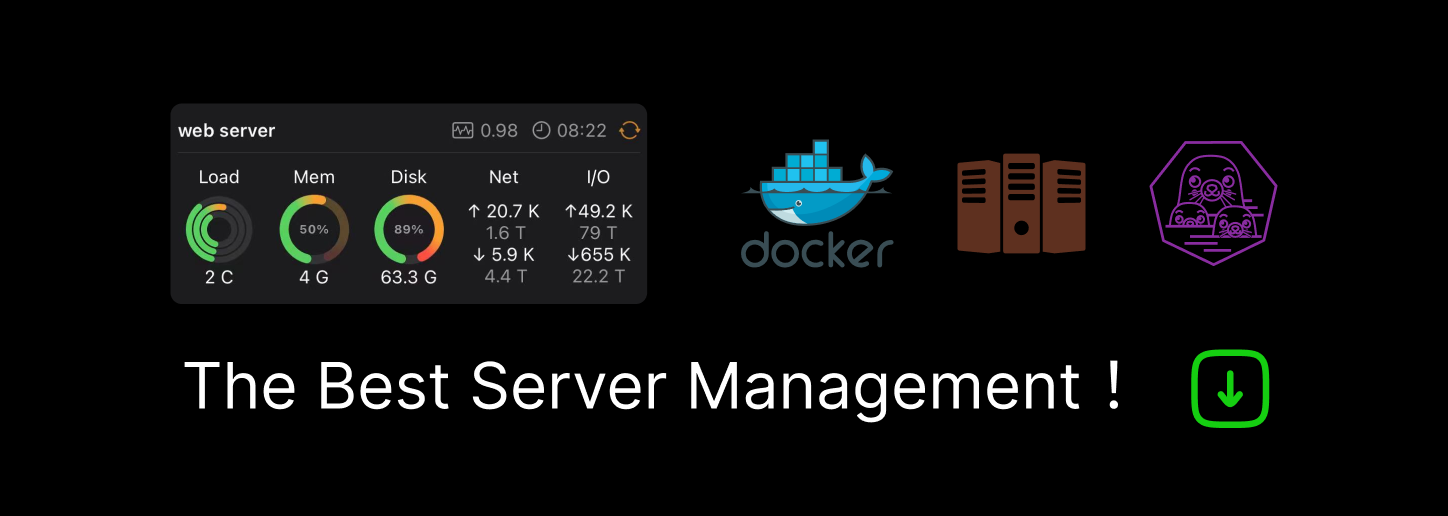
Abap2ui5 Project – Development of UI5 Selection Screens in pure ABAP (no app deployment or javascript needed)
Introduction
In ABAP Cloud there is no SAP GUI anymore and one thing i miss is a lot is the former selection screen. Just write a few lines of abap code and an application with gui and event handling is automatically generated. The IF_OO_ADT_CLASSRUN approach is no replacement, because you can not transfer any inputs and you also do not get a standalone UI5 app usable by an end user.
So how can we bring the selection screen approach to the new (non SAP GUI) ABAP Cloud environment?
One solution is this little abapgit project: abap2ui5 (abapGit)
With abap2ui5 you can create standalone and ready to use UI5 applications in pure ABAP. No app deployment or javascript is needed. Data transfer and event handling in the backend is automatically integrated. At the moment it has the function scope of the former selection screen. Example:

Let’s start with a basic example:
First Start
Install the abap2ui5 project with abapGit first. After that you can find the Z2UI5_IF_APP interface in your system:
INTERFACE z2ui5_if_app PUBLIC.
INTERFACES if_serializable_object.
METHODS set_view
IMPORTING
view TYPE REF TO z2ui5_if_view.
METHODS on_event
IMPORTING
client TYPE REF TO z2ui5_if_client.
ENDINTERFACE.
Next create a new abap2ui5 application by creating a global abap class implementing the above interface. You can use the following code snippet:
CLASS z2ui5_cl_app_demo_01 DEFINITION PUBLIC.
PUBLIC SECTION.
INTERFACES z2ui5_if_app.
DATA product TYPE string.
DATA quantity TYPE string.
DATA check_initialized TYPE abap_bool.
ENDCLASS.
CLASS z2ui5_cl_app_demo_01 IMPLEMENTATION.
METHOD z2ui5_if_app~on_event.
"set initial values
IF check_initialized = abap_false.
check_initialized = abap_true.
product = 'tomato'.
quantity = '500'.
ENDIF.
"user event handling
CASE client->event( )->get_id( ).
WHEN 'BUTTON_POST'.
"do something
client->popup( )->message_toast( |{ product } { quantity } ST - GR successful| ).
ENDCASE.
ENDMETHOD.
METHOD z2ui5_if_app~set_view.
"define selection screen
view->factory_selscreen_page( title = 'My ABAP Application - Z2UI5_CL_APP_DEMO_01'
)->begin_of_block( 'Selection Screen Title'
)->begin_of_group( 'Stock Information'
)->label( 'Product'
)->input( product
)->label( 'Quantity'
)->input( quantity
)->button( text = 'Post Goods Receipt' on_press_id = 'BUTTON_POST'
).
ENDMETHOD.
ENDCLASS.
As you can see, you defined the view and some controller logic in abap. So far there is nothing more to do. Your application is now ready to use.
To start the app, call the http interface Z2UI5_HTTP_CLOUD of the project in your browser. You can find the url in this package of the project:

<your_system>:<port>/sap/bc/http/sap/z2ui5_http_cloud?sap-client=100&app=z2ui_cl_app_demo01
When you are on an On-Premise system, you need to find the url by transaction SICF.
Next call the url in your browser and your new app is ready to use:

That is all you have to do! Now feel free to change the view and add your own custom logic to the controller.
Data Transfer & Event Handling
We now change the values on the frontend to potato and the quantity of 200:

Then we set a breakpoint in eclipse and take a look to the backend system:

As you can see the attributes of the class changed to potato and 200. So we got a working data transfer between client and backend and vice versa. Also the message toast is triggered in the backend and thrown in the frontend with the updated values:

Important: For the Value Transfer between frontend and backend, the attributes have to be in the public section of the implemented class.
So how does this work? Let‘s take a deeper look into the functionality.
Functional Background
The abap2ui5 approach is influenced by two general ideas:
First the IF_OO_ADT_CLASSRUN approach is very powerful. As a customer it is very comfortable that you just need to implement one interface. Also the 100% source code based approach makes it very easy to use and copy between systems and projects.
Second in the last year there was this very nice blog post (Thanks to Patrick VILLENEUVE) about the idea of frontend developing based on a single page application combined with asynchronous ajax updates (“JSON over the wire”).
Abap2ui5 is influenced by this two ideas. The user only needs to implement one interface to develop a full working ui5 application and the frontend is kept as small as possible, so there is no specific app deployment or javascript needed. Everything is created in the backend during runtime.
Server-Client Communication:
By calling the abap2ui5 HTTP service, first a generic one page ui5 application is send to the client:

After that every event creates an ajax roundtrip between server and client. The updated values and the event information is send with the request to the backend. The response includes a new UI5 XML View+JSON View Model.

Server->Client:
For example the response of the application in the beginning of the blog post looks like this:
JSON-View-Model:

XML-View:

Client->Server:
And after the input changed the request with the updated values and event information looks like this:

Persistence, stateful-like feeling:
The HTTP Interface is based on REST, so there is no server session between two requests. Nevertheless the implementation holds all class attributes, because the abap2ui5 interface also implements the interface IF_SERIALIZABLE_OBJECT. With every request a UUID is created and the object is saved to the database:

The next request then recreates the object. For example, this is the reason why the check_initialized attribute is only false for the initial request.
Detailed Example
Now you can create more complex applications, there are a lot of controls still working to build your own selection screens. Take a look to the GitHub repository for a more detailed example. It can be implemented in abap like this:

And the controller looks like this:

Project Information
Project features:
- easy to use – 100% source code based, just implement one abap interface for a standalone ui5 app
- lightweight – based on a single http handler (no odata, no segw, no bsp, no rap, no cds), compatible to very old abap stacks
- easy installation – abapgit project, no additional app deployment or javascript needed
Compatible with all availible abap stacks and language versions:
- BTP ABAP Environment (Abap Cloud)
- S/4 Public Cloud (Abap Cloud)
- S/4 Private Cloud or On-Premise (Abap Cloud, Abap Standard)
- R/3 NW 7.5 and downport to old releases possible (Abap Standard)
Summary
The goal of the abap2ui5 project is to create an easy and fast way to generate selection screens in the new abap cloud environment. Of course it is also working on an on-premise system. The customer has minimum effort to create apps and is not forced to learn new technologies and languages. The knowledge of abap and the former selection screen logic is enough to use this project and create ui5 applications.
On abap2ui5 on (abapGit) you find all information you need to use this project.
So what do you think of this approach?
Next steps could be including more controls and extend the event handling.
Thanks for reading this blog post!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK