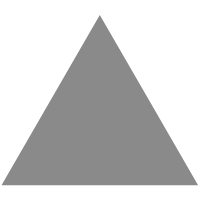
8
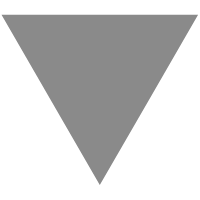
GO语言操作Elasticsearch
source link: https://blog.51cto.com/lzcit/6010742
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
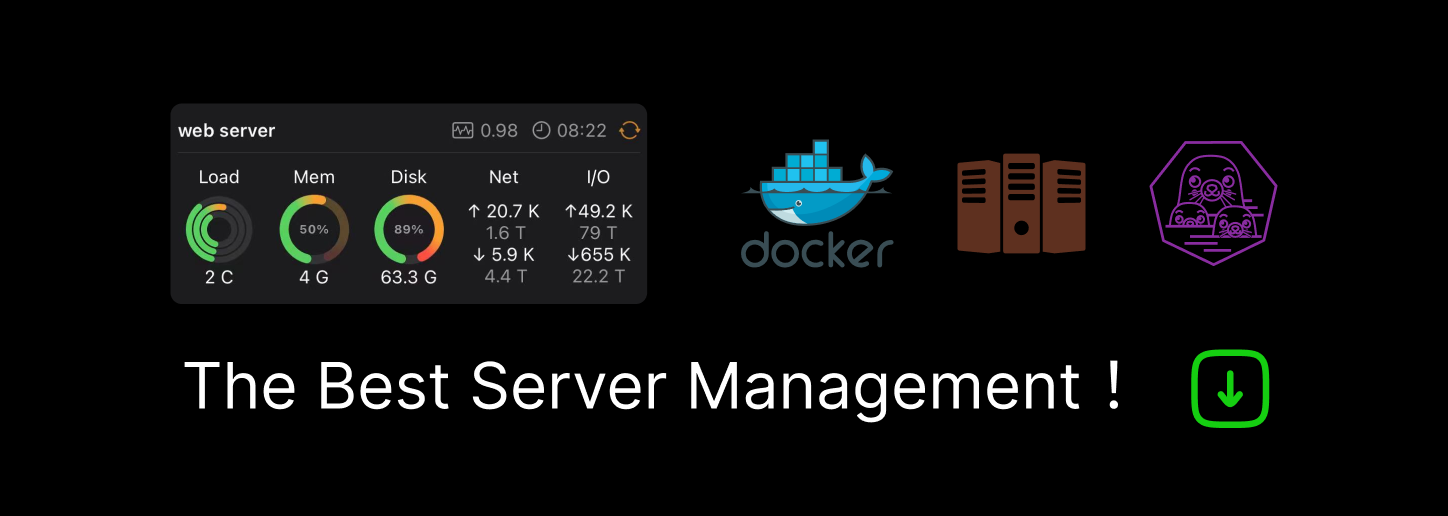
GO语言操作Elasticsearch
精选 原创Elasticsearch简介
Elasticsearch 是一个开源的搜索引擎,建立在一个全文搜索引擎库 Apache Lucene™ 基础之上。 Lucene 可以说是当下最先进、高性能、全功能的搜索引擎库–无论是开源还是私有。
连接Elasticsearch
// 引入g~
~~~~~-elasticsearch
import (
es8 "github.com/elastic/go-elasticsearch/v8"
)
// go-es配置
conf := es8.Config{
Addresses: "http://127.0.0.1:9200",
Username:"elastic",
Password:"jloMQ7ZCTlcZUr_hmDoB",
}
// 创建
client, err := es8.NewClient(conf);
if err != nil{
fmt.Println("============= 创建 elasticsearch 失败 =============")
return
}
// 连接
_, err1 := client.Info()
if err1 != nil{
fmt.Println("============= 连接 elasticsearch 失败 =============")
return
}
创建model结构体
type Admin struct{
Id int `gorm:"<-" json:"id"`
UserName string `gorm:"<-" json:"user_name"`
RealName string `gorm:"<-" json:"real_name"`
Mobile string `gorm:"<-" json:"mobile"`
}
初始化model
admin := Admin{
Id: 1,
UserName: "test",
RealName: "测试",
Mobile: "15222222222",
}
// 结构体json序列化
str,err := json.Marshal(admin);
if err != nil{
return ;
}
// 创建索引
res1, err1 := client.Index(
"db.table",
bytes.NewReader(str),
client.Index.WithDocumentID("1"), // 索引ID
client.Index.WithRefresh("true") //是否立即创建
);
创建返回结构体
type EsResponse struct{
Hits struct{
Total struct{
Value int `json:"value"`
} `json:"total"`
Hits []struct{
Index string `json:"_index"`
Id string `json:"_id"`
Score float32 `json:"_score"`
Source map[string]any `json:"_source"`
} `json:"hits"`
} `json:"hits"`
}
type EsData struct{
Total int `json:"total"`
List []map[string]any `json:"list"`
}
query := map[string]any{
"query":map[string]any{
"bool":map[string]any{
"must":[]map[string]any{
map[string]any{
"match_phrase":map[string]any{
"user_name": "test",
},
},
map[string]any{
"match_phrase":map[string]any{
"mobile": "15222222222",
},
},
},
},
},
}
str,err := json.Marshal(query);
if err != nil{
return ;
}
res1,err1 := client.Search(client.Search.WithBody(bytes.NewReader(str)));
if err1 != nil{
return ;
}
var resData EsResponse;
err2 := json.NewDecoder(res1.Body).Decode(&resData);
if err2 != nil{
return ;
}
var esData EsData;
esData.Total = resData.Hits.Total.Value;
for _, v := range resData.Hits.Hits {
cache := v.Source
cache["_index"] = v.Index;
cache["_id"] = v.Id;
cache["_score"] = v.Score;
esData.List = append(esData.List,cache)
}
update := map[string]any{
"script": map[string]any{
"source":"ctx._source.user_name='test1';ctx._source.mobile='15211111111';",
"lang": "painless",
},
}
str,err1 := json.Marshal(update)
if err1 != nil{
return ;
}
res2,err2 := client.Update(
"db.table",
"1",
bytes.NewReader(str),
client.Update.WithRefresh("true")
)
if err2 != nil{
return ;
}
update := map[string]any{
"query":map[string]any{
"bool":map[string]any{
"must":[]map[string]any{
map[string]any{
"match_phrase":map[string]any{
"user_name": "test",
},
},
map[string]any{
"match_phrase":map[string]any{
"mobile": "15222222222",
},
},
},
},
},
"script": map[string]any{
"source":"ctx._source.user_name='test1';ctx._source.mobile='15211111111';",
"lang": "painless",
},
}
str,err1 := json.Marshal(update)
if err1 != nil{
return ;
}
res2,err2 := client.UpdateByQuery(
[]string{
"db.table",
},
client.UpdateByQuery.WithBody(bytes.NewReader(str)),
client.UpdateByQuery.WithRefresh(true)
)
if err2 != nil{
return ;
}
res,err := client.Delete(
"db.table",
"1",
client.Delete.WithRefresh("true")
)
if err != nil{
return ;
}
query := map[string]any{
"query":map[string]any{
"bool":map[string]any{
"must":[]map[string]any{
map[string]any{
"match_phrase":map[string]any{
"user_name": "test",
},
},
map[string]any{
"match_phrase":map[string]any{
"mobile": "15222222222",
},
},
},
},
},
}
str,err := json.Marshal(query);
if err != nil{
return ;
}
res,err := client.DeleteByQuery(
[]string{
"db.table",
},
bytes.NewReader(str),
client.DeleteByQuery.WithRefresh(true)
)
- 赞
- 收藏
- 评论
- 分享
- 举报
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK