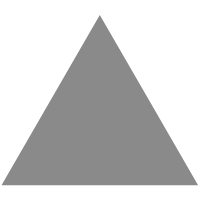
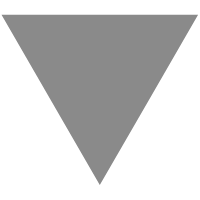
Display GitHub Repositories with SvelteKit
source link: https://blog.robino.dev/posts/github-repos-api
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
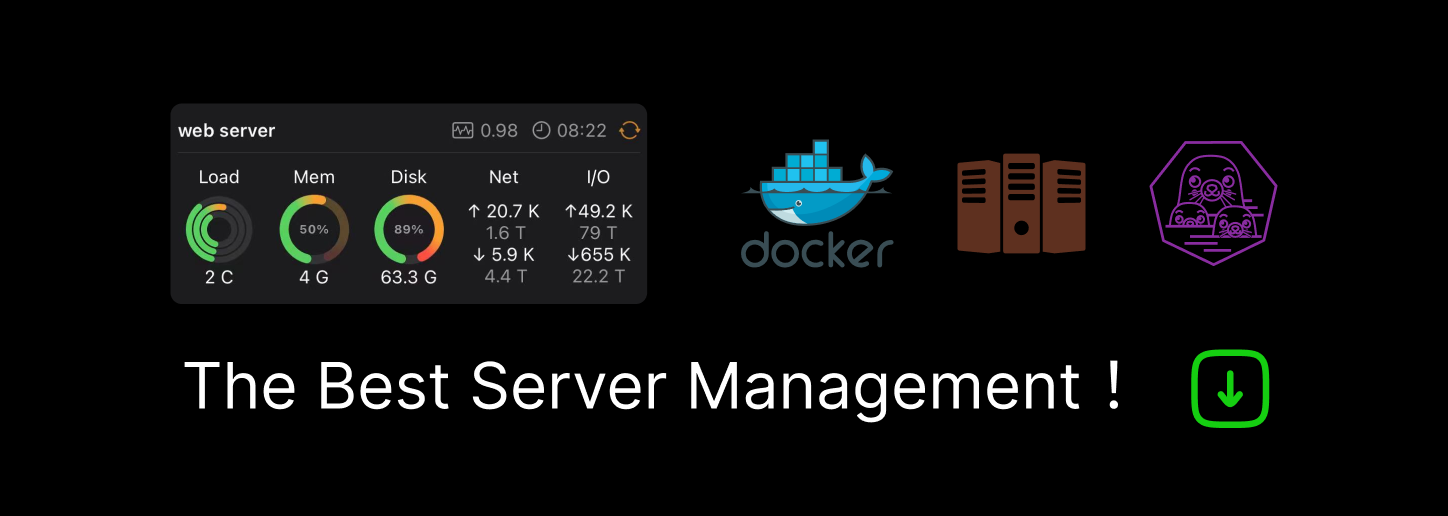
Display GitHub Repositories with SvelteKit
Use the GitHub API to display repositories on your portfolio page.
Jan 4, 2023
GitHub API
GitHub provides an API that allows you to easily pull information about your repositories without an API key.
You can pull the public information from GitHub with the following urls. These three serve as quick examples of what is possible. Click the links below to see the data for my GitHub account, or switch out my username with yours to see your information. The .../repos
page serves as a useful reference to see all the different data we can get from the API.
Loader
First we can create a loader to fetch the data from the API. If you haven’t used Svelte or SvelteKit before, the best place to learn more is the official tutorial.
Fetch the data on the server
We can use +page.server.js
to load data on the server before rendering the page. This is a good place to make our fetch
request to the GitHub API.
Repository data points
As you probably noticed above when clicking the link, GitHub provides a vast amount of data we can read on our page. Here are a few basic data points that I chose to use.
name
: name of the repositoryfull_name
: name with username in front (we can use this to link to the repository page)homepage
: click on the gear icon in the upper right corner of a repository, this is the Website field — this is a link to the live page of your website if you have one for your repositorydescription
: located in the same location as the homepage — a brief description of your projecthtml_url
: link to the GitHub repository itself
// +page.server.js
export async function load({ fetch }) {
const res = await fetch("https://api.github.com/users/rossrobino/repos");
const repos = await res.json();
// sort by pushed_at date, most recent first
repos.sort((a, b) => new Date(b.pushed_at) - new Date(a.pushed_at));
// creates a new array with just the data points needed for the page
return {
repos: repos.map((repo) => ({
name: repo.name,
full_name: repo.full_name,
homepage: repo.homepage,
description: repo.description,
html_url: repo.html_url,
})),
};
}
Render
We can now access this data in +page.svelte
contained in the same directory with the data
prop and use an {#each}
block to iterate through the list.
<!-- +page.svelte -->
<script>
export let data;
</script>
<h2>Portfolio</h2>
<div>
<!-- iterate through each repo in the data.repos list -->
{#each data.repos as repo}
<div>
<h3>
<a href={repo.homepage}>
{repo.name}
</a>
</h3>
{#if repo.description}
<div>{repo.description}</div>
{/if}
<div>
<a href={repo.html_url}>
{repo.full_name}
</a>
</div>
</div>
{/each}
</div>
Conclusion
That’s all you need to server side render a list of GitHub repos to a page with SvelteKit. You can continue and add some styles or different data points you find interesting. Check out my component styled with tailwindcss and view it on my portfolio page. Thank you for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK