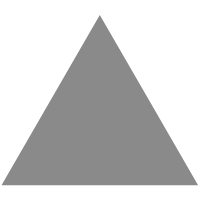
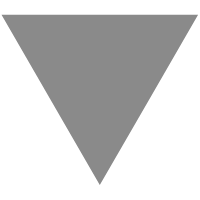
JavaScript 中三种定义函数的方式
source link: https://blog.51cto.com/u_15921635/5970954
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
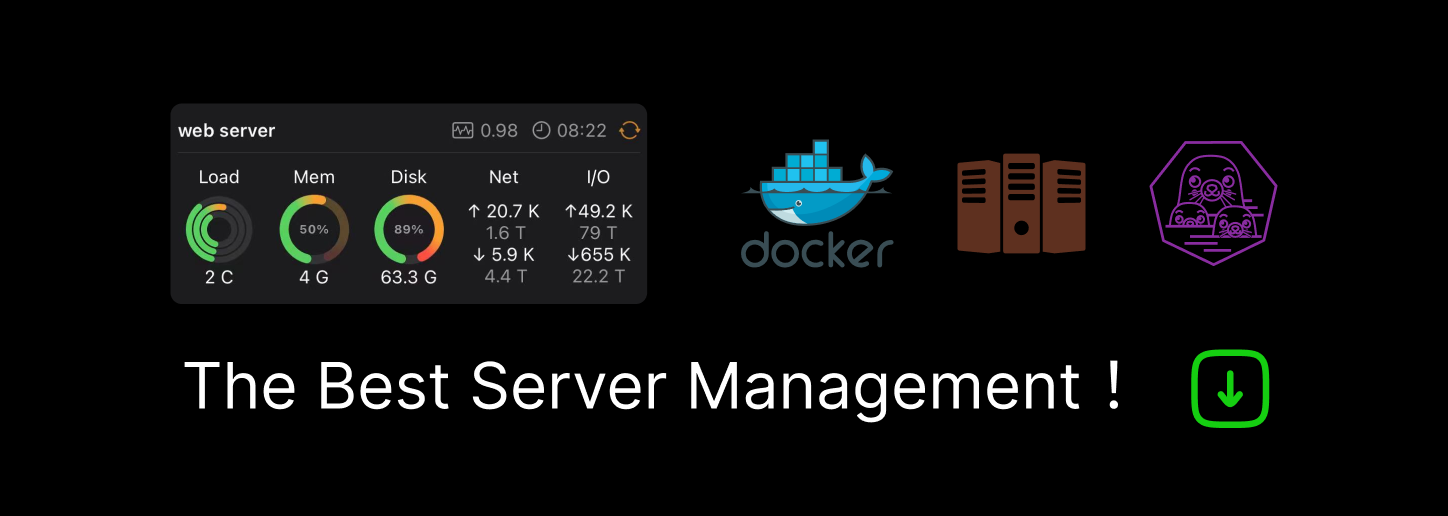
Hello,我是CoderBug,今天我们来讲讲在JavaScript中的三种定义函数的方式.
1.什么是JavaScript 函数?
JavaScript 函数是用来执行特定任务的可重复使用的代码块。你可以使用函数来组织你的代码,使其变得更加清晰、结构化,并且更容易维护。
在 JavaScript 中,你可以使用以下三种方式定义函数:
- 函数声明:使用
function
关键字来定义函数。 - 函数表达式:将一个匿名函数赋值给一个变量。
- 箭头函数:使用箭头语法来定义函数。
注意:箭头函数只能在使用函数表达式定义函数时使用。它不能作为函数声明使用。
2.函数声明:使用 function
关键字来定义函数
在 JavaScript 中,你可以使用函数声明的方式来定义函数。例如,你可以使用以下语法来定义一个名为 "add" 的函数,用来计算两个数的和:
return x + y;
}
你也可以使用函数声明的方式来定义带有返回值的函数,例如:
return "Hello, " + name + "!";
}
还有一种常见的使用函数声明的方式是,定义一个函数来执行特定任务,并将其作为一个模块导出,供其他文件使用。例如,你可以定义一个名为 "math" 的函数来执行数学运算,并将其导出为模块:
//计算两个数的和
function add(x, y) {
return x + y;
}
计算两个数的差
function subtract(x, y) {
return x - y;
}
//计算两个数的积
function multiply(x, y) {
return x * y;
}
function divide(x, y) {
return x / y;
}
module.exports = {
add: add,
subtract: subtract,
multiply: multiply,
divide: divide,
};
在其他文件中,你可以使用 require
函数来加载这个模块,并使用点语法调用其中的函数:
const math = require("./math");
console.log(math.add(6, 7)); // Output: 13
console.log(math.subtract(3, 7)); // Output: -4
console.log(math.multiply(7, 7)); // Output: 49
console.log(math.divide(5, 7)); // Output: 0.7142857142857143
2.函数表达式:将一个匿名函数赋值给一个变量
let add = function(x, y) {
return x + y;
};
//定义带有返回值的函数
let greet = function(name) {
return "Hello, " + name + "!";
};
你还可以使用函数表达式的方式来定义匿名函数,例如:
return x * y;
}(5, 7);
console.log(result); // Output: 35
你还可以使用箭头函数语法来简化函数表达式。例如,你可以使用以下语法来定义一个名为 "divide" 的函数,用来计算两个数的商:
return x / y;
};
3.箭头函数:使用箭头语法来定义函数
在 JavaScript 中,你可以使用箭头函数的方式来定义函数。箭头函数是一种简化的函数表达式,它比传统的函数表达式更简洁。
使用以下语法来定义一个名为 "add" 的函数,用来计算两个数的和:
return x + y;
};
使用箭头函数的方式来定义带有返回值的函数:
return "Hello, " + name + "!";
};
使用箭头函数来定义不带任何参数的函数:
console.log("Hello, world!");
};
sayHello(); // Output: "Hello, world!"
使用箭头函数来定义带有单个参数的函数:
return x * 2;
};
console.log(double(5)); // Output: 10
使用箭头函数来定义匿名函数:
return x * y;
})(5, 7);
console.log(result); // Output: 35
如有帮助,麻烦点个赞,如有错误请指出,我是CoderBug,一个跟你一样追风的少年!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK