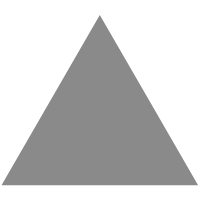
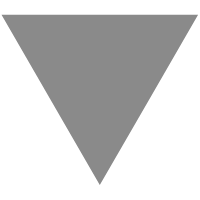
Testing State restoration - Compose
source link: https://proandroiddev.com/testing-state-restoration-compose-c80c18344f94
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
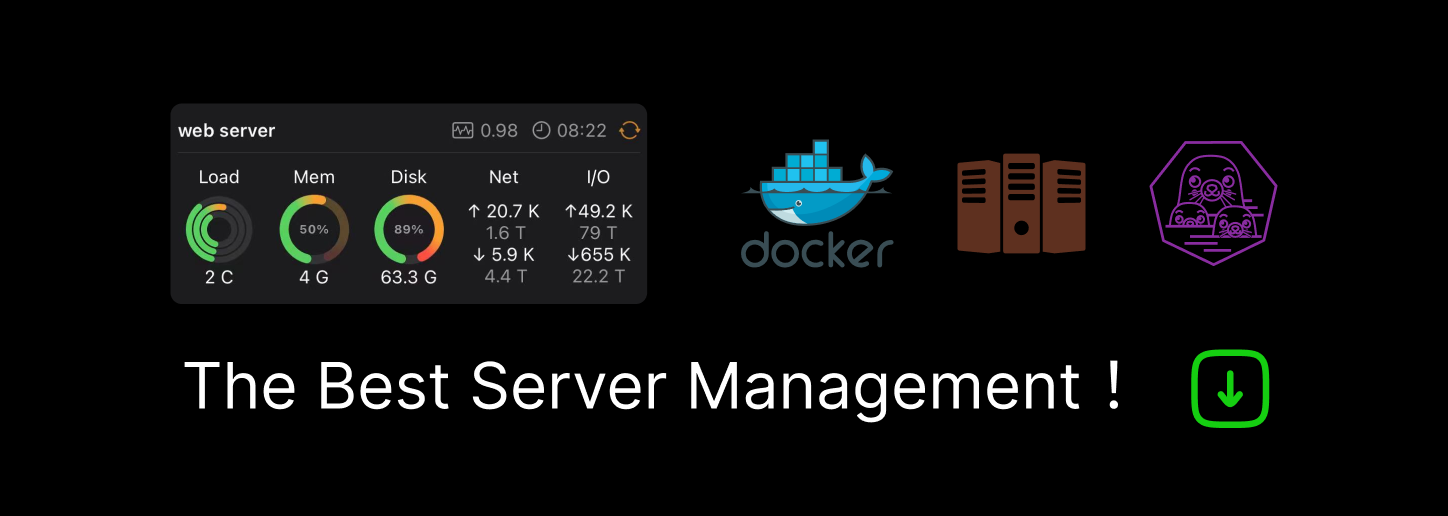
Testing State restoration - Compose

I have recently started adding UI tests for all the Composables in one of my side projects.
So I have the following composable, which uses rememberSaveable to survive configuration changes.
@Composable
fun UserNameComposable() {
var name by rememberSaveable() {
mutableStateOf("Nav Singh")
}
Column(modifier = Modifier.padding(16.dp)) {
Text(
text = "User name is: $name",
style = TextStyle(fontWeight = FontWeight.ExtraBold)
)
Spacer(modifier = Modifier.size(32.dp))
OutlinedButton(onClick = { name = "Navjot Singh" }) {
Text(text = "Change name")
}
}
}
Composable preview:

There are other tests I need to perform, but I want to ensure that this composable can survive changes such as activity recreation, etc.
My search for How to test state restoration in Compose led me to the following official documentation:
- Fortunately, we have an inbuilt class that helps us to accomplish this 😇.
- Its called StateRestorationTester
Now let’s take a look at how this class can test state restoration
- Its basically 5️⃣ step process
- Create StateRestorationTester’s instance
val restorationTester = StateRestorationTester(composeTestRule)
2. Set the content on the instance that we have created in the 1st step
restorationTester.setContent{
UserNameComposable()
}
3. Run any action that modifies the state
- In our case, Whenever we click on the button it
sets
the name = “Navjot Singh”
// Change name is the text of Button
composeTestRule.onNodeWithText("Change name").performClick()
4. Trigger the restoration
- We will call the method called
emulateSavedInstanceStateRestore()
onrestorationTester
instance.
restorationTester.emulateSavedInstanceStateRestore()
5. Final step, Verify the result
// Verify if Node still has the name that was set by the button's onClick
composeTestRule.onNodeWithText("User name is: Navjot Singh")
.assertIsDisplayed()
Here is the full sample code 🧑💻👩💻
// Composable
@Composable
fun UserNameComposable() {
var name by rememberSaveable() {
mutableStateOf("Nav Singh")
}
Column(modifier = Modifier.padding(16.dp)) {
Text(
text = "User name is: $name",
style = TextStyle(fontWeight = FontWeight.ExtraBold)
)
Spacer(modifier = Modifier.size(32.dp))
OutlinedButton(onClick = { name = "Navjot Singh" }) {
Text(text = "Change name")
}
}
}
// Restoration test
@RunWith(AndroidJUnit4::class)
class StateRestorationTest {
@get:Rule
val composeTestRule = createComposeRule()
@Test
fun onRecreation_stateIsRestored() {
// 1.
val restorationTester = StateRestorationTester(composeTestRule)
// 2.
restorationTester.setContent {
UserNameComposable()
}
// OPTIONAL: Verify the initial state before restoration
composeTestRule.onNodeWithText("User name is: Nav Singh")
.assertIsDisplayed()
// 3. Run actions that modify the state
composeTestRule.onNodeWithText("Change name")
.performClick()
// 4. Trigger a recreation
restorationTester.emulateSavedInstanceStateRestore()
// 5. Verify that state has been correctly restored.
composeTestRule.onNodeWithText("User name is: Navjot Singh")
.assertIsDisplayed()
}
}

😊😊 👏👏👏👏 HAPPY CODING 👏👏👏👏 😊😊
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK