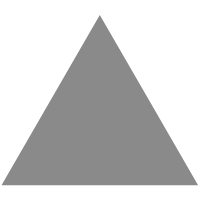
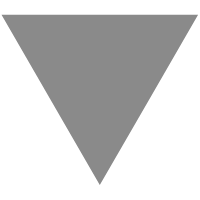
How to determine if variable is undefined or null in JavaScript
source link: https://www.laravelcode.com/post/how-to-determine-if-variable-is-undefined-or-null-in-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
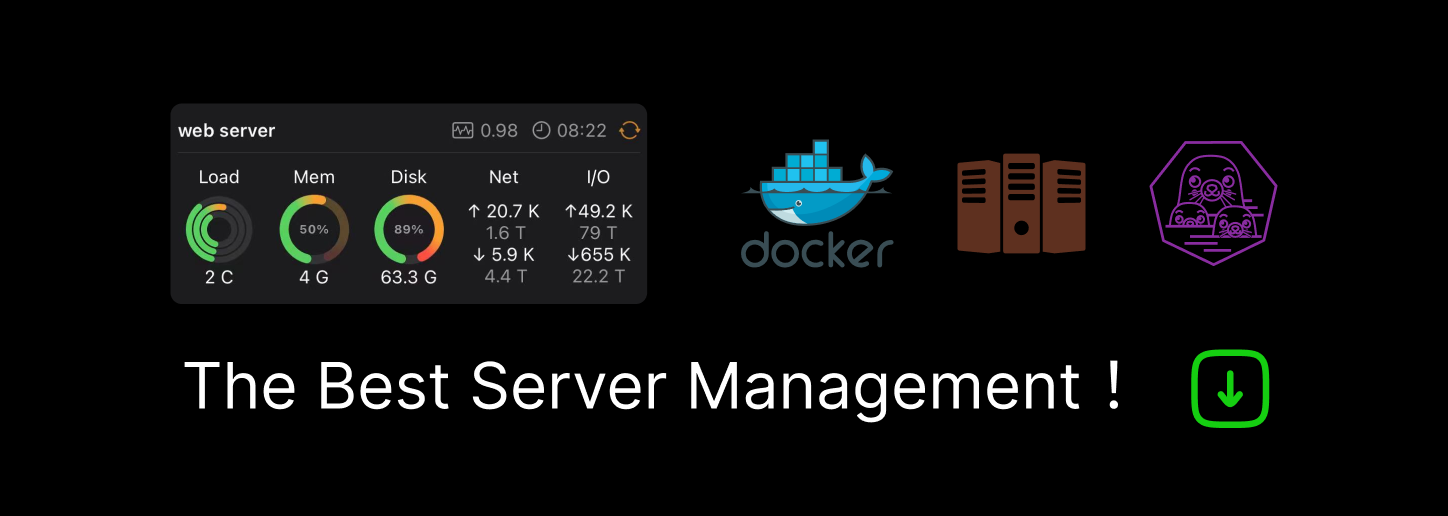
How to determine if variable is undefined or null in JavaScript
Use the equality operator (==
)
In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined
. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null
is a special assignment value, which can be assigned to a variable as a representation of no value.
In simple words you can say a null
value means no value or absence of a value, and undefined
means a variable that has been declared but no yet assigned a value.
To check if a variable is undefined or null you can use the equality operator ==
or strict equality operator ===
(also called identity operator). Let's take a look at the following example:
<script>
var firstName;
var lastName = null;
// Try to get non existing DOM element
var comment = document.getElementById('comment');
console.log(firstName); // Print: undefined
console.log(lastName); // Print: null
console.log(comment); // Print: null
console.log(typeof firstName); // Print: undefined
console.log(typeof lastName); // Print: object
console.log(typeof comment); // Print: object
console.log(null == undefined) // Print: true
console.log(null === undefined) // Print: false
/* Since null == undefined is true, the following statements will catch both null and undefined */
if(firstName == null){
alert('Variable "firstName" is undefined.');
}
if(lastName == null){
alert('Variable "lastName" is null.');
}
/* Since null === undefined is false, the following statements will catch only null or undefined */
if(typeof comment === 'undefined') {
alert('Variable "comment" is undefined.');
} else if(comment === null){
alert('Variable "comment" is null.');
}
</script>
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK