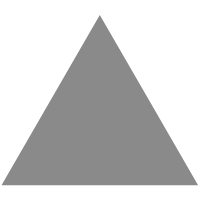
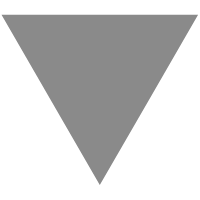
如何在 Golang 中编写 RESTful API 客户端
source link: https://www.jdon.com/63947.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
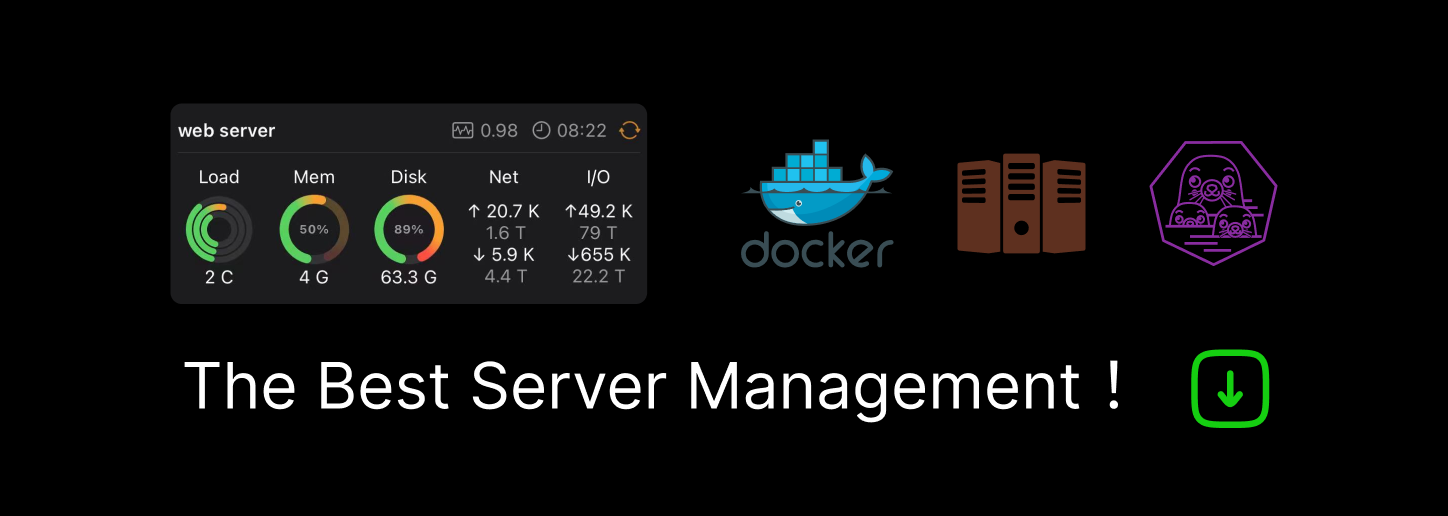
如何在 Golang 中编写 RESTful API 客户端
RESTful API 客户端允许开发人员与 RESTful API 交互以执行各种操作,例如发送请求和接收响应。本教程将教我们如何使用流行的 net/http 包在 Golang 中编写一个简单的 RESTful API 客户端。
1. 首先,我们需要导入 net/http 包并使用 http.NewClient 函数创建一个新客户端。此函数将超时持续时间作为参数并返回一个新的客户端实例:
import "net/http" client := http.NewClient(10 * time.Second) |
2. 接下来,我们将使用客户端通过调用客户端的Do方法向API发送请求。此方法将 HTTP 请求作为参数并返回 HTTP 响应和错误。
以下是向 API 发送 GET 请求的示例:
req, err := http.NewRequest("GET", "https://api.example.com/users", nil) if err != nil { // handle error } resp, err := client.Do(req) if err != nil { // handle error } defer resp.Body.Close() |
Do 方法发送请求并从 API 返回响应。如果发送请求或接收响应时出错,它将在错误变量中返回。
我们还可以使用请求对象的 Header 字段向请求添加标头:
req.Header.Add("Authorization", "Bearer YOUR_API_TOKEN") req.Header.Add("Content-Type", "application/json") |
如果 API 要求我们在请求体中发送数据,我们可以使用 io.Reader 接口将数据传递给请求。例如,要发送带有 JSON 负载的 POST 请求,我们可以使用 json 包对数据进行编码并将其传递给请求主体:
data := map[string]string{ "name": "John", "email": "[email protected]", } payload, err := json.Marshal(data) if err != nil { // handle error } req, err := http.NewRequest("POST", "https://api.example.com/users", bytes.NewBuffer(payload)) if err != nil { // handle error } req.Header.Add("Content-Type", "application/json") resp, err := client.Do(req) if err != nil { // handle error } defer resp.Body.Close() |
3. 一旦我们收到来自 API 的响应,我们就可以使用 io.Reader 接口读取响应主体。例如,要将响应主体读取为字符串,我们可以使用 ioutil.ReadAll 函数:
body, err := ioutil.ReadAll(resp.Body) if err != nil { // handle error } fmt.Println(string(body)) |
您现在知道如何使用 net/http 包在 Go 中编写一个简单的 RESTful API 客户端。使用这些基本构建块,您可以与任何 RESTful API 交互并执行各种操作。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK