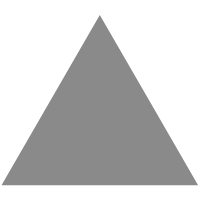
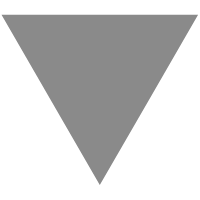
在nodejs中体验http/2 - 空山与新雨
source link: https://www.cnblogs.com/walkermag/p/16995319.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
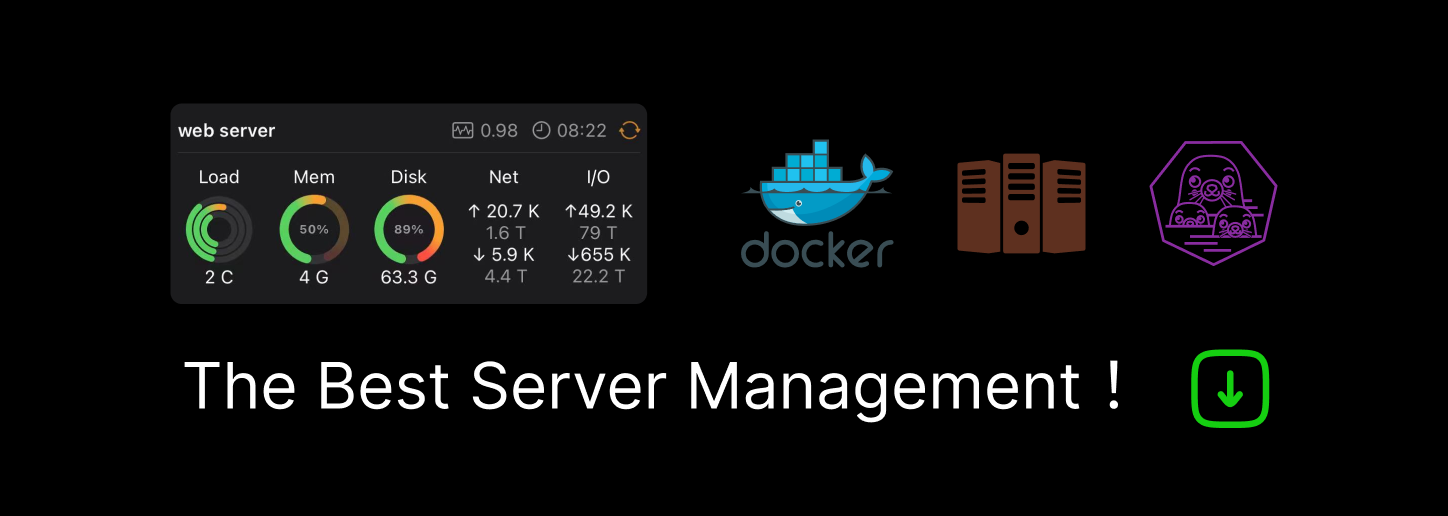
在nodejs中体验http/2
2015年,HTTP/2 发布,直到2021年公司的项目才开始在实践中应用;自己对http2诸多特点的理解只存在于字面上,于是尝试在nodejs中实践一下,加深自己的理解。
同域名下所有通信都在单个连接上完成,消除了因多个 TCP 连接而带来的延时和内存消耗,这在大量请求同时发出的情况下能够减少加载时间。
使用如下代码查看http2环境下,资源下载的情况(浏览器开启限流和disable cache):
const http2 = require('http2');
const fs = require('fs');
const { HTTP2_HEADER_PATH } = http2.constants;
const server = http2.createSecureServer({
key: fs.readFileSync('localhost-privkey.pem'),
cert: fs.readFileSync('localhost-cert.pem')
});
server.on('error', (err) => console.error(err));
server.on('stream', (stream, headers) => {
// stream is a Duplex
const path = headers[':path'];
if(path === '/img.png' || path === '/favicon.ico'){
const fd = fs.openSync('img.png', 'r');
const stat = fs.fstatSync(fd);
const headers = {
'content-length': stat.size,
'last-modified': stat.mtime.toUTCString(),
'content-type': 'image/png'
};
stream.respondWithFD(fd, headers);
} else if(path === '/') {
stream.respond({
'content-type': 'text/html; charset=utf-8',
':status': 200
});
stream.end(`
<h1>Hello World</h1>
<script>
for(var i=0;i<50;i++){
fetch('/img.png')
}
</script>
`);
}
});
server.listen(8443);
可以看到当资源开始同时请求,所有的请求形成一个队列,请求之间开始时间相差大概1ms, 因为下载的是同一个图片,50张图片同时下载,最后几乎在同时完成下载。
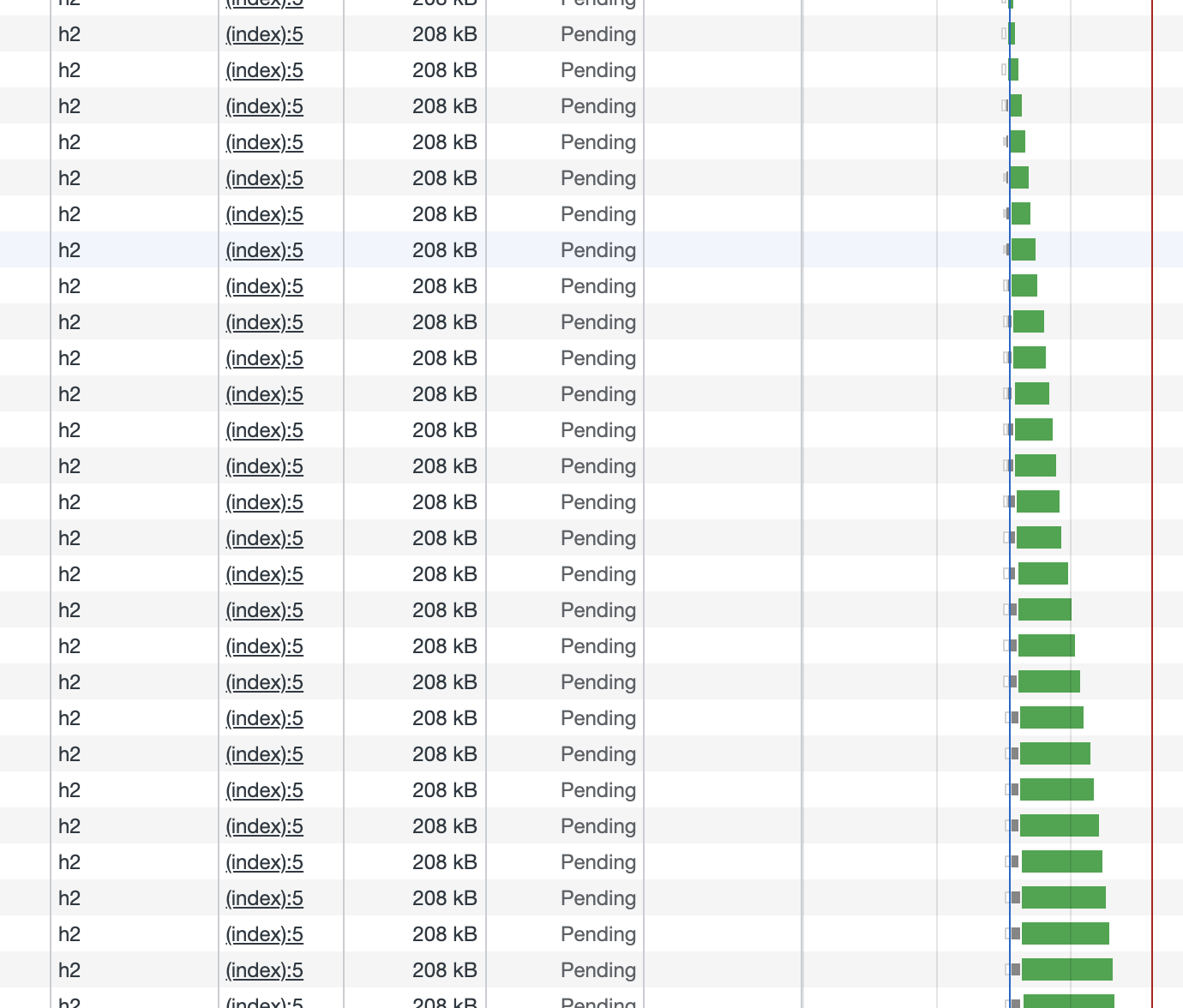
下面是http1.1的例子,通过对比发现浏览器按照自己的最大并发量同时发出请求,只有当请求返回后才发出新的请求(浏览器开启限流和disable cache):
const http = require('http');
const fs = require('fs');
const server = http.createServer(function(req,res){
const path = req.url;
if(path === '/img.png' || path === '/favicon.ico'){
res.writeHead(200,{'Content-type':'image/png'})
var stream = fs.createReadStream('img.png')
stream.pipe(res)
} else {
res.writeHead(200, { 'Content-Type': 'text/html; charset=utf-8' });
res.end(`
<h1>Hello World</h1>
<script>
for(var i=0;i<50;i++){
fetch('/img.png')
}
</script>
`);
}
});
server.listen(8444);
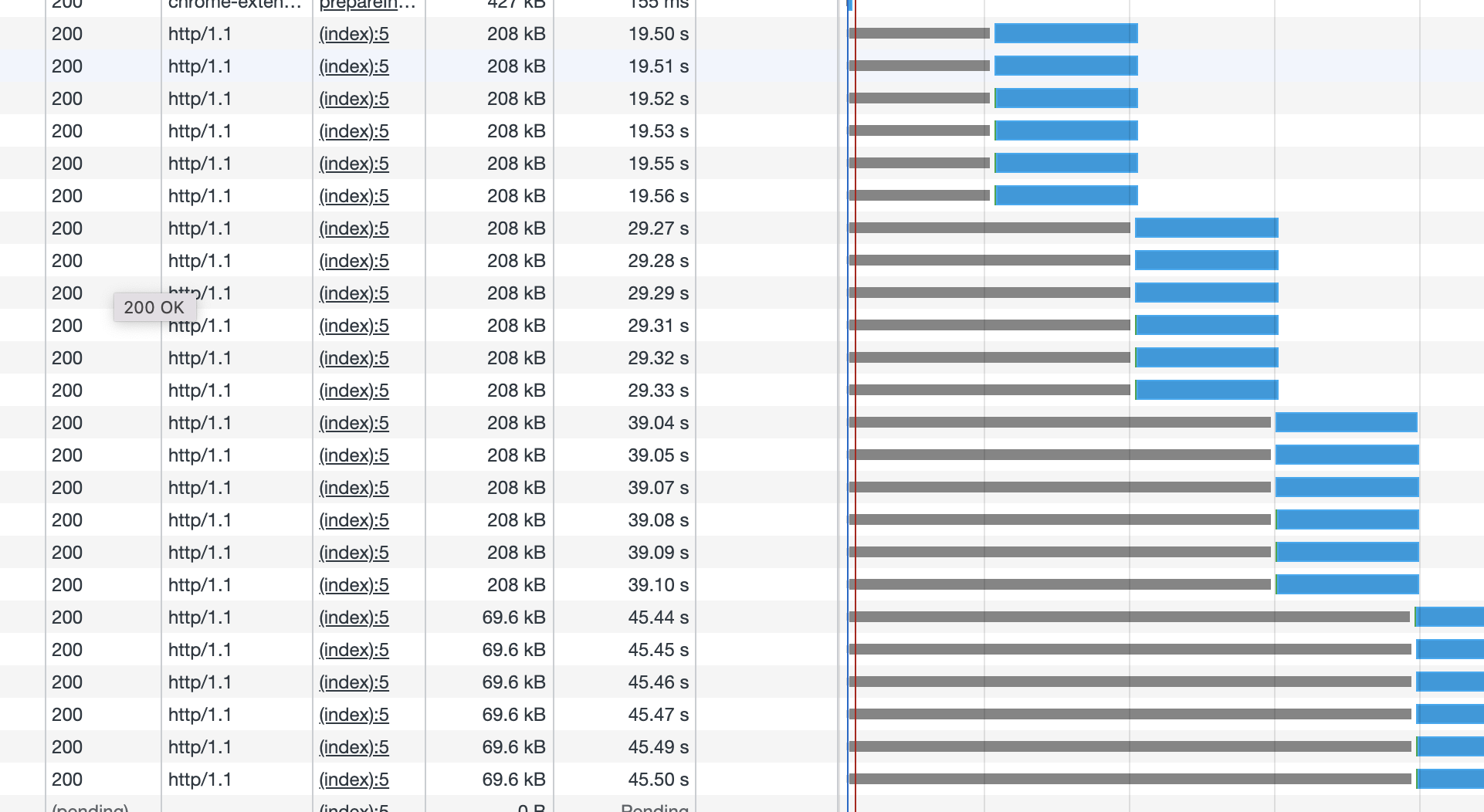
服务端推送
按照如下代码测试
const http2 = require('http2');
const fs = require('fs');
const { HTTP2_HEADER_PATH } = http2.constants;
const server = http2.createSecureServer({
key: fs.readFileSync('localhost-privkey.pem'),
cert: fs.readFileSync('localhost-cert.pem')
});
server.on('error', (err) => console.error(err));
server.on('stream', (stream, headers) => {
const path = headers[':path'];
if(path === '/') {
stream.respond({
'content-type': 'text/html; charset=utf-8',
':status': 200
});
stream.pushStream({ [HTTP2_HEADER_PATH]: '/style.css' }, (err, pushStream, headers) => {
if (err) throw err;
const fd = fs.openSync('style.css', 'r');
const stat = fs.fstatSync(fd);
const header = {
'content-length': stat.size,
'last-modified': stat.mtime.toUTCString(),
'content-type': 'text/css'
};
pushStream.respondWithFD(fd, header)
});
stream.end(`
<h1>Hello World</h1>
<script>
setTimeout(()=>{
fetch('/style.css')
},2000)
</script>
`);
} else if(path === '/style.css'){
const fd = fs.openSync('style.css', 'r');
const stat = fs.fstatSync(fd);
const headers = {
'content-length': stat.size,
'last-modified': stat.mtime.toUTCString(),
'content-type': 'text/css'
};
stream.respondWithFD(fd, headers);
}
});
server.listen(8442);
资源加载情况如下,style.css的Initiator是Push,大小是66 B, 同时首页加载的大小是207 B,
注释掉stream.pushStream
部分后,不使用推送,资源加载如下,style.css大小是89B, 同时首页加载的大小是182B,
综上所看,服务端推送可以提前加载资源,优化非首页加载有益。
令人高兴的是,因为使用率低,chrome在105版本后不再支持http2的服务端推送,导致这个特点在前端开发中可以忽略了。并且如果要测试改特点需要使用低版本的chrome,比如本例子使用的是chrome 96 mac版本。
本文所用代码:https://github.com/blank-x/pg/tree/master/http2,nodejs版本是v16.19.0.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK