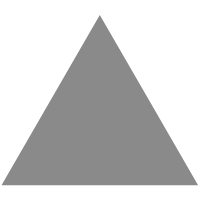
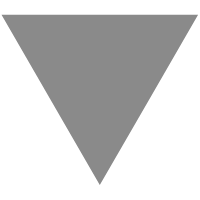
JQuery add or remove class using addClass, removeClass and toggleClass methods
source link: https://www.laravelcode.com/post/jquery-add-or-remove-class-using-addclass-removeclass-and-toggleclass-methods
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
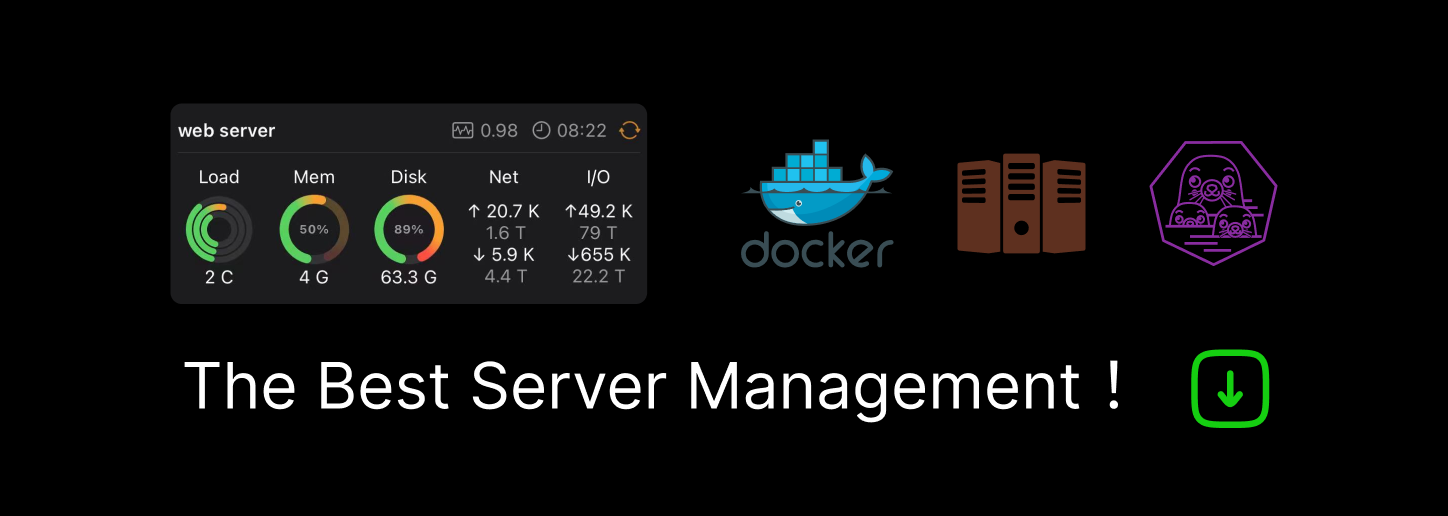
JQuery add or remove class using addClass, removeClass and toggleClass methods
Sometimes you need to change diplay property or behaviour when event occurs like click or hover on specific element. For that purpose, you may direct change property or behaviour using Javascript or JQuery which is not option and makes code length. The better option is to create class with your required CSS and add or remove class with event.
You can add or remove class on event using vanilla Javascript or JQuery. JQuery provides simple method to add or remove class from the element.
In this article, we will use JQuery addClass, removeClass and toggleClass method with example to add or remove class from the element. For the simplicity of code, we will trigger this method on click button.
addClass method
The addClass method add one or more class to the specific element.
Example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JQuery addClass method</title>
<style type="text/css">
.red {
color: red;
}
.italic {
font-style: italic;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p>This is text.</p>
<button>View</button>
<script type="text/javascript">
$('button').click(function() {
$('p').addClass('red italic');
});
</script>
</body>
</html>
removeClass method
removeClass method removes one or multiple class from the element matched from the parameter. If no parameter specified, all class from the element will be removed.
Example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JQuery removeClass method</title>
<style type="text/css">
.red {
color: red;
}
.italic {
font-style: italic;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p class="red italic">This is text.</p>
<button>View</button>
<script type="text/javascript">
$('button').click(function() {
$('p').removeClass('red italic');
});
</script>
</body>
</html>
toggleClass method
toggleClass method checks for toggles one or more classes from the element. If the class already exists, toggleClass removes it and if the class not exists, toggleClass will add the class. toggleClass is useful when you want to toggle between show and hide any element.
Example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JQuery toggleClass method</title>
<style type="text/css">
.red {
color: red;
}
.italic {
font-style: italic;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p class="red">This is text.</p>
<button>View</button>
<script type="text/javascript">
$('button').click(function() {
$('p').toggleClass('red italic');
});
</script>
</body>
</html>
This way, you can manipulate class from the element using JQuery. I hope you liked this article and help you on your work.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK