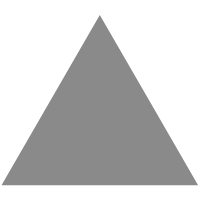
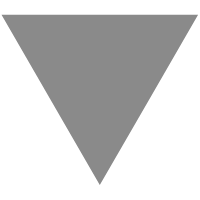
十个高级 TypeScript 开发技巧
source link: https://www.51cto.com/article/722215.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
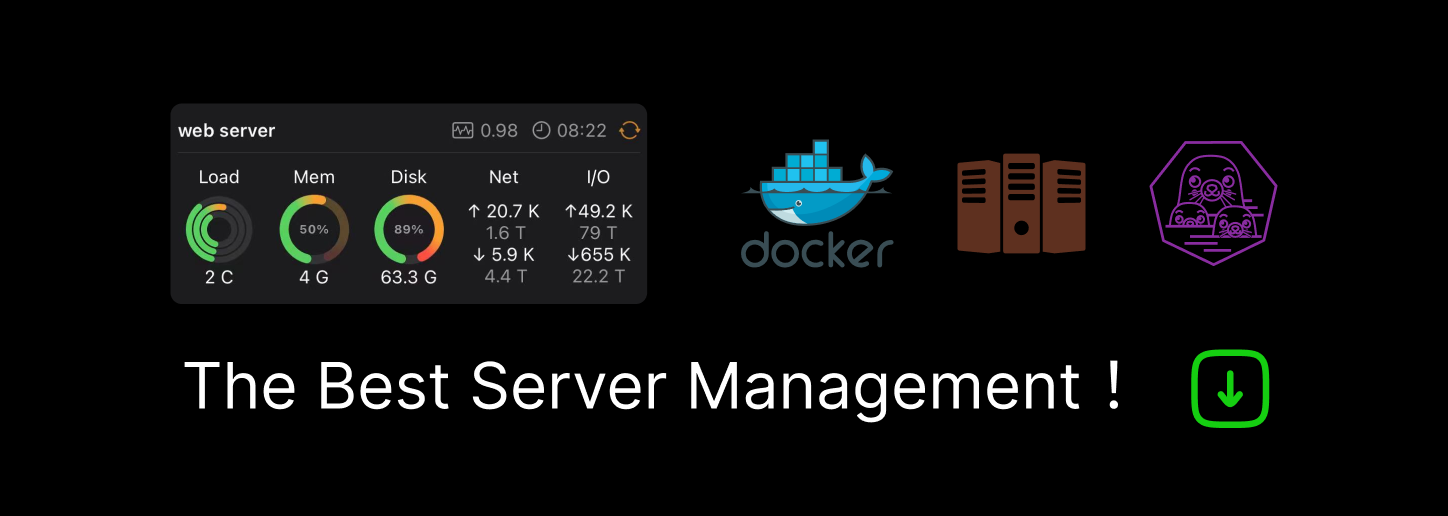
十个高级 TypeScript 开发技巧
在使用了一段时间的 Typescript 之后,我深深地感受到了 Typescript 在大中型项目中的必要性。 可以提前避免很多编译期的bug,比如烦人的拼写问题。 并且越来越多的包都在使用 TS,所以学习它势在必行。
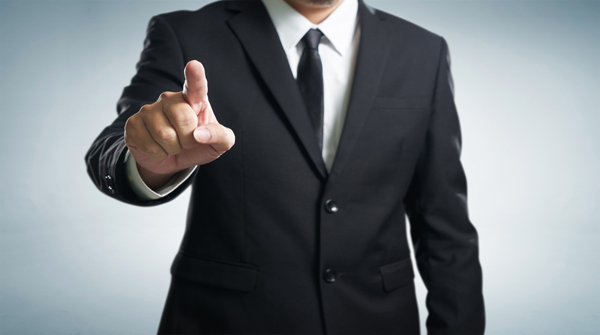
以下是我在工作中学到的一些更实用的Typescript技巧,今天把它整理了一下,分享给各位,希望对各位有帮助。
1.keyof
keyof 与 Object.keys 稍有相似,只是 keyof 采用了接口的键。
interface Point {
x: number;
y: number;
}
// type keys = "x" | "y"
type keys = keyof Point;
假设我们有一个如下所示的对象,我们需要使用 typescript 实现一个 get 函数来获取其属性的值。
const data = {
a: 3,
hello: 'max'
}
function get(o: object, name: string) {
return o[name]
}
我们一开始可能是这样写的,但它有很多缺点:
- 无法确认返回类型:这将失去 ts 的最大类型检查能力。
- 无法约束密钥:可能会出现拼写错误。
在这种情况下,可以使用 keyof 来增强 get 函数的 type 功能,有兴趣的可以查看 _.get 的 type 标签及其实现。
function get<T extends object, K extends keyof T>(o: T, name: K): T[K] {
return o[name]
}
2.必填&部分&选择
既然知道了keyof,就可以用它对属性做一些扩展,比如实现Partial和Pick,Pick一般用在_.pick中
type Partial<T> = {
[P in keyof T]?: T[P];
};
type Required<T> = {
[P in keyof T]-?: T[P];
};
type Pick<T, K extends keyof T> = {
[P in K]: T[P];
};
interface User {
id: number;
age: number;
name: string;
};
// Equivalent to: type PartialUser = { id?: number; age?: number; name?: string; }
type PartialUser = Partial<User>
// Equivalent to: type PickUser = { id: number; age: number; }
type PickUser = Pick<User, "id" | "age">
这些类型内置在 Typescript 中。
3.条件类型
它类似于 ?: 运算符,你可以使用它来扩展一些基本类型。
T extends U ? X : Y
type isTrue<T> = T extends true ? true : false
// Equivalent to type t = false
type t = isTrue<number>
// Equivalent to type t = false
type t1 = isTrue<false>
4. never & Exclude & Omit
never 类型表示从不出现的值的类型。
结合 never 和条件类型可以引入许多有趣和有用的类型,例如 Omit
type Exclude<T, U> = T extends U ? never : T;
// Equivalent to: type A = 'a'
type A = Exclude<'x' | 'a', 'x' | 'y' | 'z'>
结合Exclude,我们可以介绍Omit的写作风格。
type Omit<T, K extends keyof any> = Pick<T, Exclude<keyof T, K>>;
interface User {
id: number;
age: number;
name: string;
};
// Equivalent to: type PickUser = { age: number; name: string; }
type OmitUser = Omit<User, "id">
5.typeof
顾名思义,typeof代表一个取一定值的类型,下面的例子展示了它们的用法
const a: number = 3
// Equivalent to: const b: number = 4
const b: typeof a = 4
在一个典型的服务器端项目中,我们经常需要将一些工具塞进上下文中,比如config、logger、db models、utils等,然后使用typeof。
import logger from './logger'
import utils from './utils'
interface Context extends KoaContect {
logger: typeof logger,
utils: typeof utils
}
app.use((ctx: Context) => {
ctx.logger.info('hello, world')
// will return an error because this method is not exposed in logger.ts, which minimizes spelling errors
ctx.loger.info('hello, world')
})
在此之前,我们先来看一个koa错误处理流程, 这是集中错误处理和识别代码的过程。
app.use(async (ctx, next) => {
try {
await next();
} catch (err) {
let code = 'BAD_REQUEST'
if (err.isAxiosError) {
code = `Axios-${err.code}`
} else if (err instanceof Sequelize.BaseError) {
}
ctx.body = {
code
}
}
})
在 err.code 中,它将编译错误,即“Error”.ts(2339) 类型上不存在属性“code”。
在这种情况下,可以使用 as AxiosError 或 as any 来避免错误,但是强制类型转换不够友好!
if ((err as AxiosError).isAxiosError) {
code = `Axios-${(err as AxiosError).code}`
}
在这种情况下,你可以使用 is 来确定值的类型。
function isAxiosError (error: any): error is AxiosError {
return error.isAxiosError
}
if (isAxiosError(err)) {
code = `Axios-${err.code}`
}
在 GraphQL 源代码中,有很多这样的用途来识别类型。
export function isType(type: any): type is GraphQLType;
export function isScalarType(type: any): type is GraphQLScalarType;
export function isObjectType(type: any): type is GraphQLObjectType;
export function isInterfaceType(type: any): type is GraphQLInterfaceType;
7. interface & type
interface 和 type有什么区别? 你可以参考这里:https://stackoverflow.com/questions/37233735/interfaces-vs-types-in-typescript
interface和type的区别很小,比如下面两种写法就差不多了。
interface A {
a: number;
b: number;
};
type B = {
a: number;
b: number;
}
interface可以如下合并,而type只能使用 & 类链接。
interface A {
a: number;
}
interface A {
b: number;
}
const a: A = {
a: 3,
b: 4
}
8. Record & Dictionary & Many
这些语法糖是从 lodash 的类型源代码中学习的,并且通常在工作场所中经常使用。
type Record<K extends keyof any, T> = {
[P in K]: T;
};
interface Dictionary<T> {
[index: string]: T;
};
interface NumericDictionary<T> {
[index: number]: T;
};
const data:Dictionary<number> = {
a: 3,
b: 4
}
9. 用 const enum 维护 const 表
Use objects to maintain constsconst TODO_STATUS { TODO: 'TODO', DONE: 'DONE', DOING: 'DOING'}
// Maintaining constants with const enumconst enum TODO_STATUS { TODO = 'TODO', DONE = 'DONE', DOING = 'DOING'}
function todos (status: TODO_STATUS): Todo[];
todos(TODO_STATUS.TODO)
10. VS Code 技巧和 Typescript 命令
有时候用 VS Code,用 tsc 编译时出现的问题与 VS Code 提示的问题不匹配。
在项目的右下角找到Typescript字样,版本号显示在右侧,你可以点击它并选择Use Workspace Version,表示它始终与项目所依赖的typescript版本相同。
或编辑 .vs-code/settings.json
{
"typescript.tsdk": "node_modules/typescript/lib"
}
总之,TypeScript 增加了代码的可读性和可维护性,让我们的开发更加优雅。
如果你觉得我今天的内容对你有用的话,请记得点赞我,关注我,并将这篇文章分享给你的朋友,也许能够帮助到他,最后,感谢你的阅读,编程愉快!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK