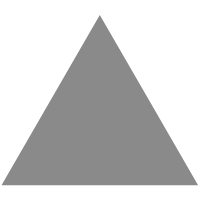
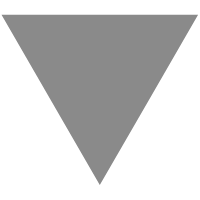
20 个超级有用的 JavaScript 技巧,让你的工作更轻松
source link: https://www.51cto.com/article/722216.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
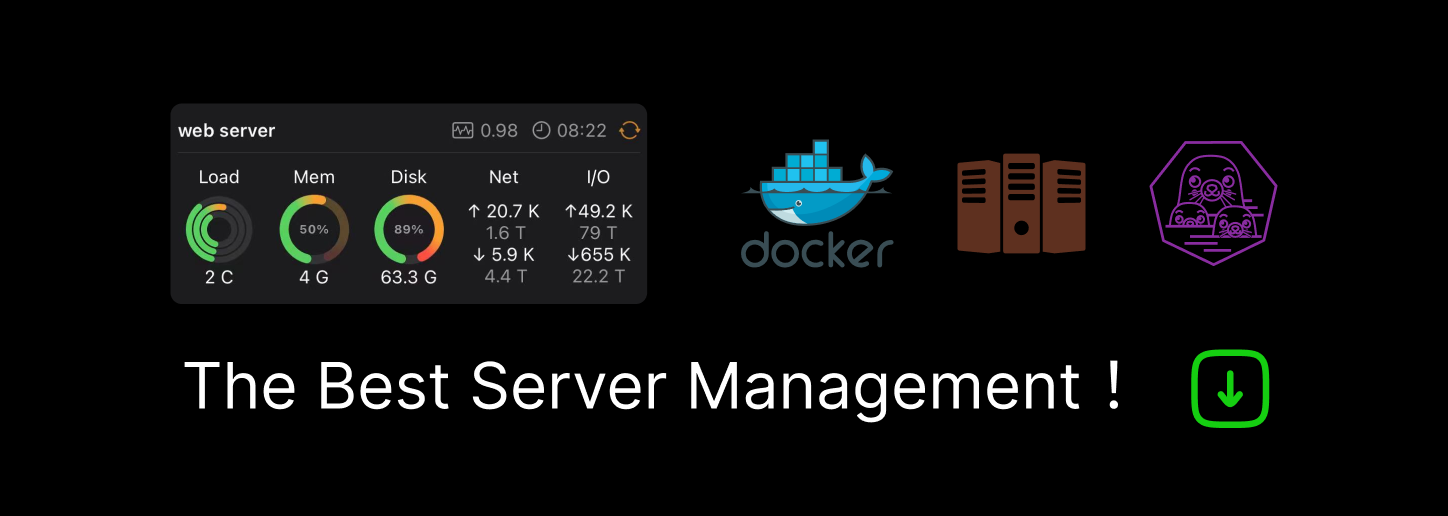
20 个超级有用的 JavaScript 技巧,让你的工作更轻松
今天这篇文章,我将跟大家分享21个我自己收藏使用的JavaScript技巧,希望今天这篇文章里的内容能够帮助到你,让你的工作更高效!更轻松!
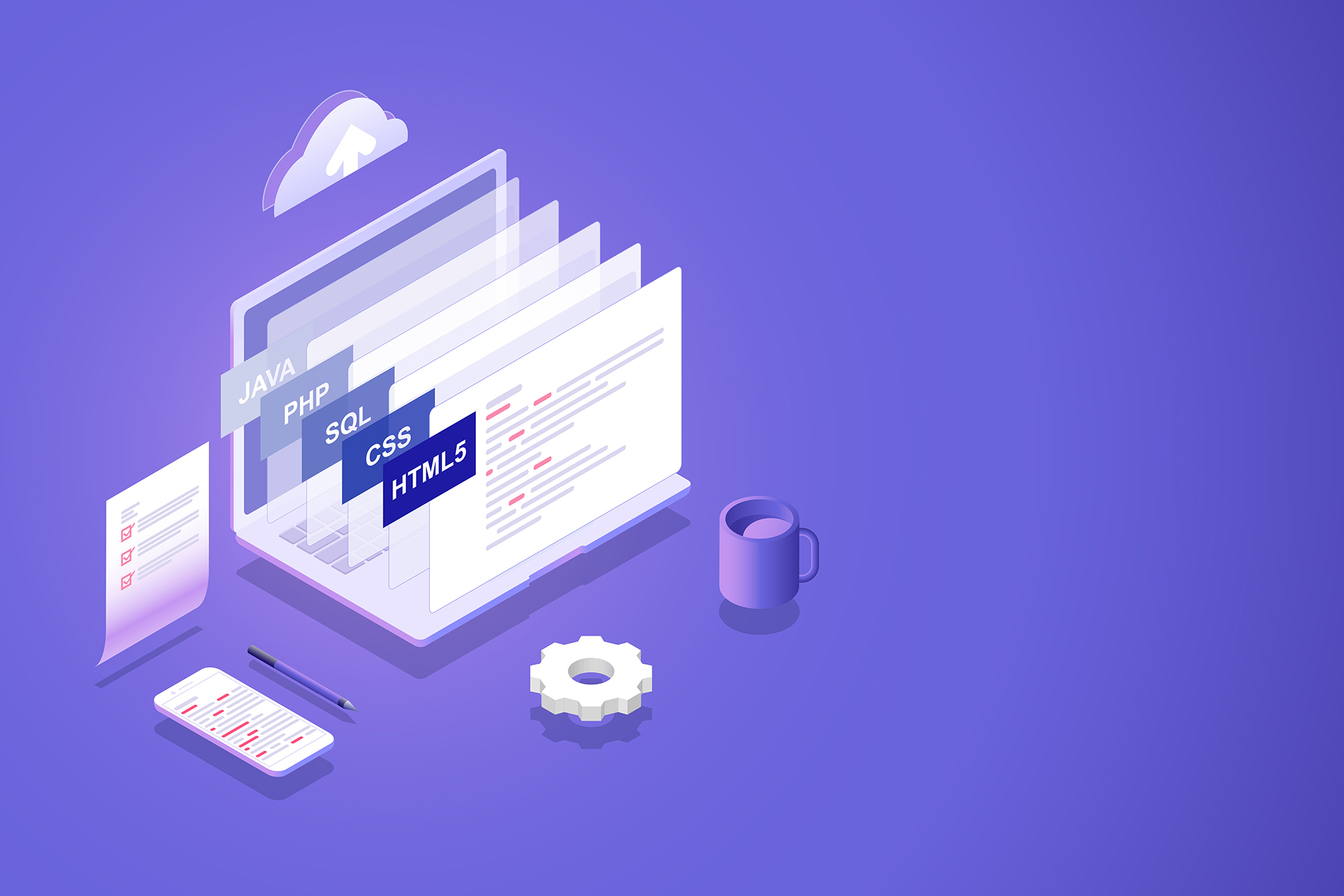
我们现在开始吧。
1. 多条件 if 语句
将多个值放入一个数组中,然后调用该数组的 include 方法。
// bad
if (x === "abc" || x === "def" || x === "ghi" || x === "jkl") {
//logic
}
// better
if (["abc", "def", "ghi", "jkl"].includes(x)) {
//logic
}
2. 简化 if true...else 条件表达式
// bad
let test: boolean;
if (x > 100) {
test = true;
} else {
test = false;
}
// better
let test = x > 10 ? true : false;
//or
let test = x > 10;
console.log(test);
3. 假值(undefined, null, 0, false, NaN, empty string)检查
当我们创建一个新变量时,有时我们想检查引用的变量是否是一个假值,例如 null 或 undefined 或空字符串。JavaScript 确实为这种检查提供了一个很好的快捷方式——逻辑 OR 运算符 (||)
|| 仅当左侧为空或 NaN 或 null 或 undefined 或 false 时,如果左侧操作数为假,则将返回右侧操作数,逻辑或运算符 (||) 将返回右侧的值。
// bad
if (test1 !== null || test1 !== undefined || test1 !== "") {
let test2 = test1;
}
// better
let test2 = test1 || "";
// bad
if (test1 === true) or if (test1 !== "") or if (test1 !== null)
// better
if (test1){
// do some
}else{
// do other
}
//Note: If test1 has a value, the logic after if will be executed.
//This operator is mainly used for null, undefined, and empty string checks.
4. null/undefined 检查和默认赋值
//null checking and default assignmentlet test1 = null;let test2 = test1 ?? "";
console.log("null check", test2); // output empty string ""
//undefined checking and default assignmentconst test = undefined ?? "default";console.log(test);// expected output: "default"
5. 获取列表中的最后一项
在其他语言中,此功能被制成可以在数组上调用的方法或函数,但在 JavaScript 中,你必须自己做一些工作。
let array = [0, 1, 2, 3, 4, 5, 6, 7];
console.log(array.slice(-1)) >>> [7];
console.log(array.slice(-2)) >>> [6, 7];
console.log(array.slice(-3)) >>> [5, 6, 7];
function lastItem(list) {
if (Array.isArray(list)) {
return list.slice(-1)[0];
}
if (list instanceof Set) {
return Array.from(list).slice(-1)[0];
}
if (list instanceof Map) {
return Array.from(list.values()).slice(-1)[0];
}
}
6.比较后返回
// bad
let test;
function checkReturn() {
if (!(test === undefined)) {
return test;
} else {
return callMe("test");
}
}
// better
function checkReturn() {
return test ?? callMe("test");
}
7. 使用可选的链接运算符 -?。
? 也称为链判断运算,它允许开发人员读取深度嵌套在对象链中的属性值,而无需验证每个引用,当引用为空时,表达式停止计算并返回 undefined。
const travelPlans = {
destination: "DC",
monday: {
location: "National Mall",
budget: 200,
},
};
// bad
const res = travelPlans && travelPlans.tuesday && travelPlans.tuesday.location && travelPlans.tuesday.location.href;
console.log(res); // Result: undefined
// better
const res1 = travelPlans?.tuesday?.location?.href;
console.log(res1); // Result: undefined
8. 多个条件的 && 运算符
要仅在变量为真时调用函数,请使用 && 运算符。
// bad
if (test) {
callMethod();
}
// better
test && callMethod();
当你想在 React 中有条件地渲染组件时,这对于使用 (&&) 进行短路很有用。例如:
<div> {this.state.isLoading && <Loading />} </div>
9.开关简化
我们可以将条件存储在键值对象中,并根据条件调用它们。
// bad
switch (data) {
case 1:
test1();
break;
case 2:
test2();
break;
case 3:
test();
break;
// And so on...
}
// better
var data = {
1: test1,
2: test2,
3: test,
};
// If type exists in data, execute the corresponding function
data[type] && data[type]();
10.默认参数值
// bad
function add(test1, test2) {
if (test1 === undefined) test1 = 1;
if (test2 === undefined) test2 = 2;
return test1 + test2;
}
// better
add = (test1 = 1, test2 = 2) => test1 + test2;
add(); //output: 3
11. 条件查找简化
如果我们想根据不同的类型调用不同的方法,我们可以使用多个 else if 语句或开关,但是还有比这更好的简化技巧吗?其实和之前的switch简化是一样的。
// bad
if (type === "test1") {
test1();
} else if (type === "test2") {
test2();
} else if (type === "test3") {
test3();
} else if (type === "test4") {
test4();
} else {
throw new Error("Invalid value " + type);
}
// better
var types = {
test1,
test2,
test3,
test4,
};
types[type] && types[type]();
12. 对象属性赋值
let test1 = "a";
let test2 = "b";
// bad
let obj = { test1: test1, test2: test2 };
// better
let obj = { test1, test2 };
13. 解构赋值
// bad
const test1 = this.data.test1;
const test2 = this.data.test2;
const test2 = this.data.test3;
// better
const { test1, test2, test3 } = this.data;
14. 模板字符串
如果你厌倦了使用 + 将多个变量连接成一个字符串,这个简化技巧会让你头疼。
// bad
const welcome = "Hi " + test1 + " " + test2 + ".";
// better
const welcome = `Hi ${test1} ${test2}`;
15. 跨越字符串
// bad
const data =
"hello maxwell this is a test\n\t" + "test test,test test test test\n\t";
// better
const data = `hello maxwell this is a test
test test,test test test test`;
16. indexOf的按位化简
在数组中查找某个值时,我们可以使用 indexOf() 方法。但是还有更好的方法,我们来看这个例子。
// bad
if (arr.indexOf(item) > -1) {
// item found
}
if (arr.indexOf(item) === -1) {
// item not found
}
// better
if (~arr.indexOf(item)) {
// item found
}
if (!~arr.indexOf(item)) {
// item not found
}
//The bitwise (~) operator will return true (except for -1),
//the reverse operation only requires !~. Alternatively, the includes() function can be used.
if (arr.includes(item)) {
// true if the item found
}
17. 将字符串转换为数字
有诸如 parseInt 和 parseFloat 等内置方法可用于将字符串转换为数字。我们也可以简单地通过在字符串前面提供一元运算符 (+) 来做到这一点。
// bad
let total = parseInt("583");
let average = parseFloat("32.5");
// better
let total = +"583";
let average = +"32.5";
18. 按顺序执行 Promise
如果你有一堆异步或普通函数都返回要求你一个一个执行它们的Promise怎么办?
async function getData() {
const promises = [fetch("url1"), fetch("url2"), fetch("url3"), fetch("url4")];
for (const item of promises) {
// Print out the promise
console.log(item);
}
// better
for await (const item of promises) {
// Print out the results of the request
console.log(item);
}
}
等待所有Promiae完成。
Promise.allSettled() 方法接受一组 Promise 实例作为参数,包装到一个新的 Promise 实例中。在所有这些参数实例都返回结果(已完成或已拒绝)之前,包装器实例不会结束。
有时候,我们并不关心异步请求的结果,我们只关心是否所有请求都结束了。这时候,Promise.allSettled() 方法就非常有用了。
const promises = [fetch("index.html"), fetch("https://does-not-exist/")];
const results = await Promise.allSettled(promises);
// Filter out successful requests
const successfulPromises = results.filter((p) => p.status === "fulfilled");
// Filter out failed requests and output the reason
const errors = results
.filter((p) => p.status === "rejected")
.map((p) => p.reason);
19.交换数组元素的位置
// bad
const swapWay = (arr, i, j) => {
const newArr = [...arr];
let temp = newArr[i];
newArr[i] = list[j];
newArr[j] = temp;
return newArr;
};
//Since ES6, swapping values from different locations in an array has become much easier
// better
const swapWay = (arr, i, j) => {
const newArr = [...arr];
const [newArr[j],newArr[i]] = [newArr[i],newArr[j]];
return newArr;
};
20. 带范围的随机数生成器
有时你需要生成随机数,但又希望数字在一定范围内,则可以使用此工具。
function randomNumber(max = 1, min = 0) {
if (min >= max) {
return max;
}
return Math.floor(Math.random() * (max - min) + min);
}
生成随机颜色
function getRandomColor() {
const colorAngle = Math.floor(Math.random() * 360);
return `hsla(${colorAngle},100%,50%,1)`;
}
到这里,我要跟你分享的20 个 JavaScript 的小技巧就结束了,希望这些小技巧对你有用。
在前面我也跟大家分享过很多这样的小技巧,不知道大家是否学会了没有?如果你没有学会的话,请记得多看看,暂时用不上的一些技巧,可以自行收藏起来。
同时,也要整理一份属于自己的使用技巧笔记。
如果你觉得我今天跟你分享的内容有帮助的话,请记得点赞我,关注我,并将其分享给你的开发者朋友,也许能够帮助到他。
以上就是我今天跟你分享的全部内容,感谢你的阅读,编程愉快!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK