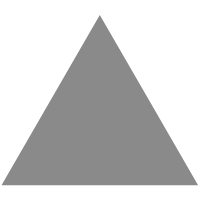
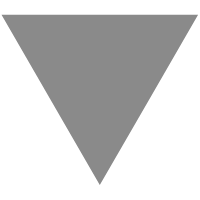
Rust与Java代码比较:将二维数组转为三维数组
source link: https://www.jdon.com/63175
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
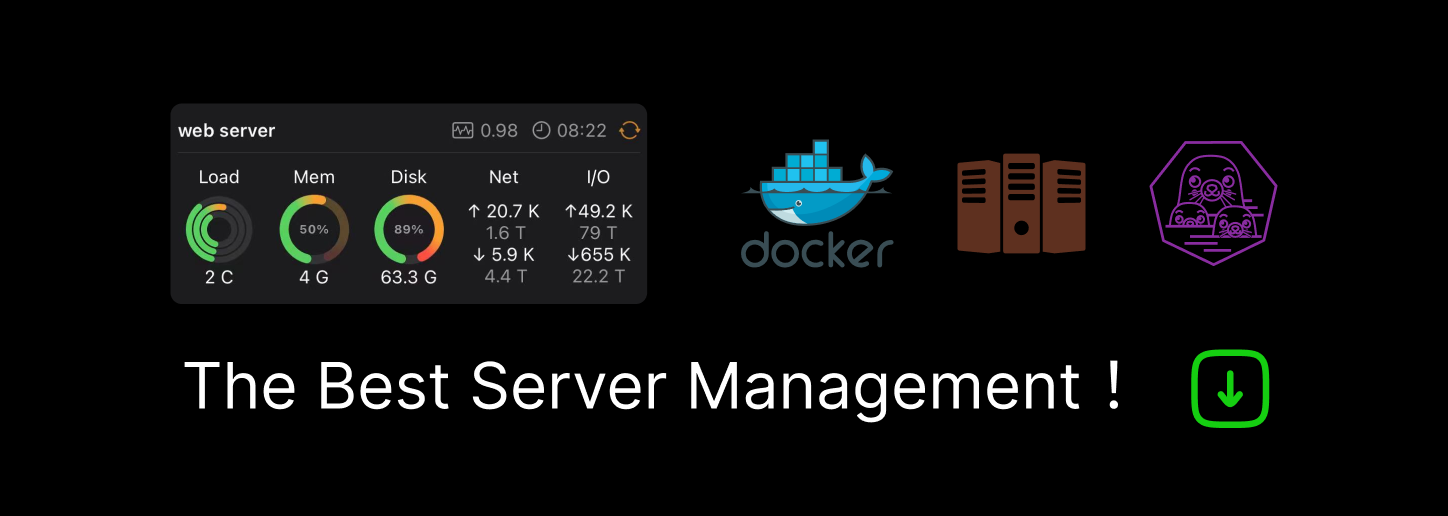
Rust与Java代码比较:将二维数组转为三维数组
在我们继续 Rust 之前,这是我可以用 Java 编写的简单代码(花不到五分钟的时间)
import java.util.Arrays; public class Demo { public static void update2dArray(int[][] array) { for (int i = 0; i < array.length; i++) { for (int j = 0; j < array[i].length; j++) { array[i][j] = 100; } } } public static void main(String[] args) { int[][] array = new int[10][10]; //by default, initial values = 0 System.out.println("Before change"); Arrays.stream(array).forEach(row -> Arrays.stream(row).forEach(item -> System.out.print(" " + item))); update2dArray(array); System.out.println("\nAfter change"); Arrays.stream(array).forEach(row -> Arrays.stream(row).forEach(item -> System.out.print(" " + item))); int[][] newArray = new int[20][20]; // size is 20x20 update2dArray(newArray); System.out.println("\nAfter change"); Arrays.stream(newArray).forEach(row -> Arrays.stream(row).forEach(item -> System.out.print(" " + item))); } } |
可以看到,在 Java 中,更新 2d 数组(或 3d 数组)自然很容易。你只需要创建一个函数,然后这个函数将一个二维数组作为参数。然后循环遍历数组的所有元素并更改每个元素的值。最后,当您返回主函数时,您将拥有一个更新的数组。
一个重要的注意事项是 update_2d 函数可以接收任何大小的二维数组。
作为一个内心深处有 Java的 开发人员,我想我会在 Rust 中做同样的事情,如下所示:
fn update_2d_array(arr: &mut [[u32; 10]; 10]) { for i in 0..arr.len() { for j in 0..arr[i].len() { arr[i][j] = 10; } } } fn main() { let mut array_2d = [[0;10]; 10]; println!("Before: {:?}", array_2d); update_2d_array(&mut array_2d); println!("After: {:?}", array_2d); } |
Rust 版本运行良好……但有一个问题,函数 update_2d_array 需要指定矩阵的宽度和高度……。
那么如何在运行时更新二维动态大小数组呢?
在谷歌上搜索了很多之后,我花了 3 个小时才找到答案。我们必须使用AsMut Trait,并创建一个新的数据类型V:AsMut<[u32]。您只需要记住 V 作为矩阵每一行的可变引用。
use std::array; fn update_2d_array(array: &mut [[u32; 10]; 10]) { for i in 0..array.len() { for j in 0..array[i].len() { array[i][j] = 100; } } } //AsMut trait fn update_2d_array_with_asmut<V: AsMut<[u32]>>(array: &mut [V]) { for i in 0..array.len() { for j in 0..array[i].as_mut().len() { array[i].as_mut()[j] = 200; } } } fn main() { let mut array_2d = [[0; 10]; 10]; // 10 x 10; println!("Before {:?}", array_2d); update_2d_array(&mut array_2d); println!("After {:?}", array_2d); update_2d_array_with_asmut(&mut array_2d); println!("After AsMut {:?}", array_2d); let mut array_2d_30 = [[0; 30]; 30]; update_2d_array_with_asmut(&mut array_2d_30); println!("After AsMut 30: {:?}", array_2d_30); } |
通过这样做,我只是想展示一个例子,学习 Rust 不像 Python 或 Java 等其他语言那样容易……要操作 2d/3d 数组,我们可以使用第三方 crate……
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK