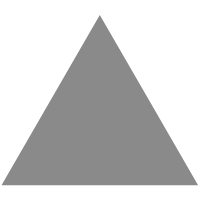
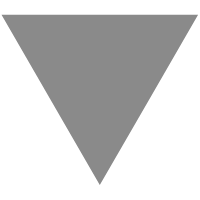
Why Javascript Developers are Poor Communicators
source link: https://confuzeus.com/shorts/javascript-poor-communicator/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
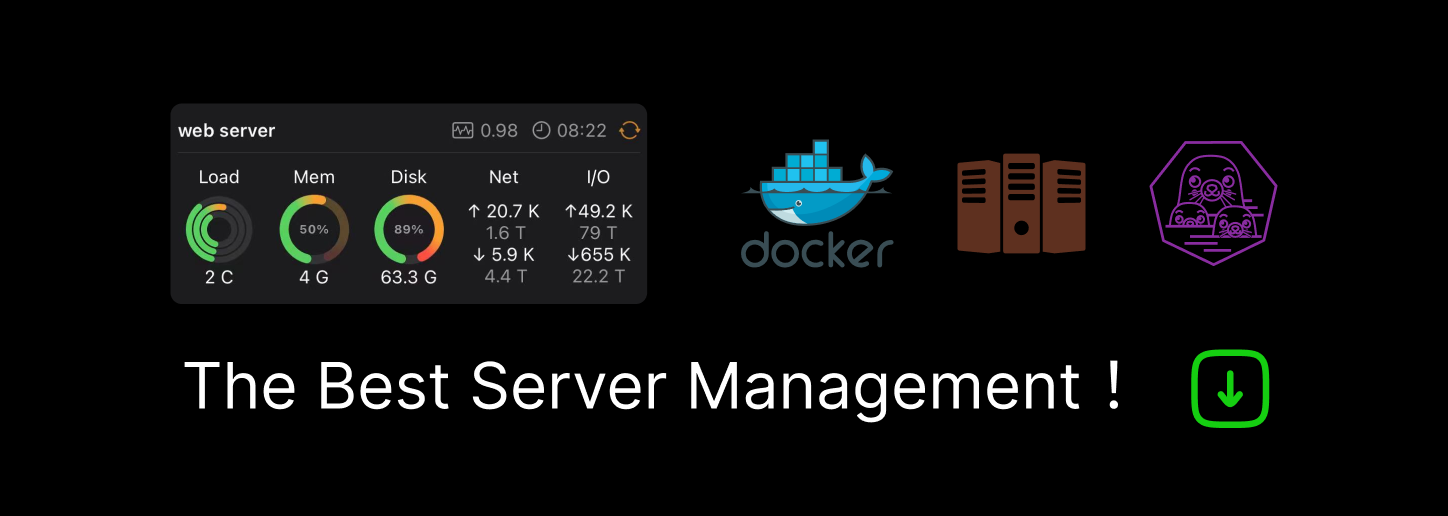
In this post, I’ll show you why 99% of Javascript code are less readable than they should be thanks to developers refusing to communicate their intention.
const the world
Before ES6, there was no way to declare constants so people used to do this:
var PI = 3.14;
Now when you get fired, your successor will read this code and figure out that PI should never be reassigned.
But as from ES6, everthing is a constant:
const pi = 3.14;
const x = 1;
const y = 2;
It’s impossible to know which variables are truly constants.
If I’m working on some code and want to reassign x, I need to go back and change
const
to var
, then I can reassign it because it’s not supposed to be a constant
in the first place.
I hear you say “Oh, but what if I accidentally reassign a variable on line 9000?”
Why exactly are you writing functions that are 9000 lines long?
There’s nothing that can prevent a junior developer from changing const to var in order to reassign a variable anymore because it’s now impossible to tell which variables are absolutely constant and which ones are not.
Let it be
Before ES6, there was only function scope so we had the following problem:
function doit(n, what) {
for (var i = 0; i < n; i++) {
what();
}
console.log(i);
}
At the end of the iteration, i will still be a valid definition.
That’s why ES6 introduced block scoping to allow developers to define variables that won’t leak outside of their enclosing blocks:
function doit(n, what) {
for (let i = 0; i < n; i++) {
what()
}
console.log(i); // ReferenceError
}
Since for loops define a new block, using let
allows you to define i and
have it get garbage collected at the end.
But these days everything is block scoped. Variables that have no business being block scoped are being block scoped.
Nobody wants to use var anymore because it’s old. If you use var during an interview, the interviewer will immediately throw your application in the trash.
Then how am I supposed to know that a particular variable was designed to be block scoped just by reading your code?
Let’s say you want to tell other developers that a variable but 100% be block scoped. You can’t and other developers will introduce scope related bugs later on thanks to this communication failure.
Never declare functions
Javascript has first class functions which means you can use a function anywhere a value is expected.
That’s why you can do the following:
const doit = (n, what) => {
...
}
Just pass a function to a variable definition and call it a day.
It’s much better than doing the following:
function doit(n, what) {
...
}
Right?
If you ask any JS developer why they prefer function expressions instead of declarations, they’ll answer with “Because”.
There’s simply no way to quickly go through a JS project anymore because of weird symbols and arrow function expressions everywhere.
Fearing this
Every function defines a keyword called this that points to the object where
this function is being called from. In foo.bar()
, the this keyword in the
bar function points to the object foo.
And yet, people can’t seem to wrap their heads around this so they go through extreme lengths to avoid having to deal with this at all.
One way to do that is by using arrow functions exclusively since those don’t define a this variable (aka lexical this):
var data = 'something';
var actions = {
data = 'stuff';
render: () => {
console.log(this.data); // something
}
}
Now if you use arrow functions everywhere, how am I supposed to know when you’re actually trying to access lexical this?
Clear communication
ES6 introduced a ton of cool features to make Javascript code more readable and easier to maintain.
A developer will use let and const in order to pretend to be superior than var peasants. Then he’s going to use arrow functions because the this keyword is evil.
No importance is given to communication. It’s easier to write your own frontend framework than contribute to an existing one because there’s no point in trying to read and understand the other framework’s code.
Next time you write JS code, think about the features you’re using and why so that your future self and other team members can have an easier time going through your code.
Email me if you want to argue.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK