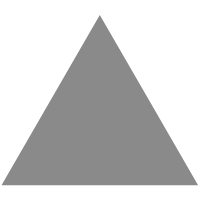
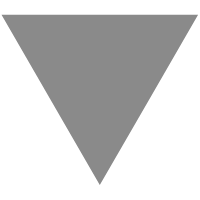
Data Binding in Angular9
source link: https://www.laravelcode.com/post/data-binding-in-angular9
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
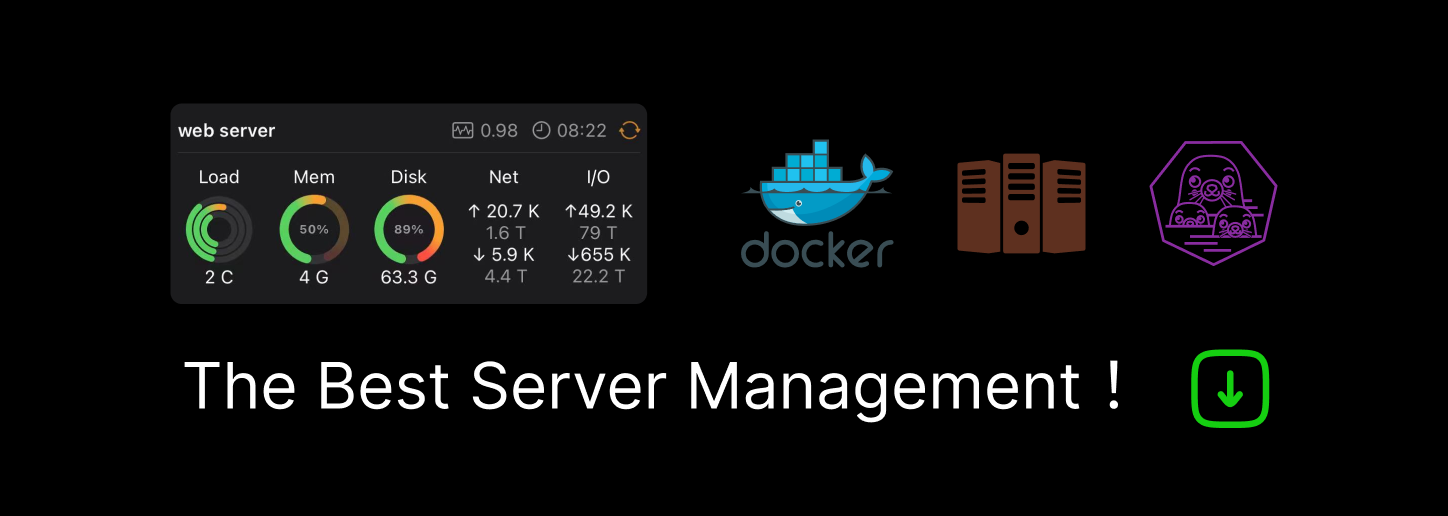
Data Binding in Angular9
In this Angular tutorial, we are going to shed light on data binding. We’ll learn how to bind data in an Angular web application using curly braces to show on the frontend.
How to use Interpolation in Angular 7|8|9 Data Binding?
Data Binding has been a part of AngularJS since Angular 7|8|9. In the code, you go for curly braces to denote data binding – {{ variable goes here }} and this process is referred to as interpolation.
The app.component.html
file has a variable named {{title}}. We initialize the value of this variable in theapp.component.ts
file. Later we display the value inapp.component.html
.
Our aim is to display the cars in a dropdown in the browser. To achieve the same, we are going to the code segment given below.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
// Title variable declared
title = 'My Angular App';
// Defined cars array
cars = ['Tesla', 'Toyota', 'BMW', 'Honda', 'Ford', 'Hyundai', 'Nissan', 'Porsche'];
}
We begin by creating a normal select tag with an option. We have taken the aid of the *ngFor loop in option. We are going to iterate through the array of the cars using this *ngFor loop. As a result, an option tag with the values in the drop-down will be created.
We will be using the following syntax in Angular – *ngFor = “let i for cars”. In order to fetch the value of cars, we are using the tag {{ i }}.
As stated before, we take the help of two curly brackets for data binding. You go to app.component.ts
file to declare the variables. Later you fetch the values with the help of curly brackets.
<div style="text-align:center">
<img width="250" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
<h1>
Welcome to {{ title }}!
</h1>
<div> Cars :
<select>
<option *ngFor="let i of cars">{{i}}</option>
</select>
</div>
</div>
Now we are going to take a look at the output of the above array of cars in the browser.
The app.component.ts
holds the variable and app.component.html
fetches the value of this variable with the aid of curly brackets – for instance, {{ }.
How to use If Statement using Angular 7|8|9 Data Binding?
Time has come for us to show the data in the browser on the basis of the condition. We have just added a variable and assigned ‘true’ as value to it. With the help of if statement, we are going to show/hide the data to be displayed.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
// isPresent var is declared
isPresent:boolean = true;
// Title variable declared
title = 'My Angular App';
// Defined cars array
cars = ['Tesla', 'Toyota', 'BMW', 'Honda', 'Ford', 'Hyundai', 'Nissan', 'Porsche'];
}
<div style="text-align:center">
<img width="250" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
<h1>
Welcome to {{ title }}!
</h1>
<div> Cars :
<select>
<option *ngFor="let i of cars">{{i}}</option>
</select>
</div>
<!-- Text will be displayed on the basis of the isPresent variable. -->
<div style="padding-top: 30px" *ngIf="isPresent">
<span>Statement is true.</span>
<p>Show me because isPresent is set to true.</p>
</div>
</div>
If then Else using Angular 7|8|9 Data Binding
Check out the Below Example
We are going to assign the value ‘false’ to the variable isPresent.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
// isPresent var is declared
isPresent:boolean = false;
// Title variable declared
title = 'My Angular App';
// Defined cars array
cars = ['Tesla', 'Toyota', 'BMW', 'Honda', 'Ford', 'Hyundai', 'Nissan', 'Porsche'];
}
In order to display the else condition, we are going to create ng-template as shown below
<div style="text-align:center">
<img width="250" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
<h1>
Welcome to {{ title }}!
</h1>
<div> Cars :
<select>
<option *ngFor="let i of cars">{{i}}</option>
</select>
</div>
<div style="padding-top: 30px">
<span *ngIf="isPresent; else condition1">Statement is true.</span>
<ng-template #condition1>NgTemplate condition is working!</ng-template>
</div>
</div>
Take a look at the below screenshot to see how it looks in the browser.
Another If then Else Example
We are going to assign true value to isPresent variable in the app.component.ts
file. We have written the condition as shown below –
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
// isPresent var is declared
isPresent:boolean = true;
}
If the variable has true value then condition1, else condition2. At this point, we have come up with 2 templates with ids #condition1 and #condition2.
<div style="text-align:center">
<img width="250" alt="Angular Logo" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHZpZXdCb3g9IjAgMCAyNTAgMjUwIj4KICAgIDxwYXRoIGZpbGw9IiNERDAwMzEiIGQ9Ik0xMjUgMzBMMzEuOSA2My4ybDE0LjIgMTIzLjFMMTI1IDIzMGw3OC45LTQzLjcgMTQuMi0xMjMuMXoiIC8+CiAgICA8cGF0aCBmaWxsPSIjQzMwMDJGIiBkPSJNMTI1IDMwdjIyLjItLjFWMjMwbDc4LjktNDMuNyAxNC4yLTEyMy4xTDEyNSAzMHoiIC8+CiAgICA8cGF0aCAgZmlsbD0iI0ZGRkZGRiIgZD0iTTEyNSA1Mi4xTDY2LjggMTgyLjZoMjEuN2wxMS43LTI5LjJoNDkuNGwxMS43IDI5LjJIMTgzTDEyNSA1Mi4xem0xNyA4My4zaC0zNGwxNy00MC45IDE3IDQwLjl6IiAvPgogIDwvc3ZnPg==">
<div style="padding-top: 30px">
<span *ngIf="isPresent; then condition1 else condition2">Statement is valid.</span>
<ng-template #condition1>Statement is valid</ng-template>
<ng-template #condition2>Statement is invalid</ng-template>
</div>
</div>
You can take a look at the display in the browser below –
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK