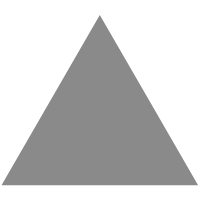
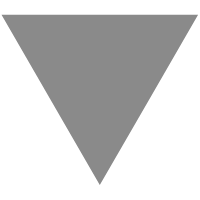
Controllers and Middleware in Express App
source link: https://codewithsudeep.com/sudeep/javascript/controllers-and-middleware-in-express-app/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
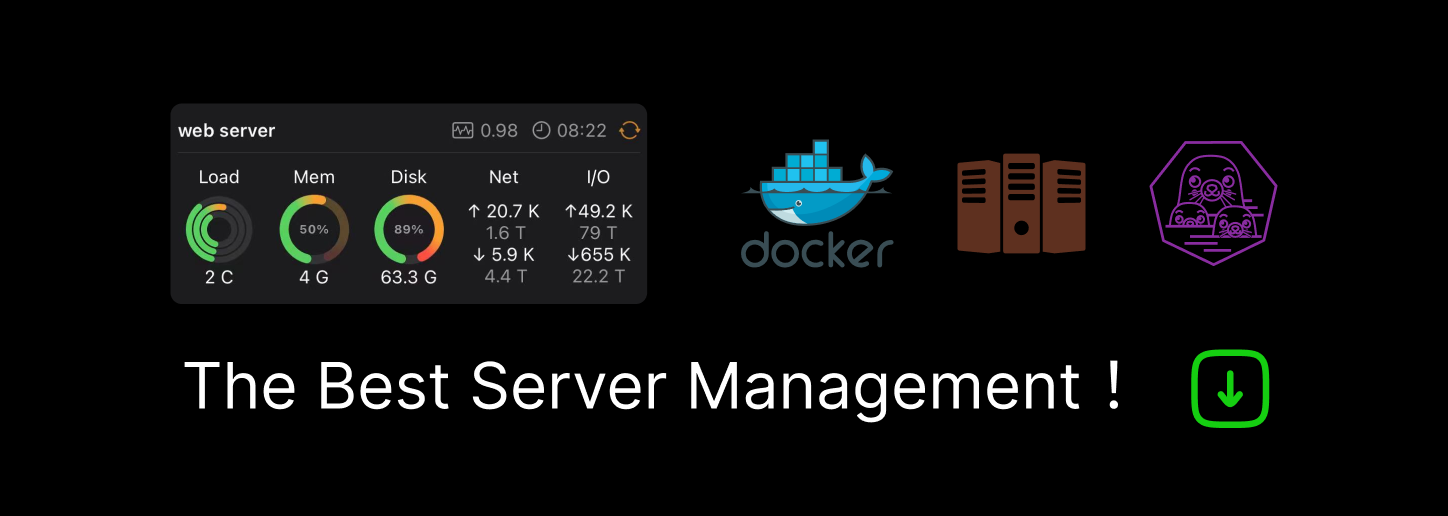
Controllers and Middleware in Express App
Read Previous: Routing in node js express app
Tips: To keep your express app running and restart whenever any file changes, install nodemon package npm install -g nodemon
and run nodemon test
Before starting with controllers and middleware, you must be familiar with a few concepts like js callbacks() [ See also: callback on Wikipedia]
Controllers are basically route handlers. some functions that are supposed to be executed for particular routes are defined in controllers.
Basic Route Handler
Let’s see an example of a function that is executed for the route path '/user'
// route handler function
const getUser=()=>{
console.log("route handler called for path '/user'");
}
// GET method route
app.get('/user', (req, res) => {
// handler function called
getUser();
})
Now, when you call this API, the output can be seen in the terminal where the server is running.

To send a response from the route handler, we can do it in this way,
const express = require('express')
const app = express()
// route handler function
const getUser=(request,response)=>{
response.send("+ Method: GET\n+ Route handler called for path '/user'");
}
// GET method route with route handle function as callback
app.get('/user', getUser)
app.listen(3000, () => {
console.log(`+ Express App Started\n+ Port:${3000}`)
})
Testing Route: [Thunder Client]

Controller File
For better understanding, we create a new file named userController.js
which will be a CJS module.

// userController.js
const getUser = (request,response) => {
const user = {
uid: "909989",
username: "cws",
}
response.status(200).json(user);
}
module.exports = { getUser }
Now in the main file which is index.js
, we have to import a method defined in userController.js
.
const express = require('express')
const app = express()
//import route handler from controller file
const {getUser} = require('./userController');
// GET method route
app.get('/user', getUser)
app.listen(3000, () => {
console.log(`+ Express App Started\n+ Port:${3000}`)
})
Middleware
Middleware is something that acts as a role between user request (routes) and response (route handler or say, controller).
in app.get('/path',callback1(),callback()2,....,finalCallback())
we can pass multiple methods for listening to any route or say as a route handler. These multiple methods just complete their job and pass the flow to the next callback function. except for the final callback which is responsible to send a response.
if none of your route handlers sends a response then the request will be failed
Let’s see a basic example of middleware,
const express = require('express')
const app = express()
const {getUser} = require('./userController');
// middleware function
const middlwareFunction = (request,response,next) => {
console.log('Middleware executed');
next()
}
// GET method route
app.get('/user',middlwareFunction, getUser)
app.listen(3000, () => {
console.log(`+ Express App Started\n+ Port:${3000}`)
})
For better understanding, let’s create a separate file for middleware which will be a CJS module.
It basically checks if userid parameter passed in the route is 111. if yes, then it passes the control to the next callback function passed as an argument in the route handler callback. otherwise, it sends a response with the text ‘no user found’.
// userMiddleware.js
const checkUserID = (request,response,next) =>{
const userid = request.params.userid;
if(userid==111){
return next()
}
response.send("No user found");
}
module.exports = { checkUserID }
Using Middleware
First, we import the middleware method from cjs module file in the index.js file. and pass it as the callback for route handling.
const express = require('express')
const app = express()
const {getUser} = require('./userController');
const { checkUserID } = require('./userMiddleware')
// GET method route
app.get('/user/:userid',checkUserID, getUser)
app.listen(3000, () => {
console.log(`+ Express App Started\n+ Port:${3000}`)
})
Testing:

User Detail Response

That is all you should know to work with route, controller, and middleware.
*** Combining Routes, MIddleware, and Controllers create an API.
Challenge 1: Create an API to add two numbers. Number Should be provided by using parameters in routes. Also, attach a middleware that checks both numbers if they are greater than 20.
Challenge 2: Create an API to log the request date & time for all routes.
Like this:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK