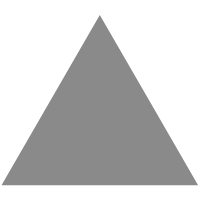
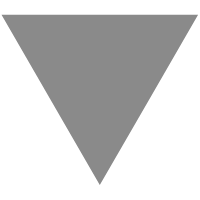
设计模式之策略模式_程序员田同学的技术博客_51CTO博客
source link: https://blog.51cto.com/u_15476035/5717966
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
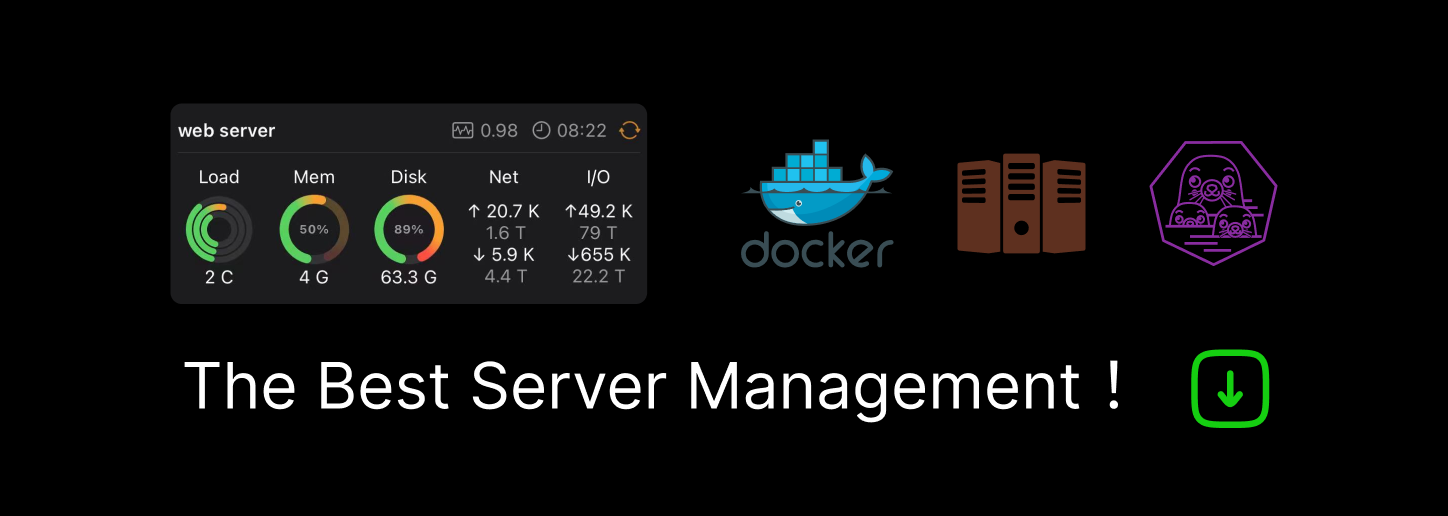
在一个收银系统中,如果普通用户、中级会员、高级会员分别对应着不同的优惠策略,常规编程就要使用一系列的判断语句,判断用户类型,这种情况下就可以使用策略模式。
一、概念理解
策略模式的概念很好理解,它将对象和行为分开,将行为定义为 一个行为接口和具体行为的实现,每个if判断都可以理解为一个策略。
如果在收银系统中使用策略模式,要将普通、中级、高级会员分别定义一个具体策略类,并实现各自的方法,定义一个环境类,持有策略类的引用,由引用调用相应的策略类方法,客户端传入相应的具体策略对象就会调用各自的策略方法。
学过了 状态模式,很多人也把状态模式和状态模式搞混,现在就可以考虑一下为什么不使用状态模式?
各个策略之间并不存在流转(比如:状态1234切换)关系,都是各自的算法实现各自的逻辑,客户端控制调用哪个策略,如果使用状态模式就变成了,先调用普通会员的策略,再调用中级会员的策略,再调用高级会员的策略,看到最后的优惠用户估计会乐疯吧!
和状态模式一样,策略模式也应包含三个角色:
抽象策略类:策略是一个接口,该接口定义若干个算法标识,即定义了若干个抽象方法
具体策略类:具体策略是实现策略接口的类
环境类 /上下文类:上下文提供一个方法,持有一个策略类的引用,最终给客户端调用。
相比于状态模式,策略模式各个角色的职责更简单,我们基于收银案例实现策略模式demo。
二、案例实现
抽象策略类:
定义业务抽象方法,我们主要是计算价格
/**
* 策略抽象类
* @author tcy
* @Date 21-09-2022
*/
public interface AbstractMemberStrategy {
// 一个计算价格的抽象方法
//price商品的价格 n商品的个数
public double calcPrice(double price, int n);
}
具体策略-高级会员:
各个具体策略实现各自的计算方法
/**高级会员
* @author tcy
* @Date 21-09-2022
*/
public class StrategyAdvanceMember implements AbstractMemberStrategy {
@Override
public double calcPrice(double price, int n) {
double money = price * n *0.8;
return money;
}
}
具体策略-中级会员:
/**
* 中级会员
* @author tcy
* @Date 21-09-2022
*/
public class StrategyIntermediateMember implements AbstractMemberStrategy {
@Override
public double calcPrice(double price, int n) {
double money = price * n*0.9;
return money;
}
}
具体策略-普通会员:
/**
* 初级会员
* @author tcy
* @Date 21-09-2022
*/
public class StrategyPrimaryMember implements AbstractMemberStrategy {
@Override
public double calcPrice(double price, int n) {
return price * n;
}
}
持有策略类的引用,调用时传入相应的具体策略对象,就会调用策略各自的方法。
/**环境类
* @author tcy
* @Date 21-09-2022
*/
public class Context {
// 用户折扣策略接口
private AbstractMemberStrategy memberStrategy;
// 注入构造方法
public Context(AbstractMemberStrategy memberStrategy) {
this.memberStrategy = memberStrategy;
}
// 计算价格
public double qoutePrice(double goodsPrice, int n){
// 通过接口变量调用对应的具体策略
return memberStrategy.calcPrice(goodsPrice, n);
}
}
客户端调用:
/**
* @author tcy
* @Date 21-09-2022
*/
public class Client {
public static void main(String[] args) {
// 具体策略类
AbstractMemberStrategy primaryMemberStrategy = new StrategyPrimaryMember();
AbstractMemberStrategy intermediateMemberStrategy = new StrategyIntermediateMember();
AbstractMemberStrategy advanceMemberStrategy = new StrategyAdvanceMember();
// 用户选择不同策略
Context primaryContext = new Context(primaryMemberStrategy);
Context intermediateContext = new Context(intermediateMemberStrategy);
Context advanceContext = new Context(advanceMemberStrategy);
//一本100块钱的书
// 普通会员:100
System.out.println("普通会员的价格:"+ primaryContext.qoutePrice(100,1));
// 中级会员 90
System.out.println("中级会员的价格:"+ intermediateContext.qoutePrice(100,1));
// 高级会员 80
System.out.println("高级会员的价格:"+ advanceContext.qoutePrice(100,1));
}
}
策略模式相对于状态模式理解起来更没有任何难度。
三、Jdk中的应用
策略模式的典型应用是Jdk中线程池满之后的拒绝策略,我们在创建一个线程池时会传入以下参数:
public ThreadPoolExecutor(int corePoolSize,int maximumPoolSize,long keepAliveTime,
TimeUnit unit,BlockingQueue<Runnable> workQueue,
ThreadFactory threadFactory,RejectedExecutionHandler handler) {
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
其中 RejectedExecutionHandler 是线程池满之后的拒绝策略,jdk中的多线程内置了四种拒绝策略,如下图:

①ThreadPoolExecutor.AbortPolicy 默认拒绝策略,拒绝任务并抛出任务
②ThreadPoolExecutor.CallerRunsPolicy 使用调用线程直接运行任务
③ThreadPoolExecutor.DiscardPolicy 直接拒绝任务,不抛出错误
④ThreadPoolExecutor.DiscardOldestPolicy 触发拒绝策略,只要还有任务新增,一直会丢弃阻塞队列的最老的任务,并将新的任务加入
这四种拒绝策略就代表策略模式角色中的具体策略角色,ThreadPoolExecutor类我们可以将其看做环境类。
我们知道执行多线程中的方法是schedule方法,可以就认为这就是角色中的客户端:
public ScheduledFuture<?> schedule(Runnable command,
long delay,
TimeUnit unit) {
if (command == null || unit == null)
throw new NullPointerException();
RunnableScheduledFuture<?> t = decorateTask(command,
new ScheduledFutureTask<Void>(command, null,
triggerTime(delay, unit)));
delayedExecute(t);
return t;
}
既然是客户端,就会有时机调用拒绝策略方法,我们点进去看delayedExecute()方法。
private void delayedExecute(RunnableScheduledFuture<?> task) {
if (isShutdown())
reject(task);
else {
super.getQueue().add(task);
if (isShutdown() &&
!canRunInCurrentRunState(task.isPeriodic()) &&
remove(task))
task.cancel(false);
else
ensurePrestart();
}
}
接着看reject()方法,该方法时机上调用的就是拒绝策略方法。传入相应的this对象,调用不同的拒绝策略。
final void reject(Runnable command) {
handler.rejectedExecution(command, this);
}
调用时序如下图:

何时调用何种拒绝策略,由delayedExecute()方法自己来决定,各个拒绝策略有各自的业务逻辑,这就是策略模式的典型应用。
四、策略模式和状态模式区别
策略模式和状态模式虽然类图一模一样,很多博客也将他们混为一谈,实际上策略模式和状态模式没有半毛钱的关系,只有理解了两种模式的使用场景,在运用时才能游刃有余,以下为我总结的四点不同之处,状态模式的博客可以参考 状态模式。
①策略模式中的各策略相互之间没有什么关系,比如支付方式选择、优惠策略选择;状态模式往往是一套流程,比如订单状态流转、请假流程审批等。
②在策略模式下,调用哪个策略由客户端决定;状态模式中,客户端只管调用,各个具体状态类定义切换下一状态。
③状态模式强调状态变化、策略模式强调的是策略的选择。
使用策略模式会让我们的代码更加的“干净”,但是如果实际的if判断中的逻辑很简单,我们仍然使用策略模式,就变成了为了使用设计模式而使用,这无疑加重系统的复杂程度。
就像商城系统中,微信支付、支付宝支付、银联支付,业务逻辑没那么简单的,使用策略模式就是一个好的选择。
整体来说策略模式在行为型模式中还属于一种比较简单的模式,无论是理解起来还是写起来都属极简单,难度堪比结构型设计模式中的 单例模式。
设计模式的学习要成体系,推荐你看我往期发布的设计模式文章。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK