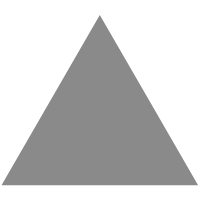
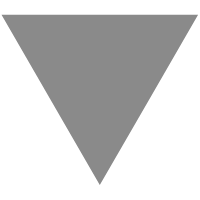
CString in alloc::ffi - Rust
source link: https://doc.rust-lang.org/stable/alloc/ffi/struct.CString.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
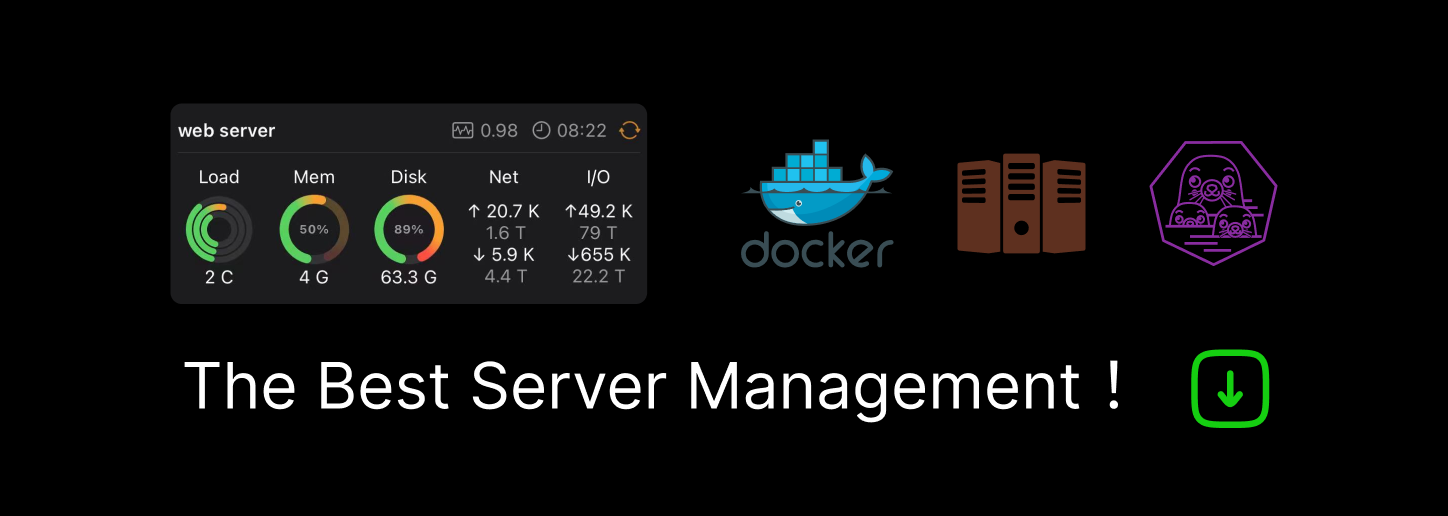
Implementations
Creates a new C-compatible string from a container of bytes.
This function will consume the provided data and use the underlying bytes to construct a new string, ensuring that there is a trailing 0 byte. This trailing 0 byte will be appended by this function; the provided data should not contain any 0 bytes in it.
Examples
use std::ffi::CString;
use std::os::raw::c_char;
extern "C" { fn puts(s: *const c_char); }
let to_print = CString::new("Hello!").expect("CString::new failed");
unsafe {
puts(to_print.as_ptr());
}
RunErrors
This function will return an error if the supplied bytes contain an
internal 0 byte. The NulError
returned will contain the bytes as well as
the position of the nul byte.
Creates a C-compatible string by consuming a byte vector, without checking for interior 0 bytes.
Trailing 0 byte will be appended by this function.
This method is equivalent to CString::new
except that no runtime
assertion is made that v
contains no 0 bytes, and it requires an
actual byte vector, not anything that can be converted to one with Into.
Examples
use std::ffi::CString;
let raw = b"foo".to_vec();
unsafe {
let c_string = CString::from_vec_unchecked(raw);
}
RunRetakes ownership of a CString
that was transferred to C via
CString::into_raw
.
Additionally, the length of the string will be recalculated from the pointer.
Safety
This should only ever be called with a pointer that was earlier
obtained by calling CString::into_raw
. Other usage (e.g., trying to take
ownership of a string that was allocated by foreign code) is likely to lead
to undefined behavior or allocator corruption.
It should be noted that the length isn’t just “recomputed,” but that
the recomputed length must match the original length from the
CString::into_raw
call. This means the CString::into_raw
/from_raw
methods should not be used when passing the string to C functions that can
modify the string’s length.
Note: If you need to borrow a string that was allocated by foreign code, use
CStr
. If you need to take ownership of a string that was allocated by foreign code, you will need to make your own provisions for freeing it appropriately, likely with the foreign code’s API to do that.
Examples
Creates a CString
, pass ownership to an extern
function (via raw pointer), then retake
ownership with from_raw
:
use std::ffi::CString;
use std::os::raw::c_char;
extern "C" {
fn some_extern_function(s: *mut c_char);
}
let c_string = CString::new("Hello!").expect("CString::new failed");
let raw = c_string.into_raw();
unsafe {
some_extern_function(raw);
let c_string = CString::from_raw(raw);
}
RunConsumes the CString
and transfers ownership of the string to a C caller.
The pointer which this function returns must be returned to Rust and reconstituted using
CString::from_raw
to be properly deallocated. Specifically, one
should not use the standard C free()
function to deallocate
this string.
Failure to call CString::from_raw
will lead to a memory leak.
The C side must not modify the length of the string (by writing a
null
somewhere inside the string or removing the final one) before
it makes it back into Rust using CString::from_raw
. See the safety section
in CString::from_raw
.
Examples
use std::ffi::CString;
let c_string = CString::new("foo").expect("CString::new failed");
let ptr = c_string.into_raw();
unsafe {
assert_eq!(b'f', *ptr as u8);
assert_eq!(b'o', *ptr.offset(1) as u8);
assert_eq!(b'o', *ptr.offset(2) as u8);
assert_eq!(b'\0', *ptr.offset(3) as u8);
// retake pointer to free memory
let _ = CString::from_raw(ptr);
}
RunConverts the CString
into a String
if it contains valid UTF-8 data.
On failure, ownership of the original CString
is returned.
Examples
use std::ffi::CString;
let valid_utf8 = vec![b'f', b'o', b'o'];
let cstring = CString::new(valid_utf8).expect("CString::new failed");
assert_eq!(cstring.into_string().expect("into_string() call failed"), "foo");
let invalid_utf8 = vec![b'f', 0xff, b'o', b'o'];
let cstring = CString::new(invalid_utf8).expect("CString::new failed");
let err = cstring.into_string().err().expect("into_string().err() failed");
assert_eq!(err.utf8_error().valid_up_to(), 1);
RunConsumes the CString
and returns the underlying byte buffer.
The returned buffer does not contain the trailing nul terminator, and it is guaranteed to not have any interior nul bytes.
Examples
use std::ffi::CString;
let c_string = CString::new("foo").expect("CString::new failed");
let bytes = c_string.into_bytes();
assert_eq!(bytes, vec![b'f', b'o', b'o']);
RunEquivalent to CString::into_bytes()
except that the
returned vector includes the trailing nul terminator.
Examples
use std::ffi::CString;
let c_string = CString::new("foo").expect("CString::new failed");
let bytes = c_string.into_bytes_with_nul();
assert_eq!(bytes, vec![b'f', b'o', b'o', b'\0']);
Run1.0.0 · source
pub fn as_bytes(&self) -> &[u8]
Returns the contents of this CString
as a slice of bytes.
The returned slice does not contain the trailing nul
terminator, and it is guaranteed to not have any interior nul
bytes. If you need the nul terminator, use
CString::as_bytes_with_nul
instead.
Examples
use std::ffi::CString;
let c_string = CString::new("foo").expect("CString::new failed");
let bytes = c_string.as_bytes();
assert_eq!(bytes, &[b'f', b'o', b'o']);
RunEquivalent to CString::as_bytes()
except that the
returned slice includes the trailing nul terminator.
Examples
use std::ffi::CString;
let c_string = CString::new("foo").expect("CString::new failed");
let bytes = c_string.as_bytes_with_nul();
assert_eq!(bytes, &[b'f', b'o', b'o', b'\0']);
Run1.20.0 · source
pub fn as_c_str(&self) -> &CStr
Extracts a CStr
slice containing the entire string.
Examples
use std::ffi::{CString, CStr};
let c_string = CString::new(b"foo".to_vec()).expect("CString::new failed");
let cstr = c_string.as_c_str();
assert_eq!(cstr,
CStr::from_bytes_with_nul(b"foo\0").expect("CStr::from_bytes_with_nul failed"));
RunConverts this CString
into a boxed CStr
.
Examples
use std::ffi::{CString, CStr};
let c_string = CString::new(b"foo".to_vec()).expect("CString::new failed");
let boxed = c_string.into_boxed_c_str();
assert_eq!(&*boxed,
CStr::from_bytes_with_nul(b"foo\0").expect("CStr::from_bytes_with_nul failed"));
RunConverts a Vec<u8>
to a CString
without checking the
invariants on the given Vec
.
Safety
The given Vec
must have one nul byte as its last element.
This means it cannot be empty nor have any other nul byte anywhere else.
Example
use std::ffi::CString;
assert_eq!(
unsafe { CString::from_vec_with_nul_unchecked(b"abc\0".to_vec()) },
unsafe { CString::from_vec_unchecked(b"abc".to_vec()) }
);
RunAttempts to converts a Vec<u8>
to a CString
.
Runtime checks are present to ensure there is only one nul byte in the
Vec
, its last element.
Errors
If a nul byte is present and not the last element or no nul bytes is present, an error will be returned.
Examples
A successful conversion will produce the same result as CString::new
when called without the ending nul byte.
use std::ffi::CString;
assert_eq!(
CString::from_vec_with_nul(b"abc\0".to_vec())
.expect("CString::from_vec_with_nul failed"),
CString::new(b"abc".to_vec()).expect("CString::new failed")
);
RunAn incorrectly formatted Vec
will produce an error.
use std::ffi::{CString, FromVecWithNulError};
// Interior nul byte
let _: FromVecWithNulError = CString::from_vec_with_nul(b"a\0bc".to_vec()).unwrap_err();
// No nul byte
let _: FromVecWithNulError = CString::from_vec_with_nul(b"abc".to_vec()).unwrap_err();
RunRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK