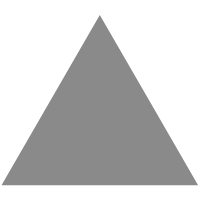
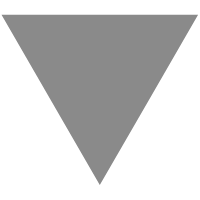
Vue 3 Reactivity Breakdown
source link: https://vue3-reactivity-breakdown.vercel.app/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
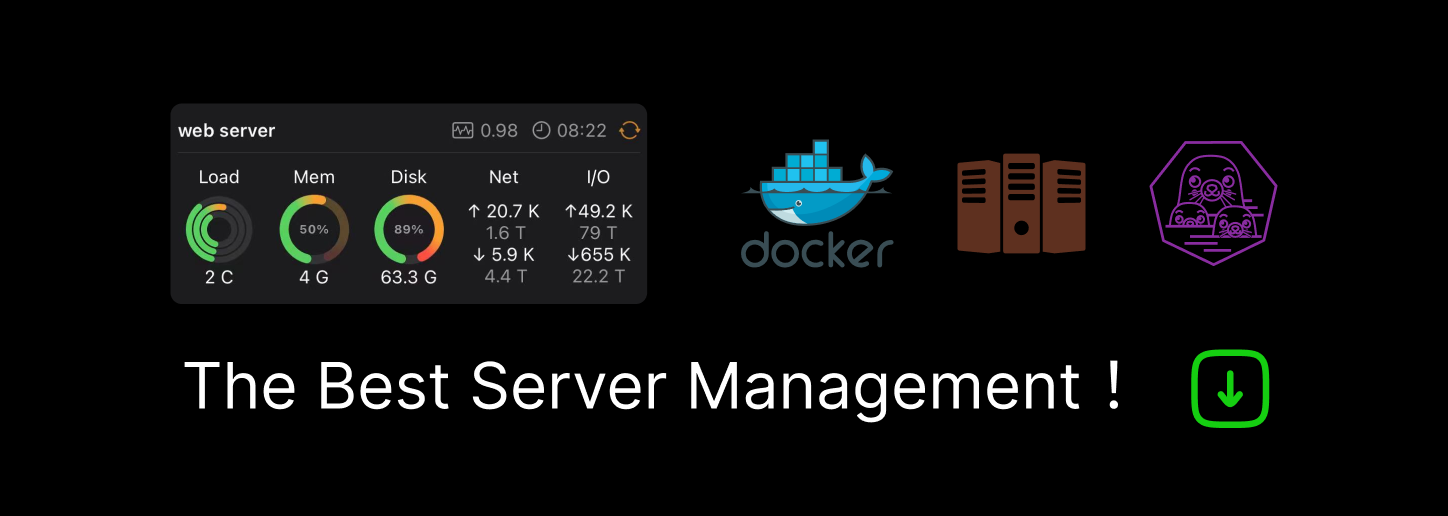
Vue 3 Reactivity Breakdown
Breaking down the power of the Vue 3 Reactivity API using TypeScript and script setup.
Built by @rbluethl, code on GitHub.
ref()
Use `ref`
to wrap any value into a reactive object. In this example, we keep track of a numeric value that we can decrement or increment.
Count is 0
Example
<script setup lang="ts"> const count = ref(0) </script>
<template> <span>Count is {{ count }}</span> <button type="button" @click="count++">Increment</button> </template>
reactive()
Use `reactive`
to return a reactive proxy of an object. In this example, we keep track of the inner numeric count that we can decrement or increment. The reactive object itself cannot be reassigned.
Count is 0
Example
<script setup lang="ts"> const state = reactive({ count: 0 }) </script>
<template> <span>Count is {{ state.count }}</span> <button type="button" @click="state.count++">Increment</button> </template>
computed()
Use `computed`
to compute a readonly reactive value. In this example, we compute the double of the value.
Count is 0
Double is 0
Example
<script setup lang="ts"> const count = ref(0) const double = computed(() => count.value * 2) </script>
<template> <span>Count is {{ count }}</span> <span>Double is {{ double }}</span> <button type="button" @click="count++">Increment</button> </template>
watch()
Use `watch`
to watch a reactive data source and call a function whenever the value changes. In this example, we store the previous value whenever the value changes.
Count is 0
Previous is uncertain
Example
<script setup lang="ts"> const count = ref(0) const previous = ref<number | null>(null) watch(count, (_val, oldVal) => { // Set previous value whenever count changes previous.value = oldVal }) </script>
<template> <span>Count is {{ count }}</span> <span>Previous is {{ previous ?? 'uncertain' }}</span> <button type="button" @click="count++">Increment</button> </template>
watchEffect()
Use `watchEffect`
to run a function whenever the value of a dependency changes. In this example, we display an alert whenever the value divided by 5 is 0. Note that the alert is displayed before the updated value is display in the UI. This is because of the default flush: pre
setting of watchEffect.
Count is 0
Example
<script setup lang="ts"> const count = ref(0) watchEffect(() => { if (count.value !== 0 && count.value % 5 === 0) { alert(`Count is ${count.value}`) } }) </script>
<template> <span>Count is {{ count }}</span> <button type="button" @click="count++">Increment</button> </template>
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK