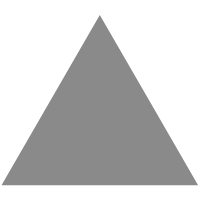
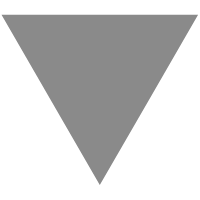
Module system in Node.js (CommonJS)
source link: https://medium.com/@dcortes.net/module-system-in-node-js-commonjs-c89f33257062
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
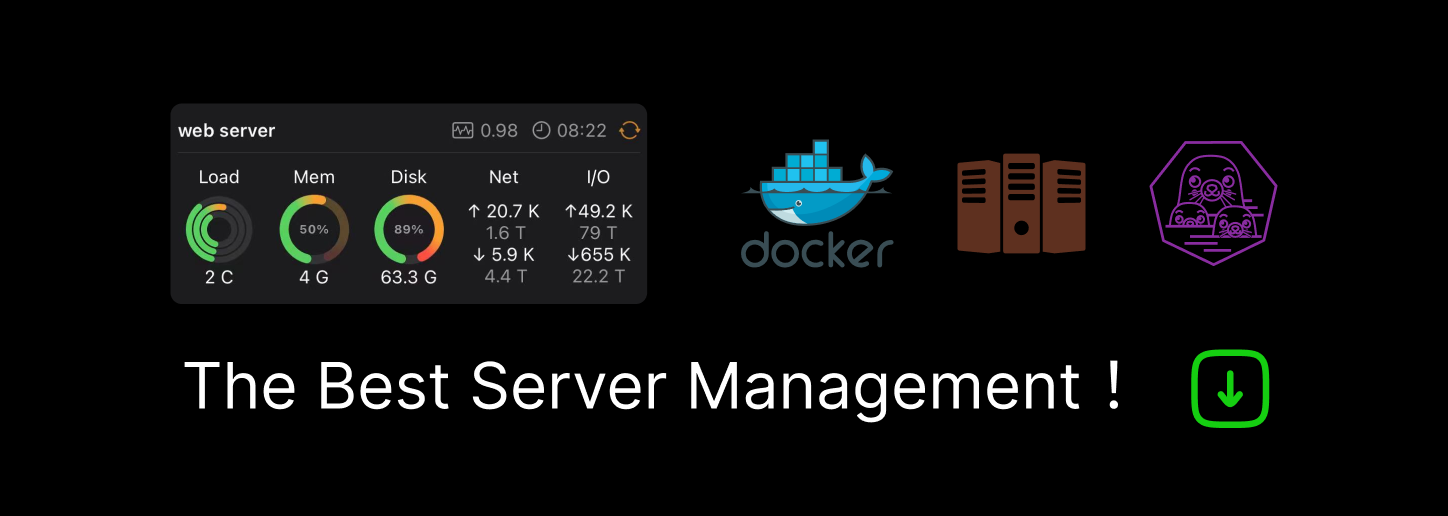
Module system in Node.js (CommonJS)

Basic article on handling modules in Nodejs. CommonJS system, object module, require and export functions.
CommonJS
CommonJS was born in 2009 as a project to establish conventions in the ecosystem of modules for Javascript outside the browser. Noting that back then, Javascript lacked common standardized ways for reusing pieces of software in environments other than the web browser. This is why a few years later the Nodejs team implemented CommonJS in all incoming versions to standardize server-side development.
Emphasizing that the module system in Javascript is different depending on the execution environment (client or server). Another module system called ES modules is used as standard in the browser and all modern client-side frameworks use it to build their applications.
NOTE: This article will only cover the server-side system.
Object module
It is a native Nodejs object that logs all references to the current modules in our application. This includes the reference to node_modules, where functionalities can be obtained just by performing the respective imports.
Here’s an inspection of the module object:
console.log(module);
This prints the following output to the console:
Module {
id: '.',
path: 'C:\\Users\\Admin\\Documents\\TEST_AREA_JS',
exports: {},
parent: null,
filename: 'C:\\Users\\Admin\\Documents\\TEST_AREA_JS\\foo.js',
loaded: false,
children: [],
paths: [
'C:\\Users\\Admin\\Documents\\TEST_AREA_JS\\node_modules',
'C:\\Users\\Admin\\Documents\\node_modules',
'C:\\Users\\Admin\\node_modules',
'C:\\Users\\node_modules',
'C:\\node_modules'
]
}
Export
The export statement is a property of the module object that is used to bind the current file reference. Thanks to the link we will have at our disposal functions, objects, or variables of the current context so that they can be used by other programs.
Here is an example of exporting 2 functionalities from a created file called math.js:
const sum = (a,b) => {
return a + b;
}const sub = (a,b) => {
return a - b;
}module.exports = {
sum,
sub
}
If we inspect the module object again we will get the following result:
Module {
id: '.',
path: 'C:\\Users\\Admin\\Documents\\TEST_AREA_JS',
exports: { sum: [Function: sum], sub: [Function: sub] },
parent: null,
filename: 'C:\\Users\\Admin\\Documents\\TEST_AREA_JS\\math.js',
loaded: false,
children: [],
paths: [
'C:\\Users\\Admin\\Documents\\TEST_AREA_JS\\node_modules',
'C:\\Users\\Admin\\Documents\\node_modules',
'C:\\Users\\Admin\\node_modules',
'C:\\Users\\node_modules',
'C:\\node_modules'
]
}
Highlighting the export property is modified by taking into account the following:
exports: { sum: [Function: sum], sub: [Function: sub] },
Require
Once the module has been exported, we must now use it, for this case we have the require statement, which is basically a function that obtains what is sought in the module object references.
Here is an example of importing the 2 previous functionalities from the created file called math.js:
const math = require('./math');console.log(math.sum(1,2));
console.log(math.sub(2,1));
This prints the following output to the console:
3
1
The require function is one of the most important functions in node, since with this function we can obtain core modules, third-party modules, and our own files.
Here is an example of some initial lines of a node express application that uses require to get the necessary functionality to work from node_modules.
const express = require('express');
const morgan = require('morgan');
const path = require('path');const DEFAULT_PORT = process.env.PORT || 6420;....
Resources Nodejs module system
- Nodejs API Module: Official documentation, examples, and tips.
Thanks for coming this far, if you find this useful don’t forget to clap 👏 and share 😀. Subscribe to receive more content 🔔.
If you need additional help, please contact me 🤠.
Thank you very much for reading, I appreciate your time.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK