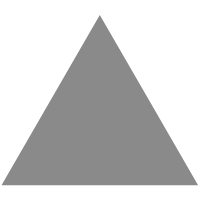
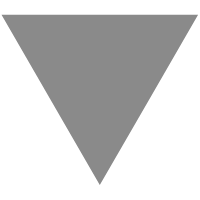
Power of Four and Reverse Vowels of a String
source link: https://hackernoon.com/power-of-four-and-reverse-vowels-of-a-string
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
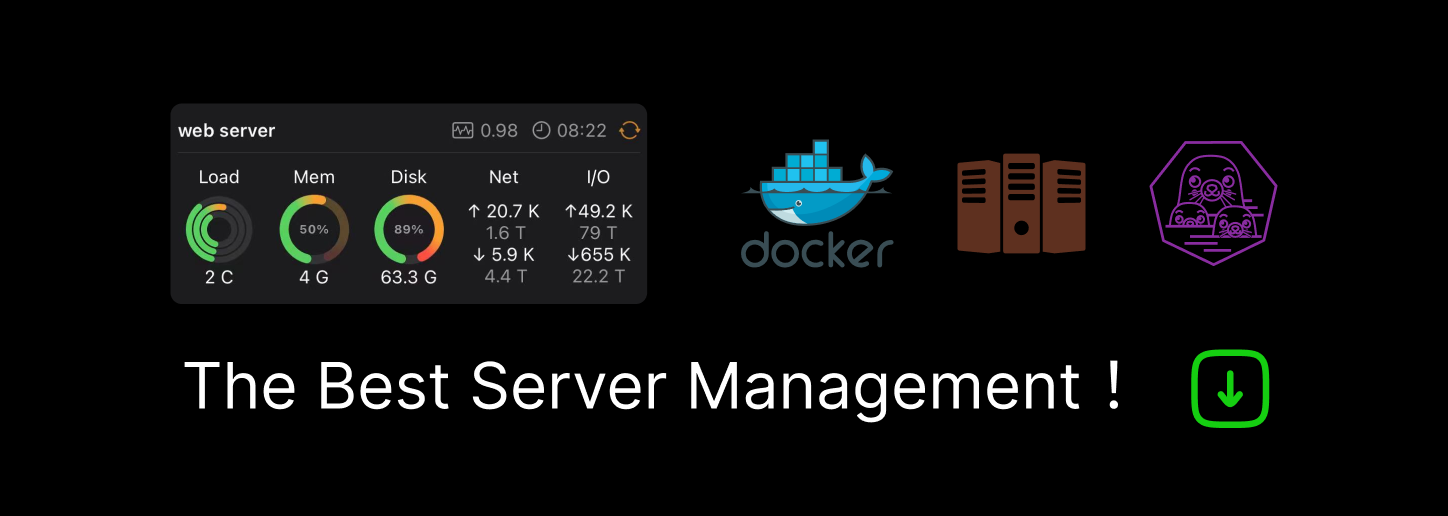
Power of Four and Reverse Vowels of a String
Power of Four and Reverse Vowels of a String

audio
element.Senior Software Engineer with 7+ YoE building massively scalable systems both from scratch and diving into a codebase
Credibility
-
Given an integer
n
, returntrue
if it is a power of four. Otherwise, returnfalse
. -
An integer
n
is a power of four, if there exists an integerx
such thatn == 4x
.
Example 1:
Input: n = 16
Output: true
Example 2:
Input: n = 5
Output: false
Example 3:
Input: n = 1
Output: true
Solution:
The easiest way how to solve this problem is to divide N on 4 and that is it. We will iterate again and again till our number is bigger than 1.
public boolean isPowerOfFour(int n) {
if (n == 0){
return false;
}
while(n != 1){
if (n % 4 != 0){
return false;
}
n /= 4;
}
return true;
}
But also we can use recursion:
public boolean isPowerOfFour(int n) {
return check(n, 1);
}
boolean check(int n, long value) {
if (value >= n) return n == value;
return check(n, value * 4);
}
And sure, we can solve it with math, but I don’t want to share it with you. Because for me, you usually cannot find a mathematical solution without solving this problem before.
Does it clear? Write your comments.
ok ok and out next problem for today
Reverse Vowels of a String
- Given a string
s
, reverse only all the vowels in the string and return it. - The vowels are
'a'
,'e'
,'i'
,'o'
, and'u'
, and they can appear in both cases.
Example 1:
Input: s = "hello"
Output: "holle"
Example 2:
Input: s = "leetcode"
Output: "leotcede"
Solution:
To solve this problem, we will use the popular technique of two-pointers.
The main idea of solving problems like this -- is to understand base techniques and use them later in medium and hard problems.
We are going to prepare a vowel dictionary. Then we can iterate in both directions. If both pointers stay on the vowels we can swap them. And continue our direction.
public String reverseVowels(String s) {
Set<Character> dict = new HashSet<>();
dict.add('a');
dict.add('A');
dict.add('e');
dict.add('E');
dict.add('i');
dict.add('I');
dict.add('o');
dict.add('O');
dict.add('u');
dict.add('U');
int reader = 0;
int writer = s.length() - 1;
StringBuilder sb = new StringBuilder(s);
while (reader < writer) {
if(!dict.contains(s.charAt(reader))){
reader ++;
}
if (!dict.contains(s.charAt(writer))){
writer--;
}
if (dict.contains(s.charAt(reader)) && dict.contains(s.charAt(writer))){
char tmp = s.charAt(reader);
sb.setCharAt(reader++, s.charAt(writer));
sb.setCharAt(writer--, tmp);
}
}
return sb.toString();
}
Does it clear? Write your comments.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK