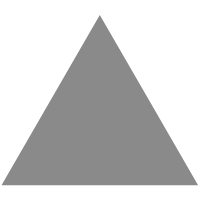
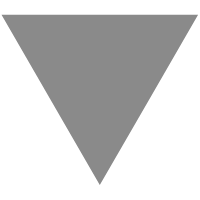
How to Handle Multiple Inheritance and TypeScript Mixins
source link: https://blog.bitsrc.io/multiple-inheritance-or-typescript-mixins-10076c4f136a
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
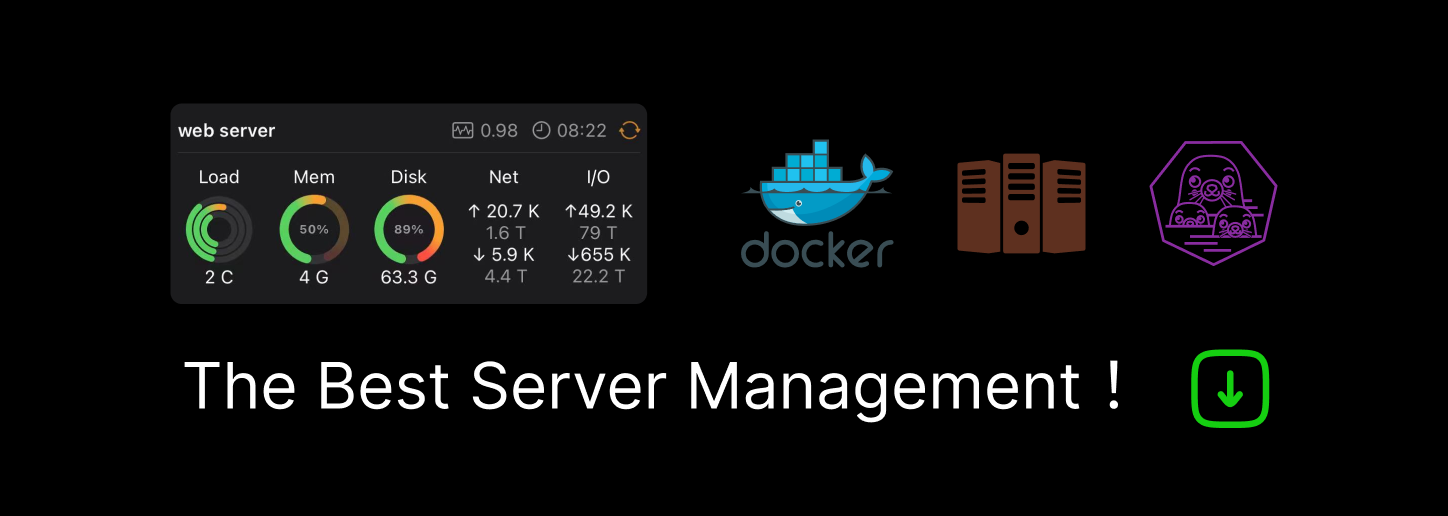
How to Handle Multiple Inheritance and TypeScript Mixins
Photo by Daniel McCullough on Unsplash
Multiple inheritance is a feature where a class can extend more than one base class.
The problem
What if we want to create different UI components like “button”, “input”, “action list item” and “menu item”? All these components have different behavior and look and feel. However, they all can be disabled, can have a base color, and the last two common base class. We are good developers and want to write clean and DRY code. So, let’s see what we can do with this situation.
Extending
Let’s say, we have three classes that provide three methods and we want to construct the fourth class that will expose all these three methods.
The most logical decision is to do something like this:
However, it won’t work. Typescript allows for an extension of only one base class. So, we need to extend them one by one in a chain.
Everything works and we can live with that, but what if in some of the resulting classes we don’t need method b
? We still will have it because class C
extends class B
and without it won’t have a method a
from the class A
. Of course, we can create class combinations like class AC
that will extend A
and not extend B
and so on, but this code very quickly will become unreadable and unmaintainable.
Composition
Instead of extending, we can compose our classes inside the resulting one.
This solution is pretty good and highly recommended if you are constructing components in Angular. But it has its limitations as well. What if some of these classes have properties or getters, we want to address directly in our class D
? Or we don’t want to call methods this long way?
Mixins
Typescript gives us a wonderful pattern allowing us to create the function that will extend a base class. Let’s implement the extension of classes A
and C
by D
. And, let’s our A
class has protected property _propA
and getter for it.
First of all, we need to make some preparations. We need to define the type Constructor
and interfaces that classes A
and C
implement.
Now, we can create a mixin function for class A
.
Let’s understand what’s going on here. The function mixinA
expects an anonymous class as an argument and returns a class expression pattern that extends the class we provided as an argument. Inside this expression pattern, we define all properties and methods we want class A
to have.
Now let’s create mixin for class C
.
And now let’s finally construct our resulting class D
.
You can see that class D
has all methods and properties inherited from classes A
and C
.
Awesome! Now, what if we want our class D
to have its base class, in addition to classes A
and C
? The only thing we will need to do is just define it and supply it to mixinA
as an argument.
Using Constructor
type generic, we can restrict which type we can supply as base
to mixin function.
Conclusion
Despite the JavaScript restriction of extending only one class, we have a way to solve the problem cleanly and nicely. We can use compositions or mixins depending on the tasks set before us.
Go composable: Build apps faster like Lego
Bit is an open-source tool for building apps in a modular and collaborative way. Go composable to ship faster, more consistently, and easily scale.
Build apps, pages, user-experiences and UIs as standalone components. Use them to compose new apps and experiences faster. Bring any framework and tool into your workflow. Share, reuse, and collaborate to build together.
Help your team with:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK