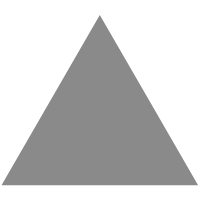
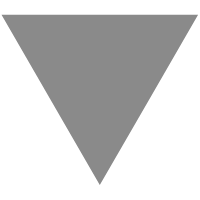
Laravel 8 File Upload and Download Tutorial Example
source link: https://www.laravelcode.com/post/laravel-8-file-upload-and-download-tutorial-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
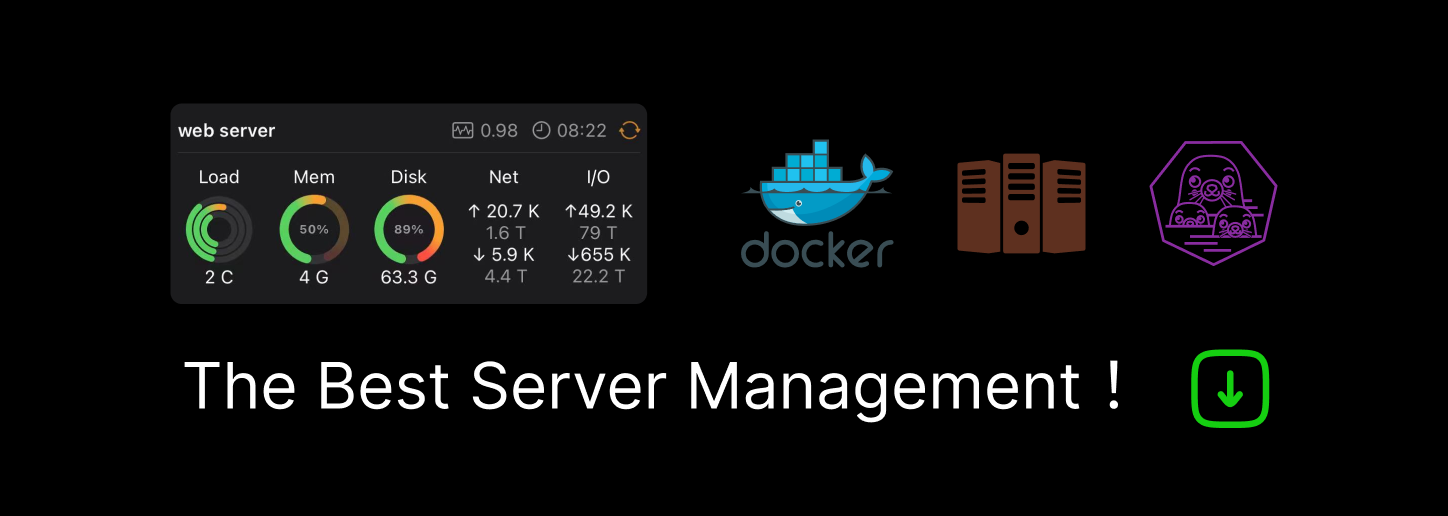
Laravel 8 File Upload and Download Tutorial Example
File upload and download is most common and important functionality. Laravel provides simple ways to upload and download files. In Laravel application, you can store files in storage or public folder.
In this article, we will see examples how you can store uploaded files and download. So first lets start with file upload examples:
Uploading and Storing file in Laravel
There are many ways you can store files in either storage folder or public folder. By default Laravel gives unique ID to filename while storing file. In the below examples file will be stored in Storage folder. You can get the file path in return.
/**
* upload file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function upload(Request $request)
{
$path = Storage::put('images', $request->file('image'));
echo($path); // images/FGZOpU9V5guncEb6ljnDqHAg2z7rd3yL83PgCihf.jpg
}
If you want to store file with the current file name, you can use getClientOriginalName()
method to get the current file name and use putFileAs()
method to store with current file name. The third parameter is to set the name for file.
/**
* upload file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function upload(Request $request)
{
$name = $request->file('image')->getClientOriginalName();
$path = Storage::putFileAs('images', $request->file('image'), $name);
echo($path); // images/avatar.jpg
}
If you want to store file in public folder instead of storage folder, then you can simply chain move()
method with $request
object. move() method acceps two parameters, first one is folder name and second is file name.
/**
* upload file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function upload(Request $request)
{
$name = $request->file('image')->getClientOriginalName();
$path = $request->file('image')->move('images', $name);
echo($path);
}
Downloading file
Downloading files in Laravel is even more simple than uploading. You can pass download()
method with file path to download file.
/**
* download file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function download(Request $request)
{
return Storage::download('images/test.jpg');
}
Same way, if you want to download file from the public folder, you can use download()
method from Response class.
/**
* download file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function download(Request $request)
{
$file = public_path(). "/images/test.jpg";
$headers = ['Content-Type: image/jpeg'];
return \Response::download($file, 'plugin.jpg', $headers);
}
It is better to first check whether the file exist or not to prevent error when the file not found on location.
/**
* download file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function download(Request $request)
{
$file = public_path(). "/images/test.jpg";
$headers = ['Content-Type: image/jpeg'];
if (file_exists($file)) {
return \Response::download($file, 'plugin.jpg', $headers);
} else {
echo('File not found.');
}
}
Deleting Files
Deleting files is same as downloading file. You may pass delete()
method to remove file.
/**
* delete file
*
* @param \Illuminate\Http\Request $request
*
* @return \Illuminate\Auth\Access\Response
*/
public function delete(Request $request)
{
if (file_exists($file)) {
return unlink($file);
} else {
echo('File not found.');
}
}
Thus this way, you can upload, downloads and delete files in your Laravel application. I hope you liked the article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK