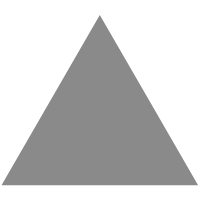
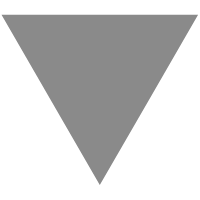
MaxHeightScrollView in Android using Android Studio
source link: https://chintanrathod.com/2015/04/13/maxheightscrollview-in-android-using-android-studio/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
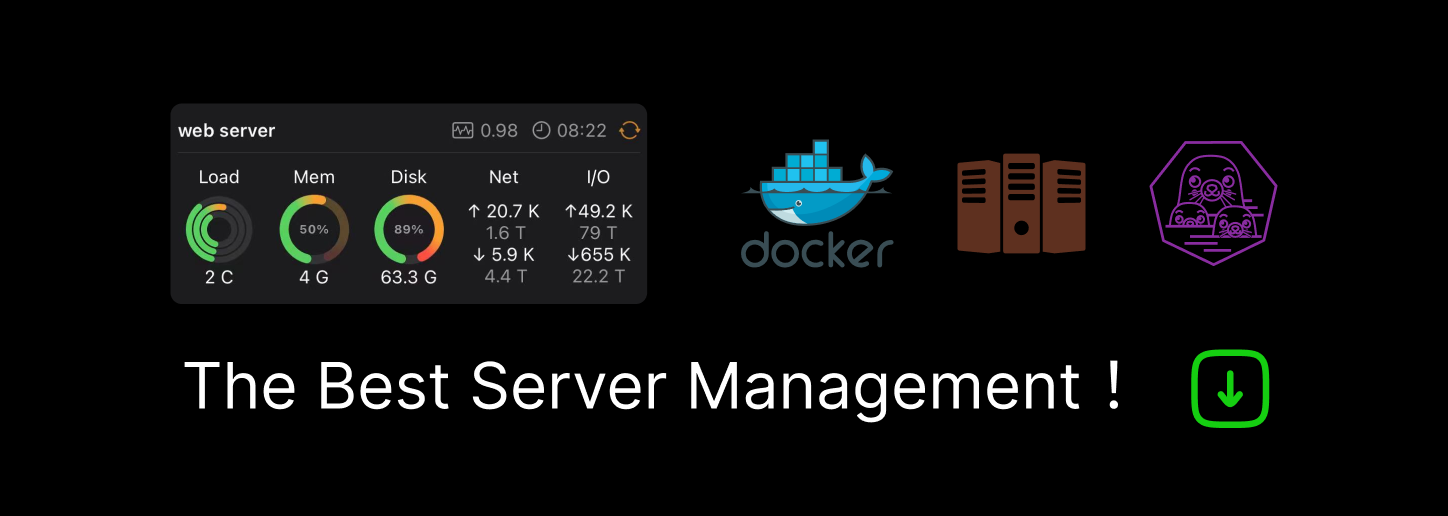
MaxHeightScrollView in Android using Android Studio
In Android, there are two constants for providing height and width of any view. But if you provide any specific height to any view, it won’t be change until you change it dynamically or statically in layout file.
What if you want to provide MAX height to any view? Max height means, your view must not exceed the limit of provided height.
In this article, I am explaining how to provide MAX height to ScrollView
in Android. So let’s begin.
Step 1
Create a project in Android Studio
Create ProjectSelect Target DevicesSelect Activity typeGive name to activity and layout filesStep 2
Create a new file called “MaHeightScrollView
” which extends “ScrollView
” and paste below code inside it.
[java]
import android.annotation.TargetApi;
import android.content.Context;
import android.content.res.TypedArray;
import android.os.Build;
import android.util.AttributeSet;
import android.widget.ScrollView;
public class MaxHeightScrollView extends ScrollView {
private int maxHeight;
private final int defaultHeight = 200;
public MaxHeightScrollView(Context context) {
super(context);
}
public MaxHeightScrollView(Context context, AttributeSet attrs) {
super(context, attrs);
if (!isInEditMode()) {
init(context, attrs);
}
}
public MaxHeightScrollView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
if (!isInEditMode()) {
init(context, attrs);
}
}
@TargetApi(Build.VERSION_CODES.LOLLIPOP)
public MaxHeightScrollView(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
if (!isInEditMode()) {
init(context, attrs);
}
}
private void init(Context context, AttributeSet attrs) {
if (attrs != null) {
TypedArray styledAttrs = context.obtainStyledAttributes(attrs, R.styleable.MaxHeightScrollView);
//200 is a defualt value
maxHeight = styledAttrs.getDimensionPixelSize(R.styleable.MaxHeightScrollView_maxHeight, defaultHeight);
styledAttrs.recycle();
}
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
heightMeasureSpec = MeasureSpec.makeMeasureSpec(maxHeight, MeasureSpec.AT_MOST);
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
}
}
[/java]
In above code, there is one method called onMeasure
is overriden here.
[java]
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
heightMeasureSpec = MeasureSpec.makeMeasureSpec(maxHeight, MeasureSpec.AT_MOST);
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
}
[/java]
Measure the view and its content to determine the measured width and the measured height. This method is invoked by measure(int, int) and should be overriden by subclasses to provide accurate and efficient measurement of their contents.
Read more : onMeasure(int,int);
[java]
heightMeasureSpec = MeasureSpec.makeMeasureSpec(maxHeight, MeasureSpec.AT_MOST);
[/java]
This line will calculate MAX height of view and it will be provided to super.onMeasure(..)
method.
Step 3
Open layout file and paste below code inside it.
[xml]
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"
android:id="@+id/rootLayout"
android:background="@android:color/white">
<TextView
android:text="@string/hello_world"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/txtHeader"/>
<com.chintanrathod.mhsv.MaxHeightScrollView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/txtHeader"
app:maxHeight="300dp"
android:background="@android:color/holo_blue_bright"
android:layout_marginTop="20dp"
android:fadeScrollbars="false">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:id="@+id/linearHolder">
<!– We will add/remove views dynamically here –>
</LinearLayout>
</com.chintanrathod.mhsv.MaxHeightScrollView>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@android:color/black"
android:layout_above="@+id/txtLimit"
android:layout_marginBottom="10dp"/>
<TextView
android:text="@string/limit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/llBottom"
android:id="@+id/txtLimit"
android:layout_marginBottom="30dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:id="@+id/llBottom">
<Button
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="Add"
android:id="@+id/btnAdd"/>
<Button
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="Remove"
android:id="@+id/btnRemove"/>
</LinearLayout>
</RelativeLayout>
[/xml]
Step 4
Open “MainActivity.java
” file and paste below code inside it.
[java]
import android.os.Bundle;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.LinearLayout;
public class MainActivity extends ActionBarActivity {
private Button btnAdd, btnRemove;
private LinearLayout linearHolder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnAdd = (Button) findViewById(R.id.btnAdd);
btnRemove = (Button) findViewById(R.id.btnRemove);
linearHolder = (LinearLayout) findViewById(R.id.linearHolder);
btnAdd.setOnClickListener(new View.OnClickListener() {@Override
public void onClick(View view) {
Button btnNew = new Button(MainActivity.this);
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
btnNew.setLayoutParams(params);
btnNew.setText(String.valueOf(linearHolder.getChildCount() + 1));
btnNew.setTextSize(20.0f);
linearHolder.addView(btnNew);
}
});
btnRemove.setOnClickListener(new View.OnClickListener() {@Override
public void onClick(View view) {
/**
* Check this condition before removing views.
*/
if (linearHolder.getChildCount() & gt; 0) {
linearHolder.removeViewAt(linearHolder.getChildCount() – 1);
}
}
});
}
}
[/java]
Step 5
Build your project and Run your sample code in device or AVD (Android Virtual Device).
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK