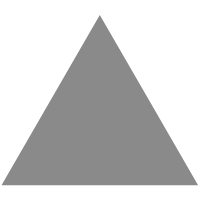
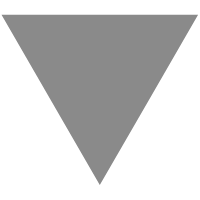
Ferret Programmer's Manual
source link: https://ferret-lang.org/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
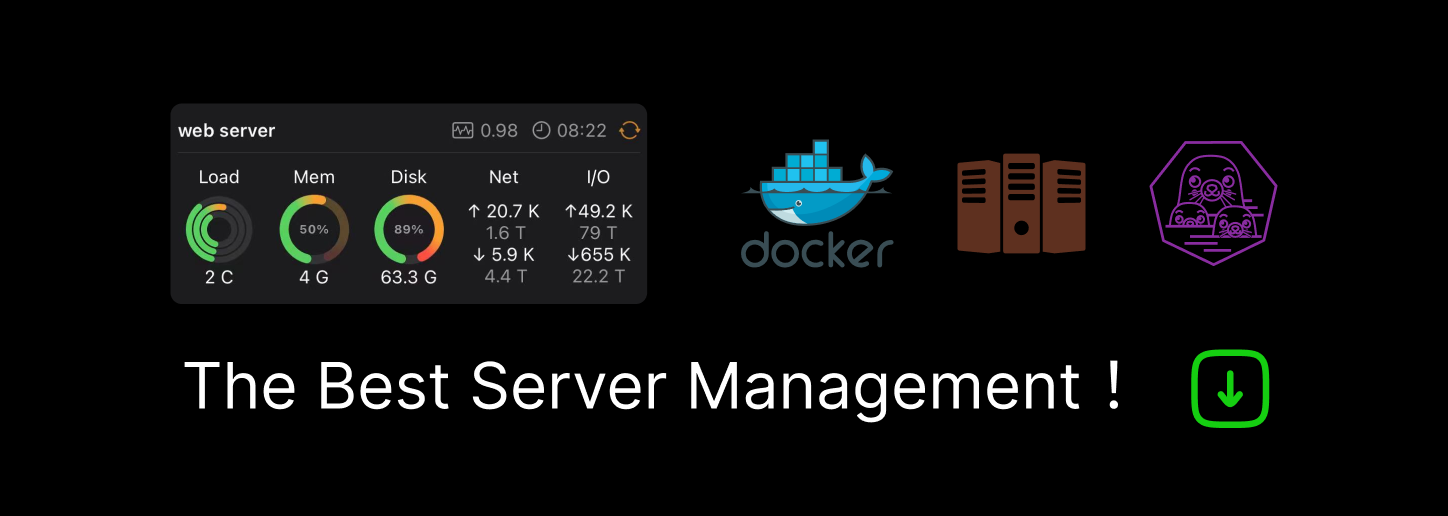
What Is Ferret
Ferret is a free software lisp implementation designed to be used in real time embedded control systems. Ferret lisp compiles down to self contained C++11. Generated code is portable between any Operating System and/or Microcontroller that supports a C++11 compliant compiler. It has been verified to run on architectures ranging from embedded systems with as little as 2KB of RAM to general purpose computers running Linux/Mac OS X/Windows.
- General Purpose Computers
- Clang on Mac OS X
- GCC & Clang on Linux
- Microcontrollers
- Arduino
- Uno / Atmega328
- Due / AT91SAM3X8E
- 101 / Intel Curie
- Teensy
- 2.0 / 16 MHz AVR
- 3.2 / Cortex-M4
- 3.6 / Cortex-M4F
- SparkFun SAMD21 Mini / ATSAMD21G18 - ARM Cortex-M0+
- NodeMcu - ESP8266
- Arduino
- Hardware / Operating System Support
Features
- Tailored for Real Time Control Applications. (Deterministic Execution.)
- Immutable Data Structures
- Functional
- Macros
- Easy FFI (Inline C,C++. See Accessing C,C++ Libraries)
- Easily Embeddable (i.e Ferret fns are just C++ functors.)
- Memory Pooling (Ability to run without heap memory. See Memory Management)
- Destructuring
- Module System
Download
Ferret is available as prebuilt and source code distributions. See Building From Sources for links to source distribution.
Platform independent builds (requires JVM),
Supported package managers,
- Debian/Ubuntu
echo "deb [trusted=yes]\ https://ferret-lang.org/debian-repo ferret-lisp main" >> /etc/apt/sources.list apt-get update apt-get install ferret-lisp
- Clojars - https://clojars.org/ferret
[ferret "0.4.0-171a575"]
A glimpse of Ferret
On any system, we can just compile a program directly into an executable. Here's a program that sums the first 5 positive numbers.
;;; lazy-sum.clj (defn positive-numbers ([] (positive-numbers 1)) ([n] (cons n (lazy-seq (positive-numbers (inc n)))))) (println (->> (positive-numbers) (take 5) (apply +)))
We can compile this program using ferret, creating an executable named lazy-sum.
$ ./ferret -i lazy-sum.clj $ g++ -std=c++11 -pthread lazy-sum.cpp -o lazy-sum $ ./lazy-sum 15
Output will be placed in a a file called lazy-sum.cpp. When -c flag is used ferret will call g++ or if set CXX environment variable on the resulting cpp file.
$ ./ferret -i lazy-sum.clj -c $ ./lazy-sum 15
Following shows a blink example for Arduino. (See section Arduino Boards for more info on how to use Ferret lisp on Arduino boards.)
;;; blink.clj (require '[ferret.arduino :as gpio]) (gpio/pin-mode 13 :output) (forever (gpio/digital-write 13 1) (sleep 500) (gpio/digital-write 13 0) (sleep 500))
$ ./ferret -i blink.clj -o blink/blink.ino
Then upload as usual. Following is another example, showing the usage of Memory Pooling. Program will blink two LEDs simultaneously at different frequencies (Yellow LED at 5 hz Blue LED at 20 hz). It uses a memory pool of 512 bytes allocated at compile time instead of calling malloc/free at runtime.
(configure-runtime! FERRET_MEMORY_POOL_SIZE 512 FERRET_MEMORY_POOL_PAGE_TYPE byte) (require '[ferret.arduino :as gpio]) (def yellow-led 13) (def blue-led 12) (gpio/pin-mode yellow-led :output) (gpio/pin-mode blue-led :output) (defn make-led-toggler [pin] (fn [] (->> (gpio/digital-read pin) (bit-xor 1) (gpio/digital-write pin)))) (def job-one (fn-throttler (make-led-toggler yellow-led) 5 :second :non-blocking)) (def job-two (fn-throttler (make-led-toggler blue-led) 20 :second :non-blocking)) (forever (job-one) (job-two))
$ ./ferret -i ferret-multi-led.clj -o ferret-multi-led/ferret-multi-led.ino
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK