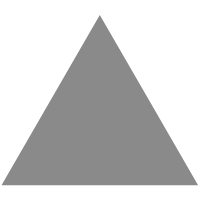
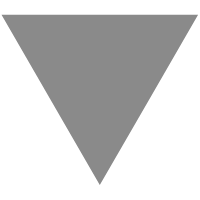
如何去掉vue的url地址中的#号?及其原理?
source link: https://www.fly63.com/article/detial/12041
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
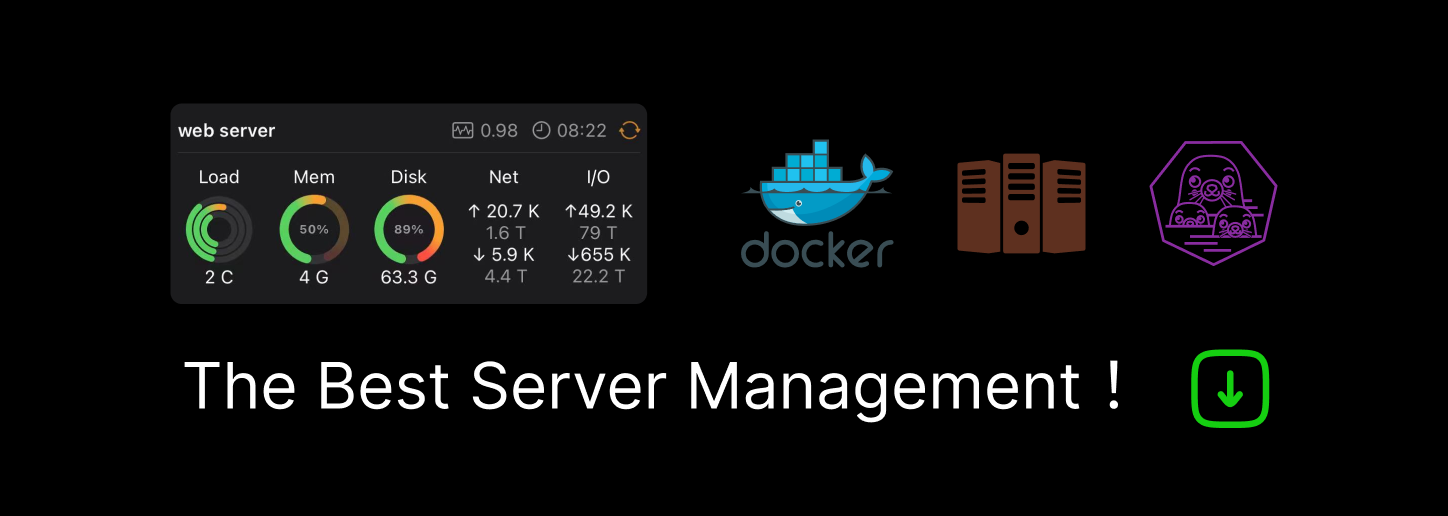
如何去掉vue的url地址中的#号?
import Vue from 'vue';
import VueRouter from 'vue-router';
Vue.use(VueRouter)
// 1. 定义一些路由
// 每个路由都需要映射到一个组件。
const routes = [
{ path: '/home', component: ()=> import('../views//home.vue') },
{ path: '/about', component: ()=> import('../views/about.vue') },
]
const router = new VueRouter({
mode: 'hash', //默认是hash模式,url是带#号的
// mode: 'history', //history模式url不带#号
routes
})
export default router
hash模式实现原理
hash模式主要是根据url的hash值来跳转不同的路由页面。
采用hash模式的路由模式中,url后面有一个#,#后面包括#就是此路由的hash值,hash模式背后的原理是onhashchange事件,可以在window对象上监听这个事件
vue中hash模式的原理就是通过监听hash值的变化来匹配不同的组件,进而来重新渲染视图。
优点
- 兼容性强,兼容性达到了IE8
- 除发送ajax和资源请求外不会发送其他多余请求
- 改变#后的路径、不会自动刷新页面
- 无需服务端进行配合
缺点
- 访问路径上包含#,不美观
- 对于需要锚点功能的需求会与当前路由机制发生冲突
- 重定向操作时,后段无法获取url完整路径。
监听onhashchange事件案例:
src\views\home.vue
<template>
<div>
home
<button @click="handerHref">跳转</button>
</div>
</template>
<script>
export default {
name: 'home',
data(){
return {
}
},
created(){
},
mounted() {
window.addEventListener('hashchange',this.onhashchange)
},
computed:{
},
methods:{
handerHref(){
window.location.href = "http://localhost:8080/#/about"
},
onhashchange(e){
console.log(e.oldURL,'home');
console.log(e.newURL);
console.log(location.hash);
}
}
}
</script>
<style scoped>
</style>
src\views\about.vue
<template>
<div>
about
</div>
</template>
<script>
export default {
name: 'about',
data(){
return {
}
},
created(){
},
mounted() {
window.addEventListener('hashchange',this.onhashchange)
},
computed:{
},
methods:{
onhashchange(e){
console.log(e.oldURL,'about');
console.log(e.newURL);
console.log(location.hash);
}
}
}
</script>
<style scoped>
</style>
history模式实现原理
优点
- 符合url地址规范, 不需要#, 使用起来比较美观
- 可以使用history.state获取完整的路由信息
- 后端可以获取到完整的路由信息
缺点
- 兼容性只到IE10
- 改变url路径后、会重新请求资源。
- 若访问的路由地址不存在时、会报404,需服务端配合支持重定向返回统一的404页面。
history路由中我们使用onpopstate事件函数来监听history路由的变化,但是popstate事件函数只能监听到history.go、forward、back的切换路由方式,但是它不能够监听到pushState添加历史记录(就是在页面中点击某个a标签进行跳转的方式,点击页面顺序:a->b->c,记录的历史记录中a、b、c都存在,而replaceState则不同)、replaceState(点击页面顺序:a->b->c,记录的历史记录中只有a->c,即用c代替了b记录,b记录被删除了)切换路由的方式。
监听popstate、pushState、replaceState事件案例:
src\views\home.vue
<template>
<div>
home
<button @click="handerHref">跳转</button>
</div>
</template>
<script>
export default {
name: 'home',
data(){
return {
}
},
created(){
},
mounted() {
window.addEventListener('hashchange',this.onhashchange)
},
computed:{
},
methods:{
handerHref(){
window.location.href = "http://localhost:8080/#/about"
},
onhashchange(e){
console.log(e.oldURL,'home');
console.log(e.newURL);
console.log(location.hash);
}
}
}
</script>
<style scoped>
</style>
src\views\about.vue
<template>
<div>
about
<button @click="handerBack">返回</button>
</div>
</template>
<script>
export default {
name: 'about',
data(){
return {
}
},
created(){
},
mounted() {
window.addEventListener('hashchange',this.onhashchange) //hash模式跳转页面触发onhashchange事件
window.addEventListener("popstate", this.onpopstate) //popstate事件函数只能监听到history.go、forward、back的切换路由方式,但是它不能够监听到pushState添加历史记录
// 但是它不能够监听到pushState添加历史记录(就是在页面中点击某个a标签进行跳转的方式,点击页面顺序:a->b->c,记录的历史记录中a、b、c都存在,而replaceState则不同)、replaceState(点击页面顺序:a->b->c,记录的历史记录中只有a->c,即用c代替了b记录,b记录被删除了)切换路由的方式
// 对于pushState、replaceState需要通过函数重写的方式进行劫持,也就是说我们重写pushState和replaceState
// 但是我们一般都是pushState来跳转链接,是通过this.$router.replace()来触发;而pushState()是通过this.$router.push()来触发
// 重写pushState方法
const rawPushState = window.history.pushState
window.history.pushState = function (...args) {
rawPushState.apply(window.history, args)
console.log("终于监视到pushState了");
}
// 重写replaceState方法
const rawReplaceState = window.history.replaceState
window.history.replaceState = function (...args) {
rawReplaceState.apply(window.history, args)
console.log("终于监视到replaceState了");
}
},
computed:{
},
methods:{
handerBack(){
// window.location.reload() //刷新
// window.history.go(1) //前进
// window.history.go(-1) //后退
// window.history.forward() //前进
// window.history.back() //后退+刷新
this.$router.replace('/home')
},
onhashchange(e){
console.log(e.oldURL,'about');
console.log(e.newURL);
console.log(location.hash);
},
onpopstate(e){
console.log(e,'popstate')
}
}
}
</script>
<style scoped>
</style>
hash和history的区别
hash模式的url后跟hash值#…,它的原理就是使用window.onHashChange来监听hash值的改变,一旦发生变化就找出此hash值所匹配的组件,进而将组件渲染到页面中。但是hash模式这种带hash值的url是非常丑的,项目中也很少用hash模式。
history模式中的url是以/user这种格式,比较常见,它的原理是通过window.onpopstate来监听路由变化,进而匹配不同的组件来渲染出来。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK