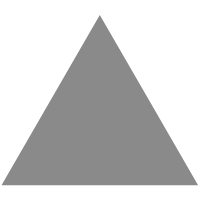
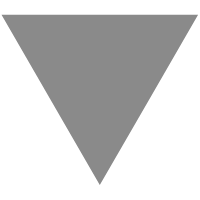
Spring+Dubbo搭建一个简单的分布式
source link: https://blog.51cto.com/u_15430445/5624387
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
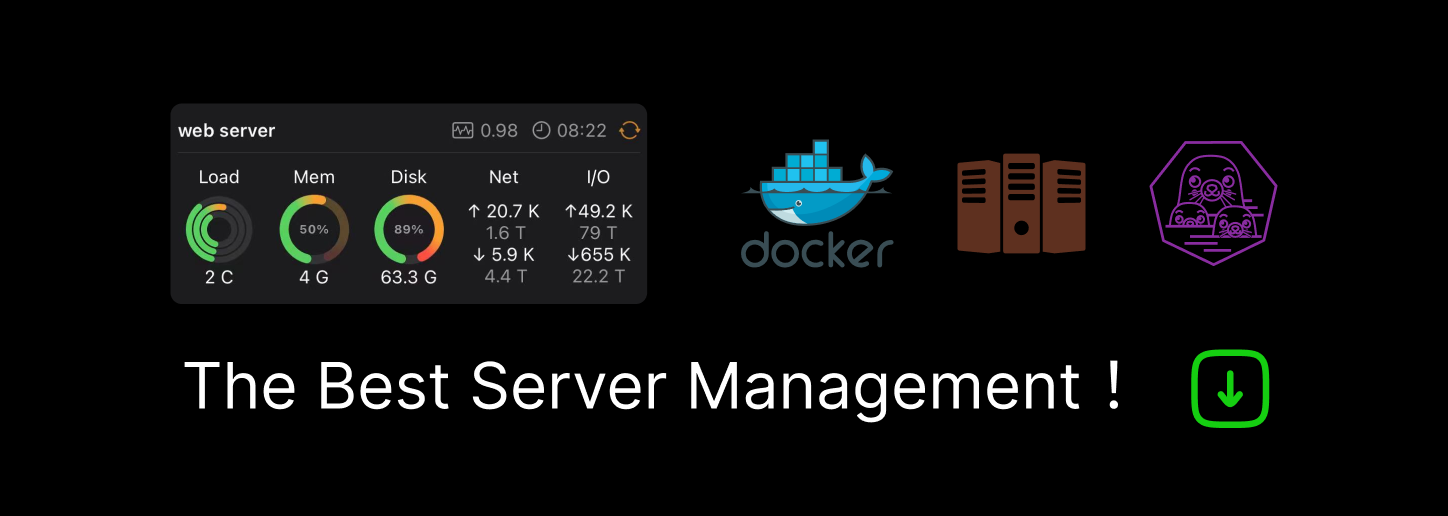
一、zookeeper 环境安装搭建
搭建 zookeeper 之前要确保当前的机器已经有 JDK 环境。
我使用的是 CentOS 7.5 华为云服务器,注意:如果你也同样华为云服务器必须配置一个安全组,不然你的应用程序会无法访问你的 zookeeper 服务器,这一点我在后面也提到了。
二、实现服务接口 dubbo-interface
主要分为下面几步:
- 创建 Maven 项目;
- 创建接口类
- 将项目打成 jar 包供其他项目使用
项目结构:

dubbo-interface 后面被打成 jar 包,它的作用只是提供接口。
1. dubbo-interface 项目创建
File->New->Module...
,然后选择 Maven类型的项目,其他的按照提示一步一步走就好。


2. 创建接口类
public String sayHello(String name);
}
3. 将项目打成 jar 包供其他项目使用
点击右边的 Maven Projects
然后选择 install ,这样 jar 包就打好了。

三、实现服务提供者 dubbo-provider
主要分为下面几步:
- 创建 springboot 项目;
- 加入 dubbo 、zookeeper以及接口的相关依赖 jar 包;
- 在 application.properties 配置文件中配置 dubbo 相关信息;
- 实现接口类;
- 服务提供者启动类编写
项目结构:

1. dubbo-provider 项目创建
创建一个 SpringBoot 项目,注意勾选上 web 模块。

2. pom 文件引入相关依赖
需要引入 dubbo 、zookeeper以及接口的相关依赖 jar 包。注意将本项目和 dubbo-interface
项目的 dependency
依赖的 groupId
和 artifactId
改成自己的。
dubbo 整合spring boot 的 jar 包在这里找dubbo-spring-boot-starter
。zookeeper 的 jar包在 Maven 仓库 搜索 zkclient
即可找到。
<groupId>top.snailclimb</groupId>
<artifactId>dubbo-interface</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<!--引入dubbo的依赖-->
<dependency>
<groupId>com.alibaba.spring.boot</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>2.0.0</version>
</dependency>
<!-- 引入zookeeper的依赖 -->
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.10</version>
</dependency>
3. 在 application.properties 配置文件中配置 dubbo 相关信息
配置很简单,这主要得益于 springboot 整合 dubbo 专属的@EnableDubboConfiguration
注解提供的 Dubbo 自动配置。
server.port=8333
spring.dubbo.application.name=dubbo-provider
spring.dubbo.application.registry=zookeeper://ip地址:2181
4. 实现接口
注意:@Service
注解使用的时 Dubbo 提供的而不是 Spring 提供的。另外,加了Dubbo 提供的 @Service
注解之后还需要加入
import org.springframework.stereotype.Component;
import top.snailclimb.service.HelloService;
@Component
@Service
public class HelloServiceImpl implements HelloService {
@Override
public String sayHello(String name){
return "Hello " + name;
}
}
5. 服务提供者启动类编写
注意:不要忘记加上 @EnableDubboConfiguration
注解开启Dubbo 的自动配置。
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
// 开启dubbo的自动配置
@EnableDubboConfiguration
public class DubboProviderApplication {
public static void main(String[] args){
SpringApplication.run(DubboProviderApplication.class, args);
}
}
四、实现服务消费者 dubbo-consumer
主要分为下面几步:
- 创建 springboot 项目;
- 加入 dubbo 、zookeeper以及接口的相关依赖 jar 包;
- 在 application.properties 配置文件中配置 dubbo 相关信息;
- 编写测试类;
- 服务消费者启动类编写
项目结构:

第1,2,3 步和服务提供者的一样,这里直接从第 4 步开始。
4. 编写一个简单 Controller 调用远程服务
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import top.snailclimb.service.HelloService;
@RestController
public class HelloController {
@Reference
private HelloService helloService;
@RequestMapping("/hello")
public String hello(){
String hello = helloService.sayHello("world");
System.out.println(helloService.sayHello("CrazyK"));
return hello;
}
}
5. 服务消费者启动类编写
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableDubboConfiguration
public class DubboConsumerApplication {
public static void main(String[] args){
SpringApplication.run(DubboConsumerApplication.class, args);
}
}
浏览器访问 http://localhost:8330/hello
页面返回 Hello world
,控制台输出 Hello SnailClimb
,和预期一致,使用SpringBoot+Dubbo 搭建第一个简单的分布式服务实验成功!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK