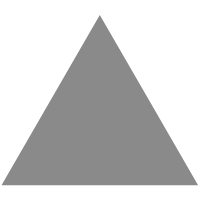
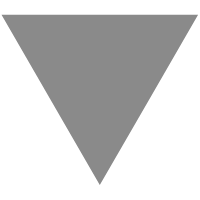
React TS: Don't repeat the type when you pass functions as props, use their type...
source link: https://dev.to/lico/react-ts-dont-repeat-the-type-when-you-pass-functions-as-props-use-their-types-5h6h
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
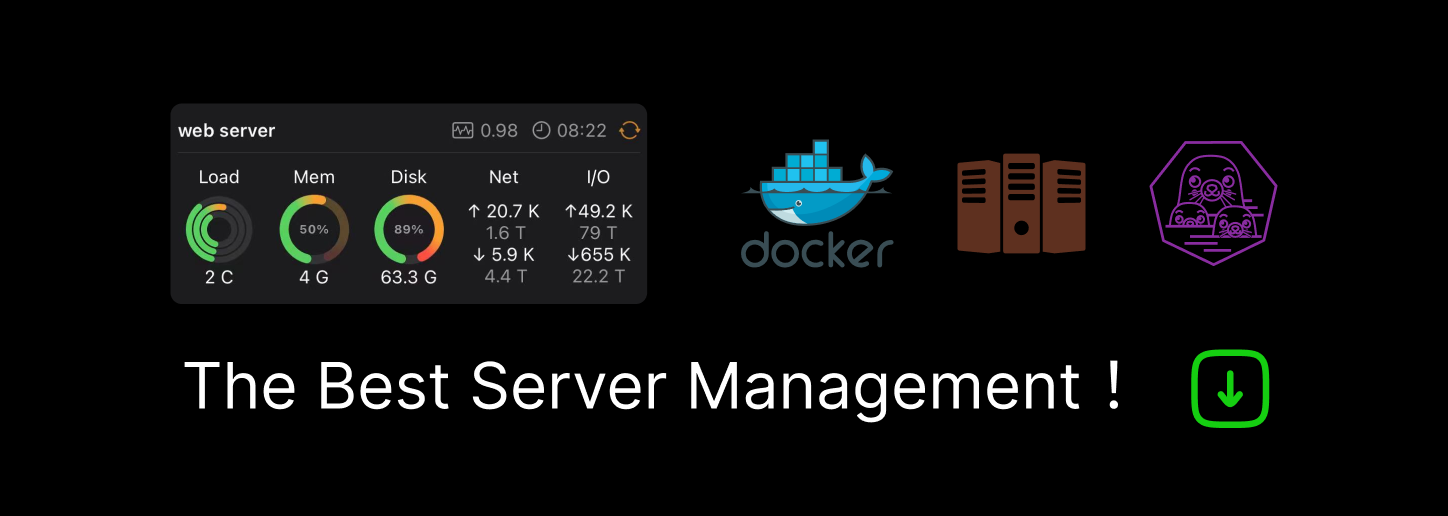

React TS: Don't repeat the type when you pass functions as props, use their types.
Don't repeat the type when you pass functions as props, use their types.
Let's suppose, there is a component named 'SignUpForm'.
export interface SignUpFormProps {
onSubmit?: (values: {
username: string;
nickname: string;
password: string;
}) => void;
}
export const SignUpForm = ({ onSubmit }: SignUpFormProps) => {
const [values, setValues] = useState({
username: "",
nickname: "",
password: "",
});
const handleChange: React.ChangeEventHandler<HTMLInputElement> = (e) => {
setValues((prevValues) => ({
...prevValues,
[e.target.name]: e.target.value,
}));
};
const handleSubmit: React.FormEventHandler = (e) => {
e.preventDefault();
onSubmit?.(values);
};
return (
<form
onSubmit={handleSubmit}
style={{ display: "flex", flexDirection: "column" }}
>
<input
type="text"
name="username"
value={values.username}
onChange={handleChange}
/>
<input
type="text"
name="nickname"
value={values.nickname}
onChange={handleChange}
/>
<input
type="password"
name="password"
value={values.password}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
);
};
The submit event passes the arguments 'username', 'nickname' and 'password' to the onSubmit
prop if it is there.
You can pass the function like below.
function App() {
const handleSubmit = (
values
) => {
console.log(values);
};
return (
<div>
<SignUpForm onSubmit={handleSubmit} />
</div>
);
}
If you don't have an option "noImplicitAny": false
, it occurs an error.
For avoiding this problem, you can repeat the type of the onSubmit
.
If you just repeated like this, you'll have to keep up-to-date depending on the onSubmit
prop.
It would bother you.
In this case, you can get the field type of the interface using brackets.
function App() {
const handleSubmit:SignUpFormProps['onSubmit'] = (
values
) => {
console.log(values);
};
return (
<div>
<SignUpForm onSubmit={handleSubmit} />
</div>
);
}
If there is no interface, use React.ComponentProps
.
function App() {
const handleSubmit: React.ComponentProps<typeof SignUpForm>["onSubmit"] = (
values
) => {
console.log(values);
};
return (
<div>
<SignUpForm onSubmit={handleSubmit} />
</div>
);
}
That's it. I hope it will be helpful for someone.
Happy Coding!
+
Thanks for reading it, all you guys. I'm not sure if that was an appropriate example though. The point was that you can get the field type, and you can use it with other packages. Thank you for your attention again, Good coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK