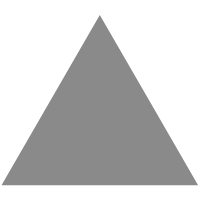
7
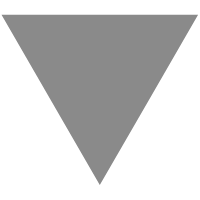
GitHub - Aaron-Junker/sssm
source link: https://github.com/Aaron-Junker/sssm/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
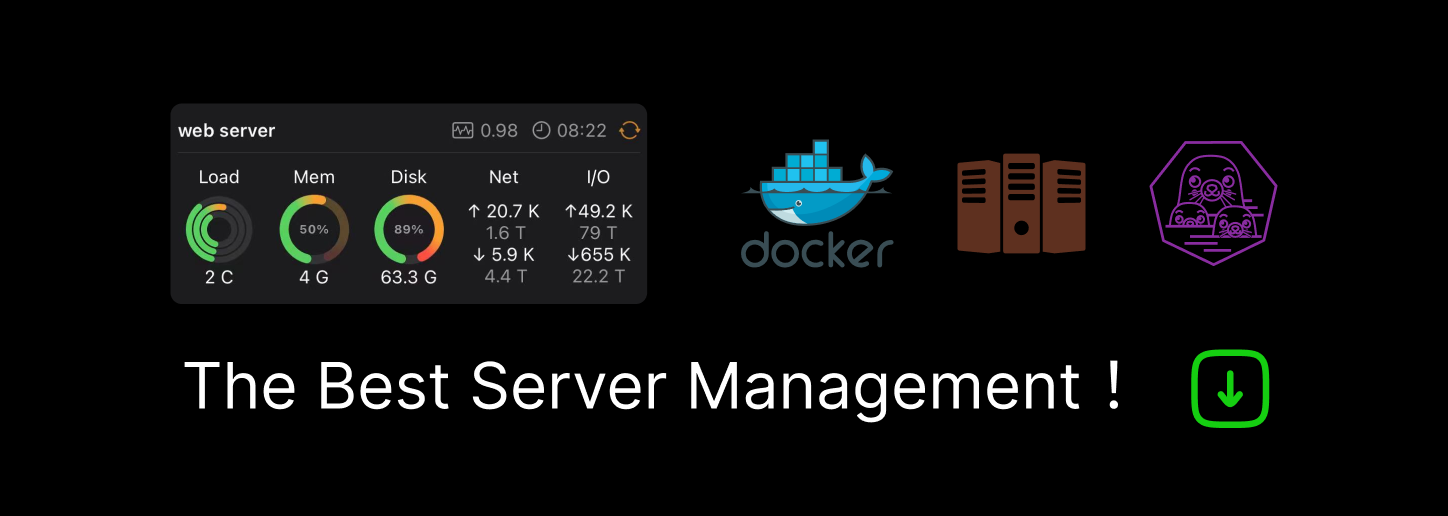
SSSM - Super simple state machine for PHP
Report issues
Please open a new issue to report a bug or request a new feature.
Installation
composer require sssm/sssm
View package on packagist.
Usage
1. Include SSSM
// 1. Include SSSM
include_once "vendor\sssm\sssm\index.php";
use sssm\state;
use sssm\stateMachine;
2. Create the states
Syntax:
$stateName = new state(["stateName of allowed transition 1", "stateName of allowed transition 2", ...], canLoop);
Example:
// State 1 allows transition to state 2 (can loop(default))
$state1 = new state(["state2"]);
// State 2 allows transition to state 3 and state 1 (loop not allowed=
$state2 = new state(["state3","state1"], false);
// State 3 allows no transition (can loop)
$state3 = new state([]);
3. Create the state machine
Syntax:
$stateMachine = new stateMachine($initialState);
Example:
$stateMachine = new stateMachine($state1);
4. Add state events (optional)
Syntax:
$stateName->onStateEnter[] = function(){
// Things that get executed when entering the state
};
$stateName->onLoop[]= function(){
// Things that get executed when the state gets looped
};
$stateName->onStateLeave[]= function(){
// Things that get executed when leaving the state
};
Example:
$state1->onStateEnter[] = function(){
echo "State 1 entered\n";
};
$state1->onLoop[]= function(){
echo "State 1 looped\n";
};
$state1->onStateLeave[]= function(){
echo "State 1 leaved\n";
};
$state2->onStateEnter[] = function(){
echo "State 2 entered\n";
};
5. Loop state
Syntax:
$stateName->loop();
Example:
$state1->loop();
6. Switch state
Syntax:
$stateMachine->switchState($newStateName);
Example:
$stateMachine->switchState($state2);
$stateMachine->switchState($state3);
7. Get current state
Syntax:
// Returns the current state class
$currentState = $stateMachine->getCurrentState();
Example:
echo $stateMachine->getCurrentState() === $state3?"State 3 is current state":"State 3 is not current state";
Full example
<?php
include_once "vendor\sssm\sssm\index.php";
use sssm\state;
use sssm\stateMachine;
$state1 = new state(["state2"]);
$state2 = new state(["state3"]);
$state3 = new state([]);
$stateMachine = new stateMachine($state1);
$state1->onStateEnter[] = function(){
echo "State 1 entered\n";
};
$state1->onLoop[]= function(){
echo "State 1 looped\n";
};
$state1->onStateLeave[]= function(){
echo "State 1 leaved\n";
};
$state2->onStateEnter[] = function(){
echo "State 2 entered\n";
};
$state1->loop();
$stateMachine->switchState($state2);
$stateMachine->switchState($state3);
echo $stateMachine->getCurrentState() === $state3?"State 3 is current state":"State 3 is not current state";
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK